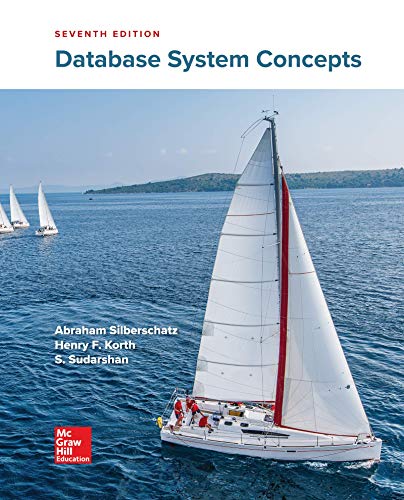
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Modify the following code to add a new function called “delete_target” that will delete the
target value from the array and update the array accordingly.
Code:
#include <stdio.h>
#define NOT_FOUND -1
int search(const int arr[], int target, int n);
int main(){
int s[] = {1,2,3,4,5,6,10};
int index;
int ID_SIZE = 7;
index = search(s,10,ID_SIZE);
printf("%d",index);
return 0;
}
int
search(const int arr[],
int target,
int n)
[15]
{
int i,
found = 0,
where;
i = 0;
while (!found && i < n) {
if (arr[i] == target)
found = 1;
else
++i;
}
if (found)
where = i;
else
where = NOT_FOUND;
return (where);
}
SAVE
AI-Generated Solution
info
AI-generated content may present inaccurate or offensive content that does not represent bartleby’s views.
Unlock instant AI solutions
Tap the button
to generate a solution
to generate a solution
Click the button to generate
a solution
a solution
Knowledge Booster
Similar questions
- The question describes a function S(k) which is defined as the sum of the positive divisors of a positive integer k, minus k itself. The function S(1) is defined as 1, and for any positive integer k greater than 1, S(k) is calculated as S(k) = σ(k) - k, where σ(k) is the sum of all positive divisors of k. Some examples of S(k) are given: S(1) = 1 S(2) = 1 S(3) = 1 S(4) = 3 S(5) = 1 S(6) = 6 S(7) = 1 S(8) = 7 S(9) = 4 The question then introduces a recursive sequence a_n with the following rules: a_1 = 12 For n ≥ 2, a_n = S(a_(n-1)) Part (a) of the question asks to calculate the values of a_2, a_3, a_4, a_5, a_6, a_7, and a_8 for the sequence. Part (b) modifies the sequence to start with a_1 = k, where k is any positive integer, and the same recursion formula applies: for n ≥ 2, a_n = S(a_(n-1)). The question notes that for many choices of k, the sequence a_n will eventually reach and remain at 1, but this is not always the case. It asks to find, with an explanation, two specific…arrow_forwardQUESTION 7 1) Write a maxConsecutiveDiff function which returns the maximum difference between any two consecutive values in the array. Consecutive values are next to each other in an array (+/- 1 index) This function should accept all required data as parameters. Examples If the array is 17 15 16 11 14 the maximum consecutive difference is 5 which is the difference between 16 and 11. If the array is 10 13 11 12 19 the maximum consecutive difference is 7 which is the difference between 12 and 19.arrow_forwardwrite this using java script arrow functions -thank you :)arrow_forward
- Programming language: Processing from Java Question attached as photo Topic: Use of Patial- Full Arraysarrow_forward1. Use the following array definition: int arr[10] = {1,9,7,0,8, 3,7,6,3,8 }; 2. Print all values stored in the array using the subscript operator (i.e.[ ]) 3. Print all values stored in the array using the dereference operator (i. e. *) 4. Print a table to show of the index, value, memory addressarrow_forwardWrite the function lastOf which searches the array a for the last occurance of any value contained in the array b. Returns the index if found. If not found, return -1. arrays.cpp 1 #include 2 int last0f(const int a[], int alen, const int b[], int bLen) { 3 4 5 6 7 8 }arrow_forward
- This program will display an array being passed to a function, show. The show function will display the elements of the given array. The main program controls the size and the inputs of an array. // function prototype void show(int [], int); int main(){ const int arraysize=5; int numbers[arraysize]={3,6,12,7,5}; show(numbers,arraysize); // (A) show(numbers,arraysize+1); return 0;} //function body void show(int nums[], int size){ for (int index=0;index<size; index++) cout<<nums[index]<<endl; } What is the output of this program? What is the output if modification (A) has been made?arrow_forward1- Write a user-defined function that accepts an array of integers. The function should generate the percentage each value in the array is of the total of all array values. Store the % value in another array. That array should also be declared as a formal parameter of the function. 2- In the main function, create a prompt that asks the user for inputs to the array. Ask the user to enter up to 20 values, and type -1 when done. (-1 is the sentinel value). It marks the end of the array. A user can put in any number of variables up to 20 (20 is the size of the array, it could be partially filled). 3- Display a table like the following example, showing each data value and what percentage each value is of the total of all array values. Do this by invoking the function in part 1.arrow_forwardC++ Dynamic Array Project Part 2arrow_forward
- C programarrow_forwardWhat does each line of code mean?arrow_forward/* (name header) #include // Function declaration int main() { int al[] int a2[] = { 7, 2, 10, 9 }; int a3[] = { 2, 10, 7, 2 }; int a4[] = { 2, 10 }; int a5[] = { 10, 2 }; int a6[] = { 2, 3 }; int a7[] = { 2, 2 }; int a8[] = { 2 }; int a9[] % { 5, 1, б, 1, 9, 9 }; int al0[] = { 7, 6, 8, 5 }; int all[] = { 7, 7, 6, 8, 5, 5, 6 }; int al2[] = { 10, 0 }; { 10, 3, 5, 6 }; %3D std::cout « ((difference (al, 4) « ((difference(a2, 4) <« ((difference (a3, 4) « ((difference(a4, 2) <« ((difference (a5, 2) <« ((difference (a6, 2) <« ((difference (a7, 2) <« ((difference(a8, 1) « ((difference (a9, 6) « ((difference(a10, 4) " <« ((difference(al1, 7) « ((difference (al2, 2) == 10) ? "OK\n" : "X\n"); << "al: << "a2: %3D 7)? "OK" : "X") << std::endl == 8) ? "OK\n" : "X\n") 8) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 1) ? "OK\n" : "X\n") == 0) ? "OK\n" : "X\n") 0) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 3) ? "OK\n" : "X\n") == 3) ? "OK\n" : "X\n") == << "a3: == << "a4: <<…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
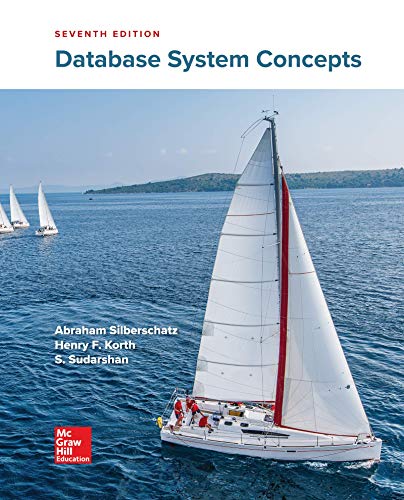
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
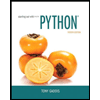
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
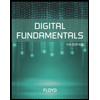
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
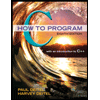
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
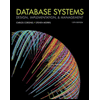
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
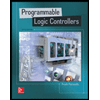
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education