Hello I need help fixing lines 16 and line 41. I'm not sure how to fix an UnsupportedOperation error. Here are the instructions: You must have a function that accepts three test scores and returns the float average You will calculate the average for an unknown number of students, by reading the data from a file named scores.txt. You must print the students’ average scores to the screen as shown above You must write the specified output to a file named grades.txt You may not use any global variables
Hello I need help fixing lines 16 and line 41. I'm not sure how to fix an UnsupportedOperation error. Here are the instructions: You must have a function that accepts three test scores and returns the float average You will calculate the average for an unknown number of students, by reading the data from a file named scores.txt. You must print the students’ average scores to the screen as shown above You must write the specified output to a file named grades.txt You may not use any global variables
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 6PE
Related questions
Question
Hello I need help fixing lines 16 and line 41. I'm not sure how to fix an UnsupportedOperation error.
Here are the instructions:
- You must have a function that accepts three test scores and returns the float average
- You will calculate the average for an unknown number of students, by reading the data from a file named scores.txt.
- You must print the students’ average scores to the screen as shown above
- You must write the specified output to a file named grades.txt
- You may not use any global variables

Transcribed Image Text:The input will come from a text file named scores.txt, which consists of multiple sets of a student ID
number followed by three test scores. The contents of a file containing the scores of four students are
shown below (the student ID number is of the form MXX where XX is a two-digit number):
M01
76
89
82
M02
91
81
83
M03
92
93
M02 85.00
M03 91.67
M04 88.33
90
M04
86
88
91
The output of the above data to the screen will be of the following form (note: 2 decimal places):
Student M01 average is 82.33
Student M02 average is 85.00
Student M03 average is 91.67
Student M04 average is 88.33
Only the student ID number and average score (separated by a space and formatted to 2 decimal places)
are written to the output file:
M01 82.33

Transcribed Image Text:6 def calc_average (scorel, score2, score3):
7
n = 0
8
9
10
11
12
B 14 15 16 17 18 19 28 21 22 23 24 25 26 27 28 298128343567839 48 1 2 3 4 45 45
13
20
30
40
41
42
43
44
def
46
read_file
out_file =
for i in range (n):
out_file.write(str(n))
out_file.write("\n")
out_file.close()
inFile =
open("grades.txt", "w")
line = inFile.readlines ()
count = 0
str(input("Enter the name of the file to which the final results can be read:"))
open("scores.txt",
"r")
avg_total = 0
=
for i in range(n):
score2
student = input("Name: ")
count + 1
score1
= 0
= 0
score3 = 0
int(line)
avg_total
print (name, avg_total)
inFile.close()
main ():
file_name
45 main()
student =
return avg_total
i = 0
=
=
student_count = 0
str(input ("Enter the name of the file to which results should've been written: "))
while student_count !=
score1 = 0
score2 = 0
1
score3 = 0
student_count +=1
avg_total calc_average (scorel, score2, score3)
print('Student', student, 'average', avg_total)
=
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
Hello i can see the output in the terminal but nothing appears in grades.txt
![1 def calc_average (score1, score2, score3):
return (score1+score2+score3)/3
2
3
4 def main():
4567 00
8
9
10
11
12
13
14
15
16
17
file_name=input("Enter a file name for reading a data: ")
score_file = open("scores.txt", "r")
file_name2 =input("Enter a file name for writting a data: ")
grade_file =open("grades", "w")
datal-score_file.read().split("\n")
length=len (data1)
if(len (data1) %4!=0):
length=len (data1)-1
for i in range (0, length, 4):
num=data1[i]
scorel=int (datal[i+1])
score2=int (datal[i+2])
score3=int (data1[i+3])
avg=calc_average (score1, score2, score3)
18
19
20
21
22
23
24
25
26 main()
print("Student {} average is {:0.2f}" .format(num, avg))
string= num+" "+"{:.2f}".format(avg)
grade_file.write(string+"\n")](https://content.bartleby.com/qna-images/question/3eb03a80-7cae-4d33-b228-e2e0361c370e/83fc8e06-b169-4976-aa27-c5418237fed4/bk533tn_thumbnail.png)
Transcribed Image Text:1 def calc_average (score1, score2, score3):
return (score1+score2+score3)/3
2
3
4 def main():
4567 00
8
9
10
11
12
13
14
15
16
17
file_name=input("Enter a file name for reading a data: ")
score_file = open("scores.txt", "r")
file_name2 =input("Enter a file name for writting a data: ")
grade_file =open("grades", "w")
datal-score_file.read().split("\n")
length=len (data1)
if(len (data1) %4!=0):
length=len (data1)-1
for i in range (0, length, 4):
num=data1[i]
scorel=int (datal[i+1])
score2=int (datal[i+2])
score3=int (data1[i+3])
avg=calc_average (score1, score2, score3)
18
19
20
21
22
23
24
25
26 main()
print("Student {} average is {:0.2f}" .format(num, avg))
string= num+" "+"{:.2f}".format(avg)
grade_file.write(string+"\n")
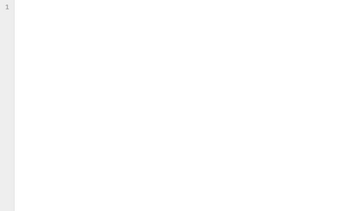
Transcribed Image Text:1
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
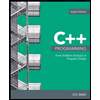
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
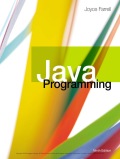
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
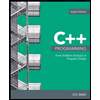
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
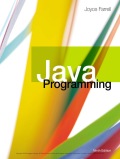
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT