Create a MIPS program that gets a set of numbers from the user, sorts them using selection sort, and displays the sorted numbers on the screen. You should create a subprogram to do the selection sort, sending it the length and address of the array. Pseudocode for selection sort: for (int i = 0; i < aLength-1; i++) { /* find the min element in the unsorted a[i .. aLength-1] */ /* assume the min is the first element */ int jMin = i; /* test against elements after i to find the smallest */ for (int j = i+1; j < aLength; j++) { /* if this element is less, then it is the new minimum */ if (a[j] < a[jMin]) { /* found new minimum; remember its index */ jMin = j; } } if (jMin != i) { swap(a[i], a[jMin]); } }
Create a MIPS
Pseudocode for selection sort:
for (int i = 0; i < aLength-1; i++) {
/* find the min element in the unsorted a[i .. aLength-1] */
/* assume the min is the first element */
int jMin = i;
/* test against elements after i to find the smallest */
for (int j = i+1; j < aLength; j++) {
/* if this element is less, then it is the new minimum */
if (a[j] < a[jMin]) {
/* found new minimum; remember its index */
jMin = j;
}
}
if (jMin != i)
{
swap(a[i], a[jMin]);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

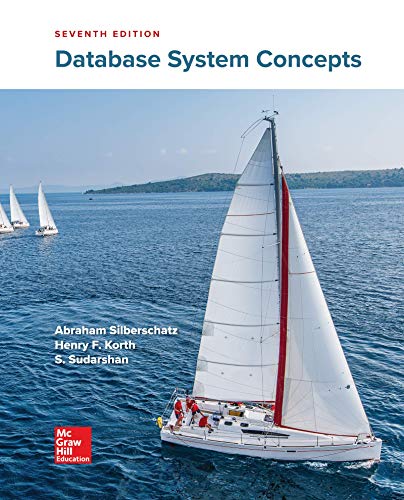
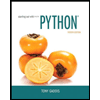
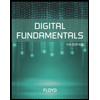
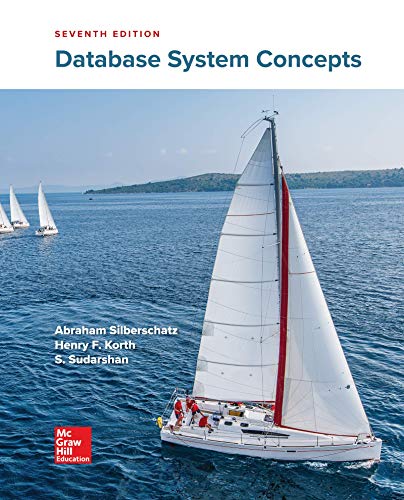
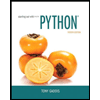
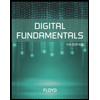
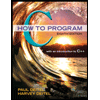
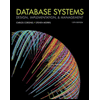
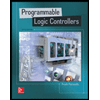