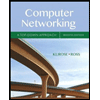
Instructions
Define the class bankAccount to implement the basic properties of a bank account. An object of this class should store the following data: Account holder’s name (string), account number (int), account type (string, checking/saving), balance (double), and interest rate (double). (Store interest rate as a decimal number.) Add appropriate member functions to manipulate an object. Use a static member in the class to automatically assign account numbers. Also, declare an array of 10 components of type bankAccount to process up to 10 customers and write a program to illustrate how to use your class.
Example output is shown below:
1: Enter 1 to add a new customer.
2: Enter 2 for an existing customer.
3: Enter 3 to print customers data.
9: Enter 9 to exit the program.
1
Enter customer's name: Dave Brown
Enter account type (checking/savings): checking
Enter amount to be deposited to open account: 10000
Enter interest rate (as a percent): .01
1: Enter 1 to add a new customer.
2: Enter 2 for an existing customer.
3: Enter 3 to print customers data.
9: Enter 9 to exit the program.
3
Account Holder Name: Dave Brown
Account Type: checking
Account Number: 1100
Balance: $10000.00
Interest Rate: 0.01%
*****************************
1: Enter 1 to add a new customer.
2: Enter 2 for an existing customer.
3: Enter 3 to print customers data.
9: Enter 9 to exit the program.
9
Since your program handles currency, make sure to use a data type that can store decimals with a precision to 2 decimals.
P.S. There are 3 tabs
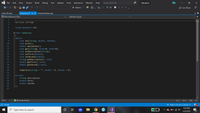
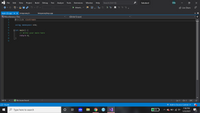

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- C++arrow_forwardComputer programmingarrow_forwardExercise 1-Account class • Design a class named Account that contains : • A private int data field named id for the account • A private double data field named balance for the account • A privet Date data field named dateCreated that stores the date when the account was created • A no-arg constructor that creates a default account • A constructor that creates an account with the specified id and initial balance • The getters (i.e., accessors) and setters (i.e., mutators) methods for id and balance • The getter method for dateCreated • A method named withdraw that withdraws a specified amount from the account • A method named deposit that deposits a specified amount to the accountarrow_forward
- It is possible to express the relationship that exists between classes and objects.arrow_forwardclass IndexItem { public: virtual int count() = 0; virtual void display()= 0; };class Book : public IndexItem { private: string title; string author; public: Book(string title, string author): title(title), author(author){} virtual int count(){ return 1; } virtual void display(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };class Category: public IndexItem { private: /* fill in the private member variables for the Category class below */ ? int count; public: Category(string name, string code): name(name), code(code){} /* Implement the count function below. Consider the use of the function as depicted in main() */ ? /* Implement the add function which fills the category with contents below. Consider the use of the function as depicted in main() */ ? virtualvoiddisplay(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };arrow_forwardCreate an Account class that a bank might use to represent customers’ bank accounts. Include a data member to represent the account balance. Provide a constructor that receives an initial balance and uses it to initialize the data member. The constructor should validate the initial balance to ensure that it is greater than or equal to 0. If not, set the balance to 0. Provide three member functions:- credit($amount) should add amount to the current balance- debit($amount) should ensure that amount does not exceed the current balance and then reduce the current balance by amount. The balance should not change if amount exceeds the balance.- getBalance() should return the current balanceNote: Create a set of test data and then write a program to test the class.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
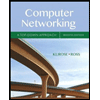
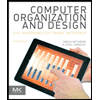
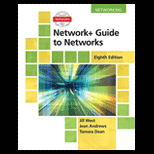
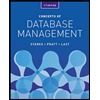
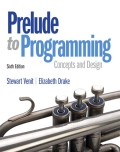
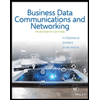