Create the following functions makeBoard Accepts two parameters, integers mm and nn, and creates an mm x nn numpy array of zeros Returns the created array makeMove Accepts two parameters, a 2D array (such as created by makeBoard), and an integer xx Checks if the value at position xx in the array is 0. If it is, changes it to 1 and returns True If it isn't, does not change it and returns False Position xx in the array is given by reading the array top-down and left-to right. A simple 2x3 array is seen below with each value representing its position You may assume without checking that the value of xx provided is valid, that is it is within the array. Hint: Arrays have a property you can use to determine their shape. Hint: This function may (and indeed should) contain if statements, but no loops. Sample array with positions: [[0 1 2] [ 3 4 5]] For testing purposes, you may copy the function provided here into your code def playGame(mm,nn): board = makeBoard(mm,nn) play = True while play: pos = np.random.randint(0,mm*nn) print(f"Move to position {pos}") play = makeMove(board,pos) print(board) print('Game Over')
Create the following functions makeBoard Accepts two parameters, integers mm and nn, and creates an mm x nn numpy array of zeros Returns the created array makeMove Accepts two parameters, a 2D array (such as created by makeBoard), and an integer xx Checks if the value at position xx in the array is 0. If it is, changes it to 1 and returns True If it isn't, does not change it and returns False Position xx in the array is given by reading the array top-down and left-to right. A simple 2x3 array is seen below with each value representing its position You may assume without checking that the value of xx provided is valid, that is it is within the array. Hint: Arrays have a property you can use to determine their shape. Hint: This function may (and indeed should) contain if statements, but no loops. Sample array with positions: [[0 1 2] [ 3 4 5]] For testing purposes, you may copy the function provided here into your code def playGame(mm,nn): board = makeBoard(mm,nn) play = True while play: pos = np.random.randint(0,mm*nn) print(f"Move to position {pos}") play = makeMove(board,pos) print(board) print('Game Over')
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Create the following functions
- makeBoard
- Accepts two parameters, integers mm and nn, and creates an mm x nn numpy array of zeros
- Returns the created array
- makeMove
- Accepts two parameters, a 2D array (such as created by makeBoard), and an integer xx
- Checks if the value at position xx in the array is 0.
- If it is, changes it to 1 and returns True
- If it isn't, does not change it and returns False
- Position xx in the array is given by reading the array top-down and left-to right. A simple 2x3 array is seen below with each value representing its position
- You may assume without checking that the value of xx provided is valid, that is it is within the array.
- Hint: Arrays have a property you can use to determine their shape.
- Hint: This function may (and indeed should) contain if statements, but no loops.
Sample array with positions:
[[0 1 2]
[ 3 4 5]]
For testing purposes, you may copy the function provided here into your code
def playGame(mm,nn): board = makeBoard(mm,nn) play = True while play: pos = np.random.randint(0,mm*nn) print(f"Move to position {pos}") play = makeMove(board,pos) print(board) print('Game Over') |
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Recommended textbooks for you
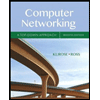
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
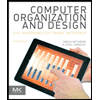
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
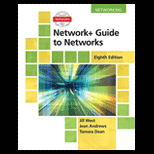
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
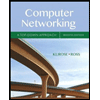
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
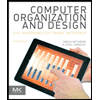
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
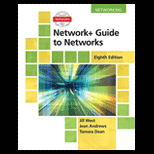
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
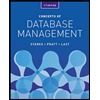
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
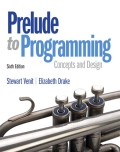
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
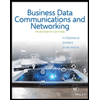
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY