Design a function bool validateStackSequences(const std::vector& v) that takes an int vector with distinct values, and returns if values in v could be a valid sequence of stack operations You may assume the input values are always{1, 2, 3, 4, 5}. #include #include #include // TODO: implement this function bool validateStackSequences(const std::vector& v) { if (v.size() % 2 != 0) return false; std::stack s; int i = 0; int j = v.size() - 1; while (i <= j) { if (v[i] == v[j]) { i++; j--; } else if (!s.empty() && s.top() == v[j]) { s.pop(); j--; } else { s.push(v[i]); i++; } } return s.empty(); } int main(){ // use following code snippet for testing // expected output: // true // false // true // false // true std::cout << std::boolalpha << validateStackSequences({5, 4, 3, 2, 1}) << std::endl; std::cout << std::boolalpha << validateStackSequences({5, 4, 3, 1, 2}) << std::endl; std::cout << std::boolalpha << validateStackSequences({3, 2, 4, 1, 5}) << std::endl; std::cout << std::boolalpha << validateStackSequences({4, 2, 3, 1, 5}) << std::endl; std::cout << std::boolalpha << validateStackSequences({1, 2, 3, 4, 5}) << std::endl; return 0; } PLEASE MAKE CHANGES IN THE ABOVE CODE
Design a function bool validateStackSequences(const std::vector<int>& v) that takes an int vector with distinct values, and returns if values in v could be a valid sequence of stack operations
You may assume the input values are always{1, 2, 3, 4, 5}.
#include <vector>
#include <stack>
#include <iostream>
// TODO: implement this function
bool validateStackSequences(const std::vector<int>& v)
{
if (v.size() % 2 != 0)
return false;
std::stack<int> s;
int i = 0;
int j = v.size() - 1;
while (i <= j)
{
if (v[i] == v[j])
{
i++;
j--;
}
else if (!s.empty() && s.top() == v[j])
{
s.pop();
j--;
}
else
{
s.push(v[i]);
i++;
}
}
return s.empty();
}
int main(){
// use following code snippet for testing
// expected output:
// true
// false
// true
// false
// true
std::cout << std::boolalpha << validateStackSequences({5, 4, 3, 2, 1}) << std::endl;
std::cout << std::boolalpha << validateStackSequences({5, 4, 3, 1, 2}) << std::endl;
std::cout << std::boolalpha << validateStackSequences({3, 2, 4, 1, 5}) << std::endl;
std::cout << std::boolalpha << validateStackSequences({4, 2, 3, 1, 5}) << std::endl;
std::cout << std::boolalpha << validateStackSequences({1, 2, 3, 4, 5}) << std::endl;
return 0;
}
PLEASE MAKE CHANGES IN THE ABOVE CODE

Step by step
Solved in 3 steps with 1 images

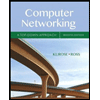
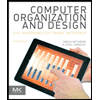
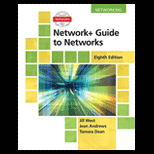
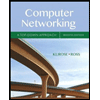
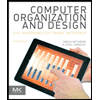
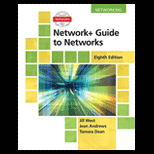
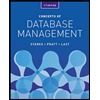
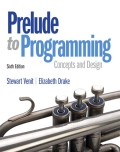
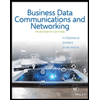