Design a program that uses two-dimensional arrays, classes and objects Assignment: Write a program that plays a tic-tac-toe game with the user. Define a class TicTacToe with the necessary data and functions to play the game. Use a 2-D char array with three rows and three columns as the game board. The class should also have the following functions: A constructor to initialize the board. I suggest either asterisk ('*') or characters '1' through '9'. A function to play the user's turn, fill in the board with either an 'X' or 'O' given row and column number (1-3) A function to play the computer's turn. This function will determine the row and column number to be filled in. You can use a simple algorithm such as look for the first space available, or random number generation. Complex AI algorithms will only be accepted with an explanation of its behavior via a zoom meeting. A function to verify if there is a win. This can be broken down by calling 3 separate private functions that can verify column win, row win or diagonal win. If there is no winner, let the user know there is a tie. The main function to test your class is given. Note that all member functions that you need to call in main through the TicTacToe object should be public, but functions needed only inside your class implementation (called only by other functions in your class) should be private. You can decide who will have 'X' or 'O'. Have fun!
Objective:
Design a program that uses two-dimensional arrays, classes and objects
Assignment:
Write a program that plays a tic-tac-toe game with the user.
Define a class TicTacToe with the necessary data and functions to play the game. Use a 2-D char array with three rows and three columns as the game board. The class should also have the following functions:
- A constructor to initialize the board. I suggest either asterisk ('*') or characters '1' through '9'.
- A function to play the user's turn, fill in the board with either an 'X' or 'O' given row and column number (1-3)
- A function to play the computer's turn. This function will determine the row and column number to be filled in. You can use a simple
algorithm such as look for the first space available, or random number generation. ComplexAI algorithms will only be accepted with an explanation of its behavior via a zoom meeting. - A function to verify if there is a win. This can be broken down by calling 3 separate private functions that can verify column win, row win or diagonal win. If there is no winner, let the user know there is a tie.
The main function to test your class is given.
Note that all member functions that you need to call in main through the TicTacToe object should be public, but functions needed only inside your class implementation (called only by other functions in your class) should be private.
You can decide who will have 'X' or 'O'.
Have fun!
Turn in:
- TicTacToe.h (or .hpp) and TicTacToe.cpp with the implementation of your class.
TicTacToe_main.cpp:
/********************************************************
Description: Tic-Tac-Toe driver program
Author: Hellen Pacheco
Date: October, 2020
********************************************************/
#include "TicTacToe.h"
#include <iostream>
using namespace std;
enum gameStatusType { USER, COMPUTER, TIE, INPROGRESS };
// values of gameStatusType are USER=0, COMPUTER=1, TIE=2, INPROGRESS=3
int main()
{
int row, col;
int gameStatus;
TicTacToe tttGame; // instantiates a Tic-tac-toe object
cout<<"Hello, welcome to the Tic-Tac-Toe game."<<endl;
do
{
tttGame.printBoard();
cout<<"Please enter row#: "<<endl;
cin >> row;
cout<<"Please enter col#: "<<endl;
cin >> col;
tttGame.userMove(row, col);
tttGame.computerMove();
gameStatus = tttGame.determineWinner();
switch (gameStatus)
{
case USER : cout << "You won!"; break;
case COMPUTER : cout << "Computer won!"; break;
case TIE: cout << "It is a tie!"; break;
case INPROGRESS: continue;
}
} while (gameStatus == INPROGRESS);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

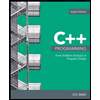
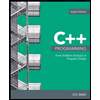