Creat a program which can process multiple groups. These groups are on the input separated by ’=\n’. Every group starts with a first line that contains the name of the group and the lines after contain the information about the students in the same way as the text file. The program needs to be written in Python, and needs to make use of functions and input parsers. With the input 1b Erik Eriksen__________4.3 4.9 6.7 Frans Franssen________5.8 6.9 8.0 = 2b Anne Adema____________6.5 5.5 4.5 Bea de Bruin__________6.7 7.2 7.7 Chris Cohen___________6.8 7.8 7.3 Dirk Dirksen__________1.0 5.0 7.7 The output should be: Report for group 1b Erik Eriksen has a final grade of 6.0 Frans Franssen has a final grade of 7.0 End of report Report for group 2b Anne Adema has a final grade of 6.0 Bea de Bruin has a final grade of 7.0 Chris Cohen has a final grade of 7.5 Dirk Dirksen has a final grade of 4.5 End of report
Creat a program which can process multiple groups.
These groups are on the input separated by ’=\n’. Every group starts with a first line that contains the name of the group and the lines after contain the information about the students in the same way as the text file.
The program needs to be written in Python, and needs to make use of functions and input parsers.
With the input
1b
Erik Eriksen__________4.3 4.9 6.7
Frans Franssen________5.8 6.9 8.0
=
2b
Anne Adema____________6.5 5.5 4.5
Bea de Bruin__________6.7 7.2 7.7
Chris Cohen___________6.8 7.8 7.3
Dirk Dirksen__________1.0 5.0 7.7
The output should be:
Report for group 1b
Erik Eriksen has a final grade of 6.0
Frans Franssen has a final grade of 7.0
End of report
Report for group 2b
Anne Adema has a final grade of 6.0
Bea de Bruin has a final grade of 7.0
Chris Cohen has a final grade of 7.5
Dirk Dirksen has a final grade of 4.5
End of report


Step by step
Solved in 5 steps with 3 images

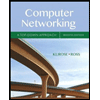
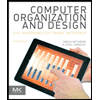
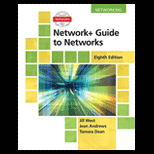
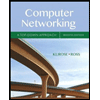
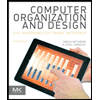
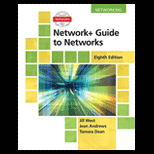
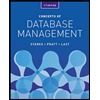
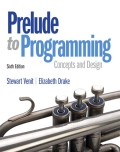
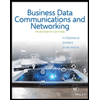