Write this program in Java using a custom method. Implementation details You will implement this program in a specific way in order to gain some experience with loops, arrays and array lists. Use an array of strings to store the 4 strings listed in the description. Use a do-while loop for your 'game engine'. This means the game starts once the user enters money. The decision to stop occurs at the bottom of the loop. The do-while loop keeps going until the user quits, or there is no money left. The pseudocode for this 'game engine' is shown below: determine the fruits to display (step 3 below) and print them determine if there are 3 or 4 of the same image display the results update the customer balance as necessary prompt to play or quit continue loop if customer wants to play and there's money for another game. Use the Random class to generate a random number between 0 and 3. This random number will be an index into the array of strings. Add the string at that index to an ArrayList. You'll have to do this four times. When done, your ArrayList will contain 4 strings that represent randomly selected fruit images. Write a custom method that is called within your do-while loop. This method will accept the array list as a parameter, and it will return an integer that represents the number of times an 'fruit' appeared in the array list. Inside this method, use the frequency method from the Collections class to determine how many times a particular value is in a ArrayList. You'll have to call Collections.frequency for each image to get the counts by image. You can see an example of using this method on page 794 in your text. Note that this is an exception to our rule about using just the techniques in our chapters because I want you to be aware of the Collections class and how it can be helpful in certain situations. If your custom method returns a three, then add $2 to the balance. If it returns a four, then add $10 to the customer's balance. Your code should display the contents of the arraylist on the same line with spaces between them. Your code should display the results of the play -- see sample output -- and the current balance. At the bottom of the do-while loop, prompt the user if they want to continue to play (P) or to quit (Q). Case should not matter. It's OK if the user enters a character other than those two characters. Treat anything other than a 'Q' as a play. Sample Output Enter dollar amount to play ==> 5 Apple Apple Orange Orange << Not a winner this time >> Balance is: $4 Play Again (P) or Quit (Q) p Banana Banana Orange Cherry << Not a winner this time >> Balance is: $3 Play Again (P) or Quit (Q) p Banana Apple Banana Banana << You matched 3! That's $2 >> Balance is: $5 Play Again (P) or Quit (Q) q Thanks for playing! Cash Balance is $5
Write this program in Java using a custom method. Implementation details You will implement this program in a specific way in order to gain some experience with loops, arrays and array lists. Use an array of strings to store the 4 strings listed in the description. Use a do-while loop for your 'game engine'. This means the game starts once the user enters money. The decision to stop occurs at the bottom of the loop. The do-while loop keeps going until the user quits, or there is no money left. The pseudocode for this 'game engine' is shown below: determine the fruits to display (step 3 below) and print them determine if there are 3 or 4 of the same image display the results update the customer balance as necessary prompt to play or quit continue loop if customer wants to play and there's money for another game. Use the Random class to generate a random number between 0 and 3. This random number will be an index into the array of strings. Add the string at that index to an ArrayList. You'll have to do this four times. When done, your ArrayList will contain 4 strings that represent randomly selected fruit images. Write a custom method that is called within your do-while loop. This method will accept the array list as a parameter, and it will return an integer that represents the number of times an 'fruit' appeared in the array list. Inside this method, use the frequency method from the Collections class to determine how many times a particular value is in a ArrayList. You'll have to call Collections.frequency for each image to get the counts by image. You can see an example of using this method on page 794 in your text. Note that this is an exception to our rule about using just the techniques in our chapters because I want you to be aware of the Collections class and how it can be helpful in certain situations. If your custom method returns a three, then add $2 to the balance. If it returns a four, then add $10 to the customer's balance. Your code should display the contents of the arraylist on the same line with spaces between them. Your code should display the results of the play -- see sample output -- and the current balance. At the bottom of the do-while loop, prompt the user if they want to continue to play (P) or to quit (Q). Case should not matter. It's OK if the user enters a character other than those two characters. Treat anything other than a 'Q' as a play. Sample Output Enter dollar amount to play ==> 5 Apple Apple Orange Orange << Not a winner this time >> Balance is: $4 Play Again (P) or Quit (Q) p Banana Banana Orange Cherry << Not a winner this time >> Balance is: $3 Play Again (P) or Quit (Q) p Banana Apple Banana Banana << You matched 3! That's $2 >> Balance is: $5 Play Again (P) or Quit (Q) q Thanks for playing! Cash Balance is $5
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write this program in Java using a custom method.
Implementation details
You will implement this program in a specific way in order to gain some experience with loops, arrays and array lists.
- Use an array of strings to store the 4 strings listed in the description.
- Use a do-while loop for your 'game engine'. This means the game starts once the user enters money. The decision to stop occurs at the bottom of the loop. The do-while loop keeps going until the user quits, or there is no money left. The pseudocode for this 'game engine' is shown below:
- determine the fruits to display (step 3 below) and print them
- determine if there are 3 or 4 of the same image
- display the results
- update the customer balance as necessary
- prompt to play or quit
- continue loop if customer wants to play and there's money for another game.
- Use the Random class to generate a random number between 0 and 3. This random number will be an index into the array of strings. Add the string at that index to an ArrayList. You'll have to do this four times. When done, your ArrayList will contain 4 strings that represent randomly selected fruit images.
- Write a custom method that is called within your do-while loop. This method will accept the array list as a parameter, and it will return an integer that represents the number of times an 'fruit' appeared in the array list.
- Inside this method, use the frequency method from the Collections class to determine how many times a particular value is in a ArrayList. You'll have to call Collections.frequency for each image to get the counts by image. You can see an example of using this method on page 794 in your text. Note that this is an exception to our rule about using just the techniques in our chapters because I want you to be aware of the Collections class and how it can be helpful in certain situations.
- If your custom method returns a three, then add $2 to the balance. If it returns a four, then add $10 to the customer's balance.
- Your code should display the contents of the arraylist on the same line with spaces between them.
- Your code should display the results of the play -- see sample output -- and the current balance.
- At the bottom of the do-while loop, prompt the user if they want to continue to play (P) or to quit (Q). Case should not matter. It's OK if the user enters a character other than those two characters. Treat anything other than a 'Q' as a play.
Sample Output
Enter dollar amount to play ==> 5
Apple Apple Orange Orange
<< Not a winner this time >>
Balance is: $4
Play Again (P) or Quit (Q) p
Banana Banana Orange Cherry
<< Not a winner this time >>
Balance is: $3
Play Again (P) or Quit (Q) p
Banana Apple Banana Banana
<< You matched 3! That's $2 >>
Balance is: $5
Play Again (P) or Quit (Q) q
Thanks for playing! Cash Balance is $5
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
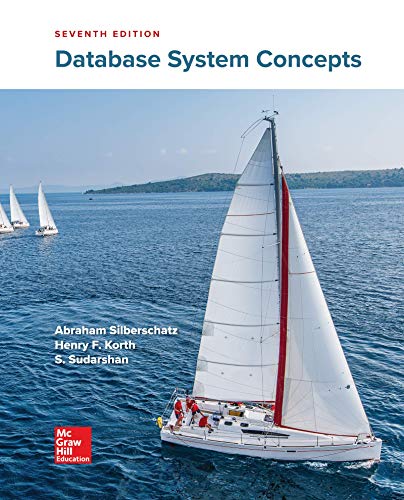
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
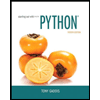
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
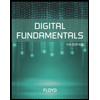
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
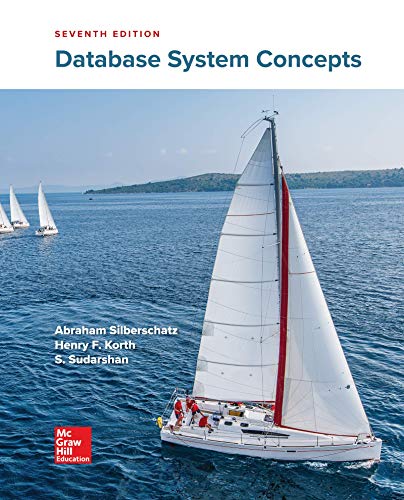
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
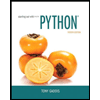
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
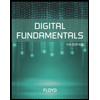
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
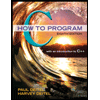
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
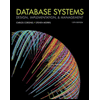
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
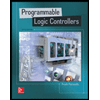
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education