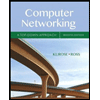
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Direction: Complete the program below using case switch statement and single array with Function. Copy
the source code and screen the sample output.
PROGRAMMING
//Single array - A group of consecutive memory locations of the same name and type, structures of related
data items.
//Array index always starts with zero.
// Single array with function
#include<iostream>
using namespace std;
// Function Name
int sumAll(int num[5]);
void display(int num[5]);
//Variable global declaration
int x, sum=0, choice;
int num[5];
main(){
// Save the input numbers to the memory
for(x=0; x<=4; x++)
{ cout<<(" Enter a number: ");
cin>>num[x];
}
cout<<"\nCHOICES: ";
cout<<"\n 1.The sum of all numbers ";
cout<<"\n 2. The sum of even subscripts";
cout<<"\n 3. The sum of even numbers ";
cout<<"\n 4. The product of odd subscripts ";
cout<<"\n 5. The product of odd numbers ";
cout<<"\n 6. The highest number ";
cout<<"\n 7. The lowest number ";
cout<<"\n 8. Display all numbers";
the source code and screen the sample output.
PROGRAMMING
//Single array - A group of consecutive memory locations of the same name and type, structures of related
data items.
//Array index always starts with zero.
// Single array with function
#include<iostream>
using namespace std;
// Function Name
int sumAll(int num[5]);
void display(int num[5]);
//Variable global declaration
int x, sum=0, choice;
int num[5];
main(){
// Save the input numbers to the memory
for(x=0; x<=4; x++)
{ cout<<(" Enter a number: ");
cin>>num[x];
}
cout<<"\nCHOICES: ";
cout<<"\n 1.The sum of all numbers ";
cout<<"\n 2. The sum of even subscripts";
cout<<"\n 3. The sum of even numbers ";
cout<<"\n 4. The product of odd subscripts ";
cout<<"\n 5. The product of odd numbers ";
cout<<"\n 6. The highest number ";
cout<<"\n 7. The lowest number ";
cout<<"\n 8. Display all numbers";
do{
cout<<("\n\nEnter your choice[1-7]: ");
cin>>choice;
switch(choice)
{ case 1:cout<< "Display the sum of all numbers";
sum= sumAll(num);
cout<<"\nThe sum is "<<sum;
break;
case 2:cout<< "Display the sum of numbers in even subscripts";
sum=0;
for(x=0; x<=4; x=x+2)
{ sum = sum +num[x]; }
cout<<"\nThe sum is "<<sum;
break;
case 3:cout<< "Display the sum of even numbers";
sum=0;
for(x=0; x<=4; x++)
{if (num[x] % 2==0)
{
sum = sum +num[x];
} // if
} //for loop
cout<<"\nThe sum is "<<sum;
break;
case 8: cout<< "\nDisplay all numbers\n";
display(num);
break;
default : cout<<"\nYou entered an Invalid Number\n\n";
} //switch
} while (choice<=8);
} // end of main
//START OF FUNCTION
int sumAll(int num[5]) //case 1 Sum all numbers
{ sum=0;
for(x=0; x<=4; x++)
{ sum = sum +num[x]; }
return sum; }
void display(int num[5]) // case 8 display all numbers
{
cout<<("\n\nEnter your choice[1-7]: ");
cin>>choice;
switch(choice)
{ case 1:cout<< "Display the sum of all numbers";
sum= sumAll(num);
cout<<"\nThe sum is "<<sum;
break;
case 2:cout<< "Display the sum of numbers in even subscripts";
sum=0;
for(x=0; x<=4; x=x+2)
{ sum = sum +num[x]; }
cout<<"\nThe sum is "<<sum;
break;
case 3:cout<< "Display the sum of even numbers";
sum=0;
for(x=0; x<=4; x++)
{if (num[x] % 2==0)
{
sum = sum +num[x];
} // if
} //for loop
cout<<"\nThe sum is "<<sum;
break;
case 8: cout<< "\nDisplay all numbers\n";
display(num);
break;
default : cout<<"\nYou entered an Invalid Number\n\n";
} //switch
} while (choice<=8);
} // end of main
//START OF FUNCTION
int sumAll(int num[5]) //case 1 Sum all numbers
{ sum=0;
for(x=0; x<=4; x++)
{ sum = sum +num[x]; }
return sum; }
void display(int num[5]) // case 8 display all numbers
{
for(x=0; x<=4; x++)
{ cout<<num[x] << " "; }
}
{ cout<<num[x] << " "; }
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Similar questions
- One dimension array in C:Create an array of 100 integer elements and initialize the array elements to zero.Populate the array (using a for loop) with the values 10,20,30,..., 990,1000.Write code (using for loops) to sum all the elements and output the sum to the screen.arrow_forwardplease give answer of all with proper explanation dont copy from googlearrow_forwardC++arrow_forward
- This program will display an array being passed to a function, show. The show function will display the elements of the given array. The main program controls the size and the inputs of an array. // function prototype void show(int [], int); int main(){ const int arraysize=5; int numbers[arraysize]={3,6,12,7,5}; show(numbers,arraysize); // (A) show(numbers,arraysize+1); return 0;} //function body void show(int nums[], int size){ for (int index=0;index<size; index++) cout<<nums[index]<<endl; } What is the output of this program? What is the output if modification (A) has been made?arrow_forwardC++ Dynamic Array Project Part 2arrow_forwardC++ Code: This function will print out the information that has been previously read (using the function readData) in a format that aligns an individual's STR counts along a column. For example, the output for the above input will be: name Alice Bob Charlie ---------------------------------------- AGAT 5 3 6 AATG 2 7 1 TATC 8 4 5 This output uses text manipulators to left-align each name and counts within 10 characters. The row of dashes is set to 40 characters. /** * Computes the longest consecutive occurrences of several STRs in a DNA sequence * * @param sequence a DNA sequence of an individual * @param nameSTRs the STRs (eg. AGAT, AATG, TATC) * @returns the count of the longest consecutive occurrences of each STR in nameSTRs **/ vector<int> getSTRcounts(string& sequence, vector<string>& nameSTRs) For example, if the sequence is AACCCTGCGCGCGCGCGATCTATCTATCTATCTATCCAGCATTAGCTAGCATCAAGATAGATAGATGAATTTCGAAATGAATGAATGAATGAATGAATGAATG and the vector namesSTRs is…arrow_forward
- Please complete the following guidelines and hints. Using C language. Please use this template: #include <stdio.h>#define MAX 100struct cg { // structure to hold x and y coordinates and massfloat x, y, mass;}masses[MAX];int readin(void){/* Write this function to read in the datainto the array massesnote that this function should return the number ofmasses read in from the file */}void computecg(int n_masses){/* Write this function to compute the C of Gand print the result */}int main(void){int number;if((number = readin()) > 0)computecg(number);return 0;}Testing your workTypical Input from keyboard:40 0 10 1 11 0 11 1 1Typical Output to screen:CoG coordinates are: x = 0.50 y = 0.50arrow_forwardWhat does the phrase "base address of an array" relate to, and how does it come to be utilized in a call to a function?arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
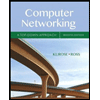
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
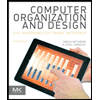
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
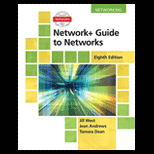
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
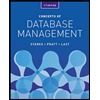
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
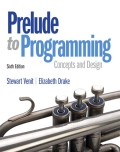
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
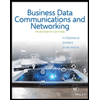
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY