Design a class named Time. The class contains:■ The private data fields hour, minute, and second that represent a time.■ A constructor that constructs a Time object that initializes hour, minute, and second using the current time.■ The get methods for the data fields hour, minute, and second, respectively.■ A method named setTime(elapseTime) that sets a new time for the object using the elapsed time in seconds. For example, if the elapsed time is 555550 seconds, the hour is 10, the minute is 19, and the second is 12.Draw the UML diagram for the class, and then implement the class. Write a test program that creates a Time object and displays its hour, minute, and second.Your program then prompts the user to enter an elapsed time, sets its elapsed time in the Time object, and displays its hour, minute, and second. Here is a sample run: Current time is 12:41:6Enter the elapsed time:55550505The hour:minute:second for the elapsed time is 22:41:45
Design a class named Time. The class contains:
■ The private data fields hour, minute, and second that represent a time.
■ A constructor that constructs a Time object that initializes hour, minute, and second using the current time.
■ The get methods for the data fields hour, minute, and second, respectively.
■ A method named setTime(elapseTime) that sets a new time for the object using the elapsed time in seconds. For example, if the elapsed time is 555550 seconds, the hour is 10, the minute is 19, and the second is 12.
Draw the UML diagram for the class, and then implement the class. Write a test
Your program then prompts the user to enter an elapsed time, sets its elapsed time in the Time object, and displays its hour, minute, and second. Here is a sample run:
Current time is 12:41:6
Enter the elapsed time:55550505
The hour:minute:second for the elapsed time is 22:41:45

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

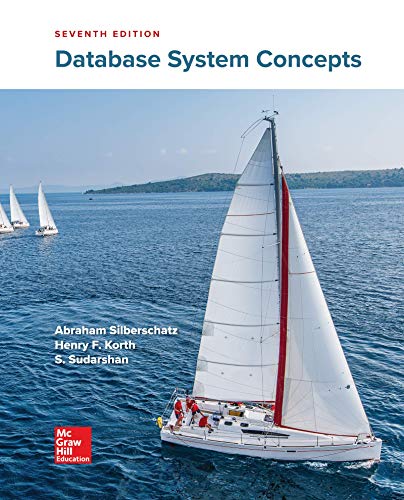
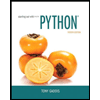
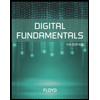
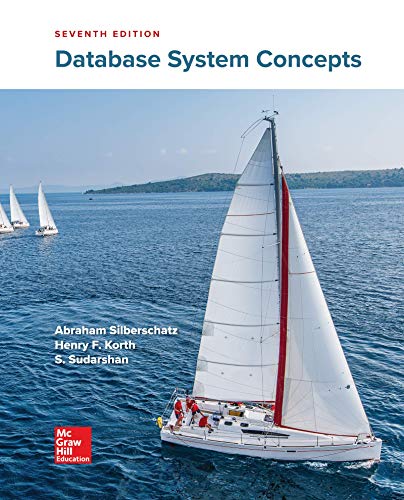
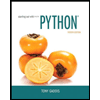
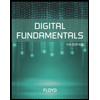
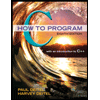
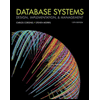
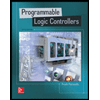