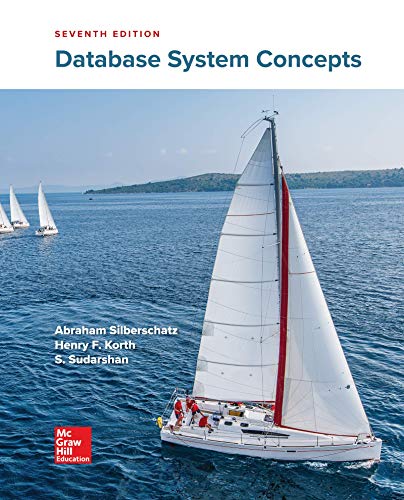
Create a class “Main” having main method to perform following tasks.
- Create two objects of Subject class having value “Math, 99.9” and “Physics, 98.9” respectively.
- Display the values of both objects
- Change the value of object from 99.9 to 89.9
- Compare both objects and display the values of the subject which have lowest score.
code:-
//Java program
package subject;
//class definition
public class Subject {
//instance variable
String subject;
double score;
//method to set the name and score
public void setSubject(String n1,double n2){
subject=n1;
score=n2;
if((n2<0.0) || (n2>100.0)){
System.out.println("Incorrect score");
score=0.0;
}
}
//method to set the score
public void setScore(double n){
score=n;
if((n<0.0) || (n>100.0)){
System.out.println("Incorrect score");
score=0.0;
}
}
//method to print the result
public void display(){
System.out.println("Name: "+subject+", Score: "+score);
}
//method to get the score
public double getScore(){
return score;
}
//method to compare teh score and retun true if the calling object has greater score
public boolean greaterThan(Subject obj){
return obj.score<score;
}
//driver method
public static void main(String args[]){
// Object of Student class is created
Subject obj = new Subject();
obj.setSubject("Math",99.9);
obj.display();
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- please quickly thanks ! use javaarrow_forwardIN JAVA PLEASE Child Class: Vegetable Write a child class called Vegetable. A vegetable is described by a name, the number of grams of sugar (as a whole number), the number of grams of sodium (as a whole number), and whether or not the vegetable is a starch. For the Vegetable class, write: the complete class header the instance data variables a constructor that sets the instance data variables based on parameters getters and setters; use instance data variables where appropriate a toString method that returns a text representation of a Vegetable object that includes all four characteristics of the vegetablearrow_forwardPlease include java doc commentarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
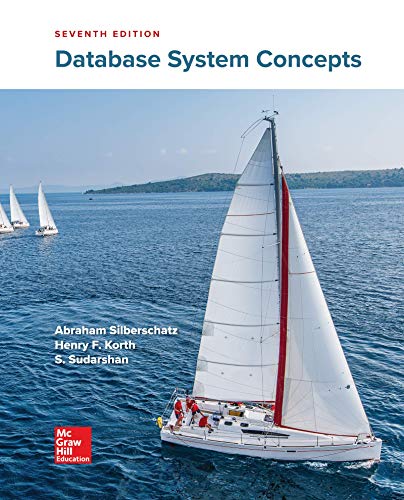
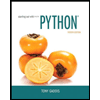
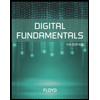
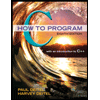
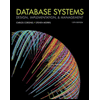
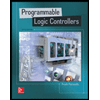