Edit the code so that there is a total price on the checkout.html page of the items in your cart. Add a form below that takes name , email, phone number, and credit card number. Use a regular expression to validate the email, phone number, and credit card. Existing code: products.html Cart shoppingcart.js const product = [ { id: 1, name: "Clorox", price: "$20.00", image: "img/clorox.jpg", category: "Household Cleaner", }, { id: 2, name: "Oxiclean", price: "$8.00", // image: "img/oxiclean.jpg", image: "https://images-na.ssl-images-amazon.com/images/I/811eCrdKg-L._SY450_.jpg", category: "Household Cleaner", }, { id: 3, name: "PineSol", price: "$11.00", // image: "img/pinesol.jpg", image: "https://www.pinesol.com/wp-content/themes/electro/img/products/bottle-original-pine-large.png", category: "Household Cleaner", }, { id: 4, name: "Mr Clean", price: "$12.00", // image: "img/mrclean.jpg", image: "https://upload.wikimedia.org/wikipedia/en/7/73/Mr._Clean_logo.png", category: "Household Cleaner", }, { id: 5, name: "Windex", price: "$15.00", // image: "img/windex.jpg", image: "https://images-na.ssl-images-amazon.com/images/I/71tgjDr5mtL._AC_SX466_.jpg", category: "Household Cleaner", }, ]; product.forEach((element, index) => { let products = ""; products += ""; products += ""; products += "" + element.name + ""; products += "" + element.price + ""; products += "" + element.category + ""; products += "Add to cart"; products += "Remove From Cart"; document.getElementById("productsDiv").innerHTML += products; }); var cart = new Array(); localStorage.removeItem("myItems"); updateCart(); function updateCart(){ let items = localStorage.getItem("myItems") ? JSON.parse(localStorage.getItem("myItems")) : []; var cartCount = document.getElementById("cartCount"); cartCount.innerHTML="("+items.length+") "; } function addtocart(productid) { let object1 = product.filter(item=>item.id==productid)[0]; let alreadyItems = localStorage.getItem("myItems") ? JSON.parse(localStorage.getItem("myItems")) : []; alreadyItems.push(object1); localStorage.setItem("myItems", JSON.stringify(alreadyItems)); updateCart(); console.log(alreadyItems) } function removecart(productid) { const index = cart.indexOf(productid); if (index > -1) { cart.splice(index, 1); } updateCart(); console.log(cart); } checkout.html Document
Edit the code so that there is a total price on the checkout.html page of the items in your cart. Add a form below that takes name , email, phone number, and credit card number. Use a regular expression to validate the email, phone number, and credit card.
Existing code:
products.html
<!DOCTYPE html>
<html>
<head>
<style>
img {
width: 80px;
height: auto;
}
</style>
</head>
<body>
<div style="width: 100%;text-align: right;">
<a href="checkout.html">
<h3><span id="cartCount"></span>Cart</h3>
</a>
</div>
<div id="productsDiv"></div>
<script src="./js/shoppingcart.js"></script>
</body>
</html>
shoppingcart.js
const product = [
{
id: 1,
name: "Clorox",
price: "$20.00",
image: "img/clorox.jpg",
category: "Household Cleaner",
},
{
id: 2,
name: "Oxiclean",
price: "$8.00",
// image: "img/oxiclean.jpg",
image:
"https://images-na.ssl-images-amazon.com/images/I/811eCrdKg-L._SY450_.jpg",
category: "Household Cleaner",
},
{
id: 3,
name: "PineSol",
price: "$11.00",
// image: "img/pinesol.jpg",
image:
"https://www.pinesol.com/wp-content/themes/electro/img/products/bottle-original-pine-large.png",
category: "Household Cleaner",
},
{
id: 4,
name: "Mr Clean",
price: "$12.00",
// image: "img/mrclean.jpg",
image: "https://upload.wikimedia.org/wikipedia/en/7/73/Mr._Clean_logo.png",
category: "Household Cleaner",
},
{
id: 5,
name: "Windex",
price: "$15.00",
// image: "img/windex.jpg",
image:
"https://images-na.ssl-images-amazon.com/images/I/71tgjDr5mtL._AC_SX466_.jpg",
category: "Household Cleaner",
},
];
product.forEach((element, index) => {
let products = "";
products += "<div id='product" + element.id + "' class='card'>";
products += "<img src='" + element.image + "' />";
products += "<h1>" + element.name + "</h1>";
products += "<p class='price'>" + element.price + "</p>";
products += "<p>" + element.category + "</h1>";
products +=
"<p><button id='product" +
element.id +
"Btn" +
element.id +
"' onclick='addtocart(" +
element.id +
")'>Add to cart</button></p>";
products +=
"<p><button id='product" +
element.id +
"Btn" +
(element.id + 1) +
"' onclick='removecart(" +
element.id +
")'>Remove From Cart</button></p>";
document.getElementById("productsDiv").innerHTML += products;
});
var cart = new Array();
localStorage.removeItem("myItems");
updateCart();
function updateCart(){
let items = localStorage.getItem("myItems") ? JSON.parse(localStorage.getItem("myItems")) : [];
var cartCount = document.getElementById("cartCount");
cartCount.innerHTML="("+items.length+") ";
}
function addtocart(productid) {
let object1 = product.filter(item=>item.id==productid)[0];
let alreadyItems = localStorage.getItem("myItems") ? JSON.parse(localStorage.getItem("myItems")) : [];
alreadyItems.push(object1);
localStorage.setItem("myItems", JSON.stringify(alreadyItems));
updateCart();
console.log(alreadyItems)
}
function removecart(productid) {
const index = cart.indexOf(productid);
if (index > -1) {
cart.splice(index, 1);
}
updateCart();
console.log(cart);
}
checkout.html
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
img {
width: 80px;
height: auto;
}
</style>
</head>
<body>
<div id="productsFromCart">
</div>
<script>
let items = localStorage.getItem("myItems") ? JSON.parse(localStorage.getItem("myItems")) : [];
items.forEach((element,index) => {
let products = "";
products += "<div id='product" + element.id + "' class='card'>";
products += "<img src='" + element.image + "' />";
products += "<h1>" + element.name + "</h1>";
products += "<p class='price'>" + element.price + "</p>";
products += "<p>" + element.category + "</h1>";
document.getElementById("productsFromCart").innerHTML += products;
});
</script>
</body>
</html>

Step by step
Solved in 2 steps

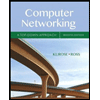
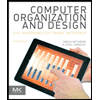
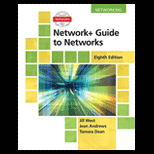
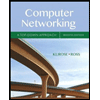
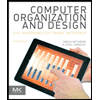
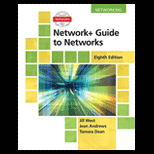
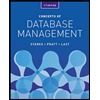
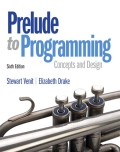
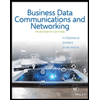