Design a Java interface called Priority that includes two methods: setPriority and getPriority. This interface would define a way to establish numeric priority among a set of objects. Your numeric priority values should be on a scale. For easy comparing of priorities, make 1 the lowest priority. It should be more complex than just 1, 2, 3 (low, medium, high). Define constants in the interface for the low, medium, and high priority values. Design and implement a class called Task that represents a task (e.g., something on a to-do list) that implements the Priority interface from above, as well as the Comparable interface from the Java standard class library. A task should have some sort of description. Illustrate your design with a UML class diagram. Create a driver whose main method exercises some Task objects. Make sure you have enough tasks to produce comparisons where a given task is higher, lower, or equal in priority to some other task. Implement the interface such that the tasks are ranked by priority. Create a driver class that shows these new features of Task objects.
-
Design a Java interface called Priority that includes two methods: setPriority and getPriority. This interface would define a way to establish numeric priority among a set of objects. Your numeric priority values should be on a scale. For easy comparing of priorities, make 1 the lowest priority. It should be more complex than just 1, 2, 3 (low, medium, high). Define constants in the interface for the low, medium, and high priority values. Design and implement a class called Task that represents a task (e.g., something on a to-do list) that implements the Priority interface from above, as well as the Comparable interface from the Java standard class library. A task should have some sort of description. Illustrate your design with a UML class diagram. Create a driver whose main method exercises some Task objects. Make sure you have enough tasks to produce comparisons where a given task is higher, lower, or equal in priority to some other task. Implement the interface such that the tasks are ranked by priority. Create a driver class that shows these new features of Task objects.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

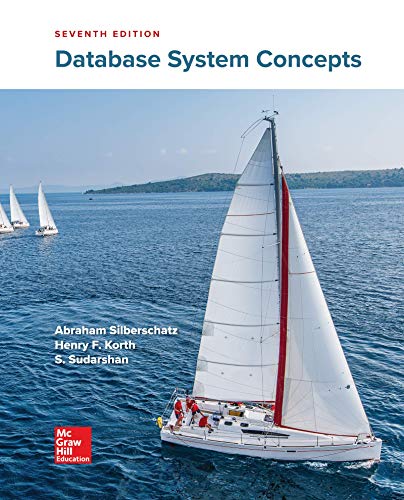
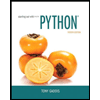
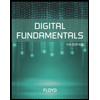
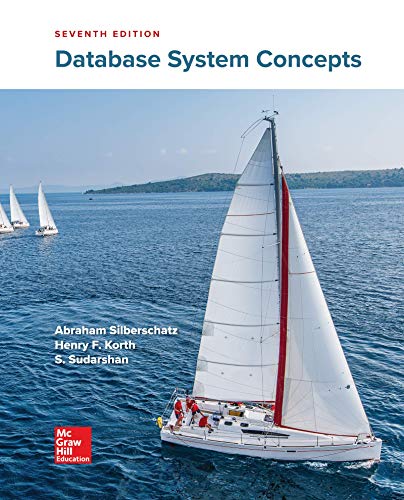
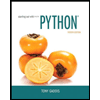
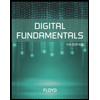
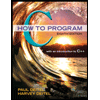
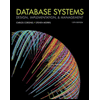
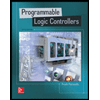