create a Word document that contains the pseudocode, flowchart, and test plan for (Programming Projects 3( import java.util.Scanner; public class Exercise08_13 { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter the number of rows and columns of the array: "); int rows = input.nextInt(); int columns = input.nextInt(); double[][] array = new double[rows][columns]; System.out.println("Enter the array:"); for (int i = 0; i < rows; i++) { for (int j = 0; j < columns; j++) { array[i][j] = input.nextDouble(); } } int[] location = locateLargest(array); System.out.println("The location of the largest element is at (" + location[0] + ", " + location[1] + ")"); } public static int[] locateLargest(double[][] a) { int[] location = new int[2]; double largest = a[0][0]; for (int i = 0; i < a.length; i++) { for (int j = 0; j < a[i].length; j++) { if (a[i][j] > largest) { largest = a[i][j]; location[0] = i; location[1] = j; } } } return location; } }) and 5( import java.util.Scanner; public class Exercise08_21 { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter the number of cities: "); int numCities = input.nextInt(); double[][] coordinates = new double[numCities][2]; System.out.print("Enter the coordinates of the cities: "); for (int i = 0; i < numCities; i++) { for (int j = 0; j < 2; j++) { coordinates[i][j] = input.nextDouble(); } } int centralCityIndex = findCentralCity(coordinates); System.out.println("The central city is at (" + coordinates[centralCityIndex][0] + ", " + coordinates[centralCityIndex][1] + ")"); double totalDistance = calculateTotalDistance(coordinates, centralCityIndex); System.out.println("The total distance to all other cities is " + totalDistance); } public static int findCentralCity(double[][] coordinates) { int centralCityIndex = 0; double smallestTotalDistance = Double.MAX_VALUE; for (int i = 0; i < coordinates.length; i++) { double totalDistance = calculateTotalDistance(coordinates, i); if (totalDistance < smallestTotalDistance) { smallestTotalDistance = totalDistance; centralCityIndex = i; } } return centralCityIndex; } public static double calculateTotalDistance(double[][] coordinates, int centralCityIndex) { double totalDistance = 0; for (int i = 0; i < coordinates.length; i++) { if (i != centralCityIndex) { double distance = calculateDistance( coordinates[centralCityIndex][0], coordinates[centralCityIndex][1], coordinates[i][0], coordinates[i][1]); totalDistance += distance; } } return totalDistance; } public static double calculateDistance(double x1, double y1, double x2, double y2) { return Math.sqrt(Math.pow(x2 - x1, 2) + Math.pow(y2 - y1, 2)); } }) from Chapter 8) that you completed in Revel.
create a Word document that contains the pseudocode, flowchart, and test plan for (
import java.util.Scanner;
public class Exercise08_13 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter the number of rows and columns of the array: ");
int rows = input.nextInt();
int columns = input.nextInt();
double[][] array = new double[rows][columns];
System.out.println("Enter the array:");
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
array[i][j] = input.nextDouble();
}
}
int[] location = locateLargest(array);
System.out.println("The location of the largest element is at (" + location[0] + ", " + location[1] + ")");
}
public static int[] locateLargest(double[][] a) {
int[] location = new int[2];
double largest = a[0][0];
for (int i = 0; i < a.length; i++) {
for (int j = 0; j < a[i].length; j++) {
if (a[i][j] > largest) {
largest = a[i][j];
location[0] = i;
location[1] = j;
}
}
}
return location;
}
})
and 5(
import java.util.Scanner;
public class Exercise08_21 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter the number of cities: ");
int numCities = input.nextInt();
double[][] coordinates = new double[numCities][2];
System.out.print("Enter the coordinates of the cities: ");
for (int i = 0; i < numCities; i++) {
for (int j = 0; j < 2; j++) {
coordinates[i][j] = input.nextDouble();
}
}
int centralCityIndex = findCentralCity(coordinates);
System.out.println("The central city is at (" +
coordinates[centralCityIndex][0] + ", " +
coordinates[centralCityIndex][1] + ")");
double totalDistance = calculateTotalDistance(coordinates, centralCityIndex);
System.out.println("The total distance to all other cities is " + totalDistance);
}
public static int findCentralCity(double[][] coordinates) {
int centralCityIndex = 0;
double smallestTotalDistance = Double.MAX_VALUE;
for (int i = 0; i < coordinates.length; i++) {
double totalDistance = calculateTotalDistance(coordinates, i);
if (totalDistance < smallestTotalDistance) {
smallestTotalDistance = totalDistance;
centralCityIndex = i;
}
}
return centralCityIndex;
}
public static double calculateTotalDistance(double[][] coordinates, int centralCityIndex) {
double totalDistance = 0;
for (int i = 0; i < coordinates.length; i++) {
if (i != centralCityIndex) {
double distance = calculateDistance(
coordinates[centralCityIndex][0], coordinates[centralCityIndex][1],
coordinates[i][0], coordinates[i][1]);
totalDistance += distance;
}
}
return totalDistance;
}
public static double calculateDistance(double x1, double y1, double x2, double y2) {
return Math.sqrt(Math.pow(x2 - x1, 2) + Math.pow(y2 - y1, 2));
}
})
from Chapter 8) that you completed in Revel.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

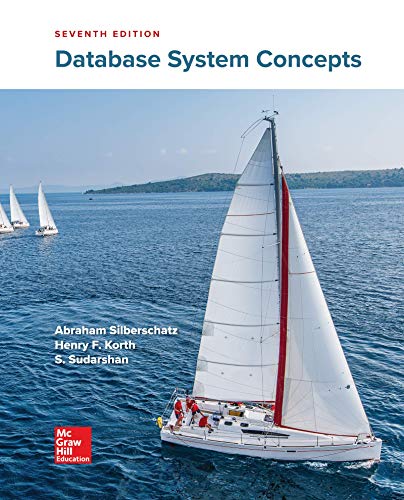
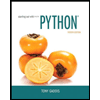
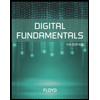
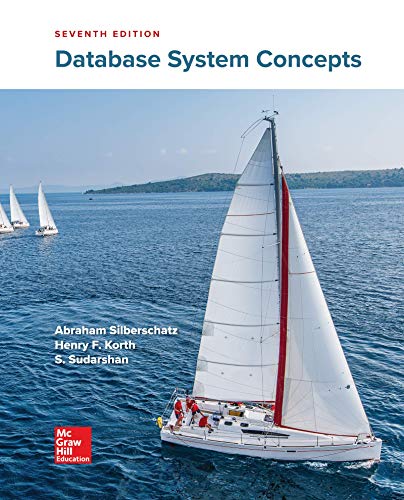
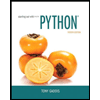
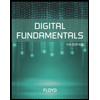
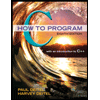
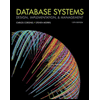
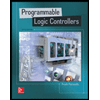