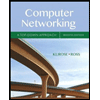
Dictionary-Returned Function
a) Define a function, dictSqrt() with a parameter, x:
1) Create a list, num and uses a loop to store, x numbers into the list in the range of (0, x).
2) Create a list, sqrtNum and store all sqrt of the numbers saved in the list above.
3) Use dict() and zip() to create a dictionary, sqrtDict, as the return value with numbers as the keys and their
corresponding sqrt as the values.
b) Define a main() function to do the following:
1) Get a random integer, n, in the range (1, 10).
2) Call dictSqrt() with the argument and print the returned dictionary.
c) Call main() function to initiate the tasks to be performed.
Example Output
dictSqrt(3)will print the following:
{0:0, 1:1, 2:1}
PYTHON

Step by stepSolved in 3 steps with 1 images

- C++arrow_forwardcreate a function in python parameters: 1. dict[str, list[str]] 2. list[str] return type: dict[str, list[str]] must do like this: 1. establish an empty dictionary that will serve as the dictionary returned at the end 2. loop thorugh each of the columns in the second parameter of the function 2a. asssign to the column key of the result dictionary the list of values stored in the input dictionary at the same column 3. return the dictionary produced do not import other libraries such as pandas, .select, .head, .iloc or anything this function is being used to work in a dataframearrow_forwarddef flight_sequences_overlap(path1: List[str], path2: List[str]) -> bool:"""Return True iff flight sequences path1 and path2 overlap: if the lastx number of stops (where x >= 1) of path1 are identical to thefirst x number of stops of path2.Precondition:- both path1 and path2 are valid flight sequences- the airport codes in each flight path is unique (i.e. we willnever have a path where we visit the same airport more than once) >>> path1 = ['AA1', 'AA2']>>> path2 = ['AA2', 'AA3']>>> flight_sequences_overlap(path1, path2)True>>> path3 = ['AA1', 'AA2', 'AA3']>>> path4 = ['AA2', 'AA3', 'AA4']>>> flight_sequences_overlap(path3, path4)True >>> path5 = ['AA2', 'AA3']>>> path6 = ['AA1', 'AA3', 'AA2']>>> flight_sequences_overlap(path5, path6)False""" passarrow_forward
- def get_task(task_collection, ...): """ param: task_collection (list) - holds all tasks; maps an integer ID/index to each task object (a dictionary) param: ... (str) - a string that is supposed to represent an integer ID that corresponds to a valid index in the collection returns: 0 - if the collection is empty. -1 - if the provided parameter is not a string or if it is not a valid integer Otherwise, convert the provided string to an integer; returns None if the ID is not a valid index in the list or returns the existing item (dict) that was requested. """arrow_forwardQ2arrow_forwardYou can create an empty dictionary with dictionary() [ ] ( ) { } This data structure stores a collection of objects in an unordered manner where each object must be unique dictionary set tuple listarrow_forward
- PYTHON: NEED CORRECTION 11) vector_add, takes two lists of the same size and adds the elements together. 12) Do subtraction, division, and multiplication for number 11. def vector_add(numList1, numList2): numList.append(numList1, numList2) return(numList) def sub_div_mult(numList): numList=list_sub(numList, 2) numList=list_divide(numList,2) numList=list_multuply(numList2) return numListarrow_forwardPython function getData(fname:str)->ndarray that inputs data from a file into a list, then converts the list to a NumPy array of integer values and returns the array. The text file (fname) contains: integer numbers, one per line numbers are in the range 0 <= n <= 99 r * c (rows * columns) quantity of numbers, where c = r + 1 Example: 15 22 65 4 78 91 7 35 60 16 99 12 == [[15 22 65 4] [78 91 7 35] [60 16 99 12]]arrow_forward# Using the routes dictionary defining places your airline can go with #with mileage to each, create a function that determines the total #distance travelled by going to the airports in sequence defined by this # list itinerary and the routes dictionary. (No published answer... def summary (routesMap) : # Please do not change any code below... airports = list (routesMap.keys ()) for airport in airports: destinations = routesMap [airport] for n in range (0,len (destinations)): destinationInfo = destinations [n] destination = destinationInfo [0] mileage = destinationInfo [1] print (f"Leaving {airport} to {destination} for (mileage} miles.") def distanceUsing (routesMap, itinerary): milesTravelled = 0 ### Your code goes here. return milesTravelled def main (): # Please do not change this dictionary... routes = { main () "Austin": "Chicago": "Dallas": "Denver": "El Paso": "Houston": "Nashville": summary (routes) [["Chicago", 500], ["Houston", 180], ["Nashville", 86011, [["Austin", 500],…arrow_forward
- Make new code pleasearrow_forwardstruct insert_at_back_of_sll { // Function takes a constant Book as a parameter, inserts that book at the // back of a singly linked list, and returns nothing. void operator()(const Book& book) { /// TO-DO (3) /// // Write the lines of code to insert "book" at the back of "my_sll". Since // the SLL has no size() function and no tail pointer, you must walk the // list looking for the last node. // // HINT: Do not attempt to insert after "my_sll.end()". // ///// END-T0-DO (3) ||||// } std::forward_list& my_sll; };arrow_forwardWrite a function in_list that will take as its parameters an input list called mylist and a number. If the number is in the list the function should retun true, ottherwise, it should retun false. def in_list(mylist,number):arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
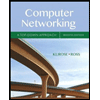
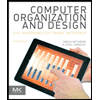
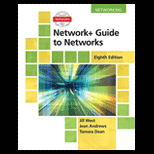
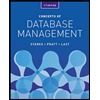
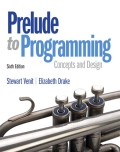
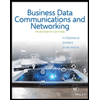