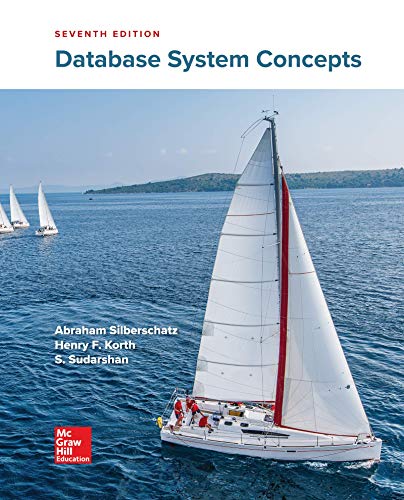
Concept explainers
Double trouble
def double_trouble(items, n):
Suppose, if just for the sake of argument, that the following operation is repeated n times for the given list of items: remove the first element, and append that same element twice to the end of items. Which one of the items would be removed and copied in the last operation performed?
Sure, this problem could be finger-quotes “solved” by actually performing that operation n times, but the point of this exercise is to come up with an analytical solution to compute the result much faster than actually going through that whole rigmarole. To gently nudge you towards thinking in
symbolic and analytical solutions, the automated tester is designed so that anybody trying to brute force their way through this problem by performing all n operations one by one for real will run out
of time and memory long before receiving the answer, as will the entire universe.
To come up with this analytical solution, tabulate some small cases (you can implement the brute force function to compute these) and try to spot the pattern that generalizes to arbitrarily large values of n. You can again also check out Wolfram Alpha or similar systems capable of manipulation
of symbolic formulas to simplify combinatorial and summing formulas.
The reason to pay attention in the discrete math and combinatorics courses is learning to derive symbolic solutions to problems of this nature so that you don't have to brute force their answers in a time that would be prohibitively long to ever be feasible. We met this very same theme back when counting the number of cannonballs that make up a brass monkey pyramid.
![items
Expected result
n
['joe', 'bob', 42]
10
'joe'
[17, 42, 99 ]
1000
17
[17, 42, 99]
10**20
99
10**1000 |'the'
['only', 'the', 'number', 'of',
'items',
'matters']](https://content.bartleby.com/qna-images/question/f8493b25-a9ac-4d4b-aab2-48b43e8654fa/692e9954-3492-4484-a459-dbfda64126a5/s1c8kb_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Write this program in Java using a custom method. Implementation details You will implement this program in a specific way in order to gain some experience with loops, arrays and array lists. Use an array of strings to store the 4 strings listed in the description. Use a do-while loop for your 'game engine'. This means the game starts once the user enters money. The decision to stop occurs at the bottom of the loop. The do-while loop keeps going until the user quits, or there is no money left. The pseudocode for this 'game engine' is shown below: determine the fruits to display (step 3 below) and print them determine if there are 3 or 4 of the same image display the results update the customer balance as necessary prompt to play or quit continue loop if customer wants to play and there's money for another game. Use the Random class to generate a random number between 0 and 3. This random number will be an index into the array of strings. Add the string at that index to an…arrow_forwardplease help with the python problem, thank youarrow_forwardLab Goal : This lab was designed to teach you more about recursion. Lab Description : Take a string and remove all occurrences of the word chicken and count how many chickens were removed. Keep in mind that removing a chicken might show a previously hidden chicken. You may find substring and indexOf useful. achickchickenen - removing the 1st chicken would leave achicken behindachicken - removing the 2nd chicken would leave a behindSample Data : itatfunitatchickenfunchchickchickenenickenchickchickfunchickenbouncetheballchickenSample Output : 01302arrow_forward
- 1. Write a recursive method expFive(n) to compute y=5^n. For instance, if n is 0, y is 1. If n is 3, then y is 125. If n is 4, then y is 625. The recursive method cannot have loops. Then write a testing program to call the recursive method. If you run your program, the results should look like this: > run RecExpTest Enter a number: 3 125 >run RecExpTest Enter a number: 3125 2. For two integers m and n, their GCD(Greatest Common Divisor) can be computed by a recursive function. Write a recursive method gcd(m,n) to find their Greatest Common Divisor. Once m is 0, the function returns n. Once n is 0, the function returns m. If neither is 0, the function can recursively calculate the Greatest Common Divisor with two smaller parameters: One is n, the second one is m mod n. Although there are other approaches to calculate Greatest Common Divisor, please follow the instructions in this question, otherwise you will not get the credit. Meaning your code needs to follow the given algorithm. Then…arrow_forwardCourse: Algorithm Project: We will use the defintion of of n-Queens Problem from the chapter Backtracking. In this project you need to describe Problem and Algorithm and Indicate input and output clearly. Analyze and prove the time complexity of your algorithm. Implement the algorithm using backtracking(including writing testing case).illustrate key functions with comments indicating: What it does, what each parameter is used for, how it handles errors etc. Indicate the testing scenarios and testing the results in a clear way. Make sure source is commented appropriately and structured well.arrow_forwardCorrect and detailed answer will be Upvoted else downvoted. Thank you!arrow_forward
- Recursion Practice Welcome back! In this lab, we will be reviewing recursion by practicing with some basic recursion problems. Objectives Increase familiarity with recursive logic by working through several recursive problems. Taking into consideration a few corner cases through analyzing the test cases. Using a regex expression that will remove punctuation. Getting Started This lab includes the following .java file: L4/└── Recursion.java└── Main.java**Main.java is a read-only file used for testing. It is not included in the starter jar.Here is the starter jar if you would like to code in a different environment: L4.jar. Please complete ALL functions. Make sure to read the description for each function carefully. Do not include any for or while loops in your methods. These can all be completed in a purely recursive style, so do it recursively! In the spirit of incremental development, implement each method one at a time, look at the test cases and take into consideration what is…arrow_forwardJAVAarrow_forwardA basic iterator offers all the following operations except Group of answer choices 1. Except nothing, it can do all of the listed operations. 2. Get the item at the current iteration 3. Return an array of all items 4. Determine if there are more items 5. Go to the next itemarrow_forward
- Lab Goal : This lab was designed to teach you more about recursion. Lab Description : Take a number and recursively determine how many of its digits are even. Return the count of the even digits in each number. % might prove useful to take the number apart digit by digit Sample Data : 453211145322224532714246813579 Sample Output : 23540arrow_forwardPython Big-O Notation/Time Complexity Pls answer only if u know big-o Thanks! Item #3.arrow_forwardI need the code from start to end with no errors and the explanation for the code ObjectivesJava refresher (including file I/O)Use recursionDescriptionFor this project, you get to write a maze solver. A maze is a two dimensional array of chars. Walls are represented as '#'s and ' ' are empty squares. The maze entrance is always in the first row, second column (and will always be an empty square). There will be zero or more exits along the outside perimeter. To be considered an exit, it must be reachable from the entrance. The entrance is not an exit.Here are some example mazes:mazeA7 9# # ###### # # ## # # #### # ## ##### ## ########## RequirementsWrite a MazeSolver class in Java. This program needs to prompt the user for a maze filename and then explore the maze. Display how many exits were found and the positions (not indices) of the valid exits. Your program can display the valid exits found in any order. See the examples below for exact output requirements. Also, record…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
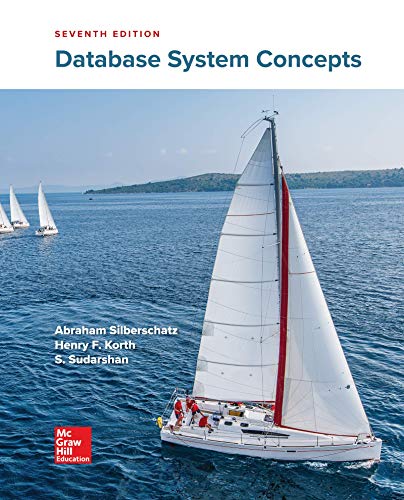
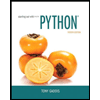
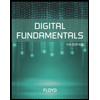
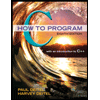
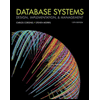
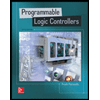