emo { private: //Private Data member section int X, Y; public: //Public Member function section //Default or no argument constructor. Demo() { X = 0;
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
// C++ program to demonstrate example of
// constructor using this pointer.
#include <iostream>
using namespace std;
class Demo {
private: //Private Data member section
int X, Y;
public: //Public Member function section
//Default or no argument constructor.
Demo()
{
X = 0;
Y = 0;
cout << endl
<< "Constructor Called";
}
//Perameterized constructor.
Demo(int X, int Y)
{
this->X = X;
this->Y = Y;
cout << endl
<< "Constructor Called";
}
//Destructor called when object is destroyed
~Demo()
{
cout << endl
<< "Destructor Called" << endl;
}
//To print output on console
void putValues()
{
cout << endl
<< "Value of X : " << X;
cout << endl
<< "Value of Y : " << Y << endl;
}
};
//main function : entry point of program
int main()
{
Demo d1 = Demo(10, 20);
cout << endl
<< "D1 Value Are : ";
d1.putValues();
Demo d2 = Demo(30, 40);
cout << endl
<< "D2 Value Are : ";
d2.putValues();
//cout << endl ;
return 0;
}.

Step by step
Solved in 3 steps with 1 images

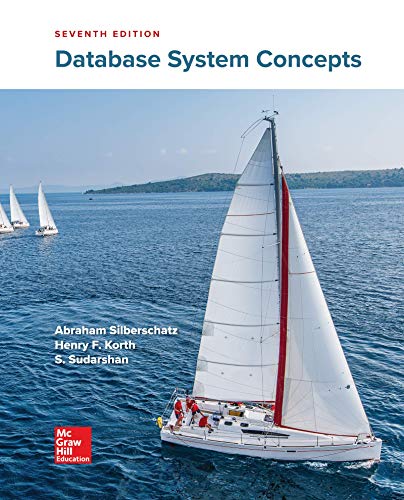
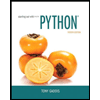
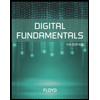
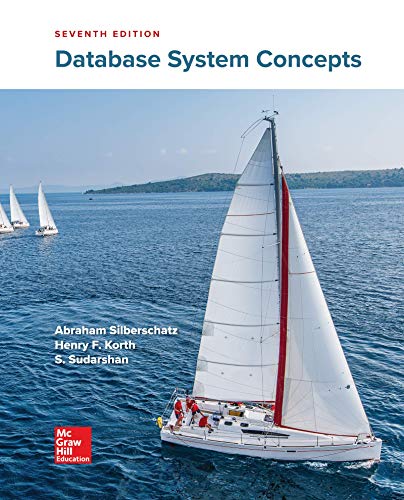
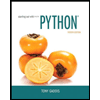
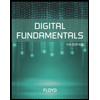
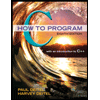
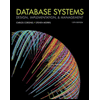
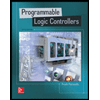