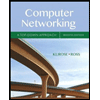
Create an abstract class called Movie. The class should declare the following variables:
- an instance variable that describes the title - String
- an instance variable that describes the rating - double
- an instance variable that describes the director - String
- an instance variable that describes the genre - String
- an instance variable that describes the year – integer
- an instance variable that describes the language – String
- an instance variable that describes the ticket price - double
Create the getter and setter methods for each instance variable except setter for ticket price and getter for genre. Create the necessary constructors. Include an abstract method setTicketPrice(double ticketPrice) to determine the ticket price for a movie. Include an abstract method getGenre() to return the genre of the movie. Provide a toString() method that returns the information stored in the above variables.
Create three subclasses called ThrillerMovie, ComedyMovie, and ChildrenMovie.
These subclasses should override the abstract methods setTicketPrice and getGenre of class Movie.
Use the following rule for setting the ticket price for a movie:
- Children movies will have a 10% discount
- Thriller movies will have a fixed price (specified by user)
- Comedy movies will have a 5% discount
Write a driver program (another class with main method) that uses the above hierarchy. In your driver program you must implement an interaction with the user.
- Use showInputDialog method to let the user input movie information.
- Use showMessageDialog method to display movie information including price and type for movies.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- in the date classOverload the constructor method as the class diagram showsIn the main program, create three objects of class date, one from each of the constructors.In the main program, overload the showdate method to display the date in the following formatsThe date in dd/mm/yy formatThe date in dd/mm formatThe date in “d of month” formatIn the main program, make use of each of the showdate methods, one with each of the created objects.Send your two files, the date file and the main program where you use the date constructor overload and the showdate method overload.arrow_forwardclass Widget: """A class representing a simple Widget === Instance Attributes (the attributes of this class and their types) === name: the name of this Widget (str) cost: the cost of this Widget (int); cost >= 0 === Sample Usage (to help you understand how this class would be used) === >>> my_widget = Widget('Puzzle', 15) >>> my_widget.name 'Puzzle' >>> my_widget.cost 15 >>> my_widget.is_cheap() False >>> your_widget = Widget("Rubik's Cube", 6) >>> your_widget.name "Rubik's Cube" >>> your_widget.cost 6 >>> your_widget.is_cheap() True """ # Add your methods here if __name__ == '__main__': import doctest # Uncomment the line below if you prefer to test your examples with doctest # doctest.testmod()arrow_forwardThe non-static class level variables and non-static methods of a class are known as a. instance members b. object behaviors c. object attributes O d. object referencesarrow_forward
- Exercise 1-Account class • Design a class named Account that contains : • A private int data field named id for the account • A private double data field named balance for the account • A privet Date data field named dateCreated that stores the date when the account was created • A no-arg constructor that creates a default account • A constructor that creates an account with the specified id and initial balance • The getters (i.e., accessors) and setters (i.e., mutators) methods for id and balance • The getter method for dateCreated • A method named withdraw that withdraws a specified amount from the account • A method named deposit that deposits a specified amount to the accountarrow_forward1- Design a class named Rectangle to represent a Rectangle. The class contains: • three variables integer data field named height, integer data filed named width and integer data filed named length. The default values are 1 for height, length, and width are 1.0 for each of them respectively. • A no-arg constructor that creates a default Rectangle. • A constructor that creates a Rectangle with the specified height, length and width. • A method named getVolume() that returns the volume of this Rectangle. (Volume = height * width * length) %3! 2- Write a test program called Test Rectangle that: • Creates two Rectangle objects: one object with height 11, length 5, and width 7, the other object with height 10, length 2 and width 6 • Display the volume of each Rectangle object.arrow_forwardDescribe the class's private and public components.arrow_forward
- Design a Ship class that has the following members:• A field for the name of the ship (a string).• A field for the year that the ship was built (a string).• A constructor and appropriate accessors and mutators.• A toString method that displays the ship's name and the year it was built.Disign a CruiseShip class that extends the Ship class. The CruiseShip class should have thefollowing members:• A field for the maximum number of passengers (an int).• A constructor and appropriate accessors and mutators.• A toString method that overrides the toString method in the base class. The CruiseShipclass's toString method should display only the ship's name and the maximum number ofpassengers.Design a CargoShip class that extends the Ship class. The CargoShip class should have thefollowing members:• A field for the cargo capacity in tonnage (an int).• A constructor and appropriate accessors and mutators.• A toString method that overrides the toString method in the base class. The CargoShipclass's…arrow_forwardclass IndexItem { public: virtual int count() = 0; virtual void display()= 0; };class Book : public IndexItem { private: string title; string author; public: Book(string title, string author): title(title), author(author){} virtual int count(){ return 1; } virtual void display(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };class Category: public IndexItem { private: /* fill in the private member variables for the Category class below */ ? int count; public: Category(string name, string code): name(name), code(code){} /* Implement the count function below. Consider the use of the function as depicted in main() */ ? /* Implement the add function which fills the category with contents below. Consider the use of the function as depicted in main() */ ? virtualvoiddisplay(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
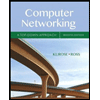
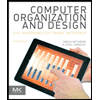
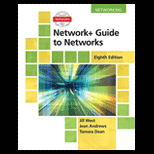
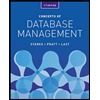
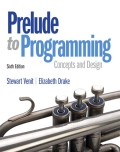
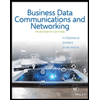