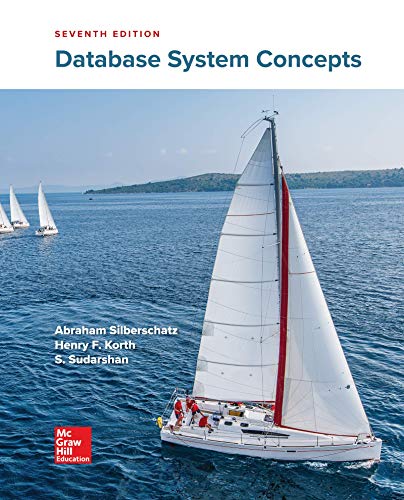
given code lab4
#include <stdio.h>
#include <stdlib.h>
/* typical C boolean set-up */
#define TRUE 1
#define FALSE 0
typedef struct StackStruct
{
int* darr; /* pointer to dynamic array */
int size; /* amount of space allocated */
int inUse; /* top of stack indicator
- counts how many values are on the stack */
} Stack;
void init (Stack* s)
{
s->size = 2;
s->darr = (int*) malloc ( sizeof (int) * s->size );
s->inUse = 0;
}
void push (Stack* s, int val)
{
/* QUESTION 7 */
/* check if enough space currently on stack and grow if needed */
/* add val onto stack */
s->darr[s->inUse] = val;
s->inUse = s->inUse + 1;
}
int isEmpty (Stack* s)
{
if ( s->inUse == 0)
return TRUE;
else
return FALSE;
}
int top (Stack* s)
{
return ( s->darr[s->inUse-1] );
}
/* QUESTION 9.1 */
void pop (Stack* s)
{
if (isEmpty(s) == FALSE)
s->inUse = s->inUse - 1;
}
void reset (Stack* s)
{
/* Question 10: how to make the stack empty? */
}
int main (int argc, char** argv)
{
Stack st1;
init (&st1);
push (&st1, 20);
push (&st1, 30);
push (&st1, 40);
push (&st1, 50);
while ( isEmpty(&st1) == FALSE)
{
int value = top (&st1);
printf ("The top value on the stack is %d\n", value);
/* QUESTION 9.2 */
pop (&st1);
}
printf ("The stack is now empty\n");
}
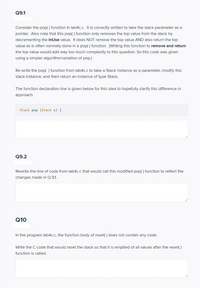

#include <stdio.h>
#include <stdlib.h>
/* typical C boolean set-up */
#define TRUE 1
#define FALSE 0
typedef struct StackStruct
{
int* darr; /* pointer to dynamic array */
int size; /* amount of space allocated */
int inUse; /* top of stack indicator
- counts how many values are on the stack */
} Stack;
void init (Stack* s)
{
s->size = 2;
s->darr = (int*) malloc ( sizeof (int) * s->size );
s->inUse = 0;
}
void push (Stack* s, int val)
{
/* QUESTION 7 */
/* check if enough space currently on stack and grow if needed */
if(s->inUse+1 >= s->size){ //If whole array is inn use i.e inUse +1 = size
s->size=s->size*2; //Increase the size to 2 times the current
//Using realloc to resize the size of array, it automatically retains previous elemnts
s->darr = (int *)realloc(s->darr,(s->size)*sizeof(int));
}
/* add val onto stack */
s->darr[s->inUse] = val;
s->inUse = s->inUse + 1;
}
int isEmpty (Stack* s)
{
if ( s->inUse == 0)
return TRUE;
else
return FALSE;
}
int top (Stack* s)
{
return ( s->darr[s->inUse-1] );
}
/* QUESTION 9 */
void pop (Stack* s)
{
s->inUse = s->inUse - 1;
}
void reset (Stack* s)
{
s->size=2;
free(s->darr);
s->darr = (int*) malloc ( sizeof (int) * s->size );
s->inUse = 0;
}
int main (int argc, char** argv)
{
Stack st1;
init (&st1);
push (&st1, 20);
push (&st1, 30);
push (&st1, 40);
push (&st1, 50);
while ( isEmpty(&st1) == FALSE)
{
int value = top (&st1);
printf ("The top value on the stack is %d\n", value);
/* QUESTION 10 */
pop (&st1);
}
printf ("The stack is now empty\n");
}
Step by stepSolved in 2 steps

- Q2: Stack stores elements in an ordered list and allows insertions and deletions at one end.The elements in this stack are stored in an array. If the array is full, the bottom item is dropped from the stack. In practice, this would be equivalent to overwriting that entry in the array. And if top method is called then it should return the element that was entered recently Please do it in C++ and use stack class(header file), not premade functionarrow_forwardAs recursion is implemented using a stack, an object of class Stack must be declared and initialized in the program to hold the class objects of every recursive call? a)true b) falsearrow_forwardWrite the report of this course that is the explanation of the algorithm in this program #include<iostream> // include necessary libraries #include<stack> // include necessary libraries #include<locale> // include necessary libraries #include <climits> // include necessary libraries using namespace std; int preced(char ch) { // defining precedence function if(ch == '+' || ch == '-') { // if condition return 1;}else if(ch == '*' || ch == '/') {return 2;}else {return 0;}} string inToPost(string infix ) { // defining push function stack<char> stk;stk.push('#'); string postfix = ""; string::iterator it; for(it = infix.begin(); it!=infix.end(); it++) { // for loop if(isalnum(char(*it))) // if condition postfix += *it;else if(*it == '(') // if condition stk.push('(');else if(*it == ')') { // if condition while(stk.top() != '#' && stk.top() != '(') {postfix += stk.top(); stk.pop(); // pop element }stk.pop(); }else {if(preced(*it) >…arrow_forward
- #include<bits/stdc++.h>using namespace std;#define MAX 1000 //Maximum size of stack class Stack{ int top;// to store top of stack public: int elements[MAX]; //Integer array to store elements Stack(){ //class constructor for initialization top=-1; } bool push(int x) { if(top>=(MAX-1)) //Condition for top when stack because full { cout<<"Stack is full\n"; return false; } else { ++top; //Increasing top value elements[top]=x; //storing it to element in to stack return true; }}int pop(){ if(top<0)// Condition for top when stack is empty { cout<<"stack is empty\n"; return INT_MIN; } else { int x=elements[top--];//poping out the element for stack by decreasing to element return x; }}int display(){if(top<0)//Condition for top when stack is empty { cout<<"Stack is…arrow_forwardData Structure Using C++ I need a function like this ::: float postfixEval(string postfix) {int a, b;stack<float> stk;string::iterator it;for(it=postfix.begin(); it!=postfix.end(); it++) {//read elements and perform postfix evaluationif(isOperator(*it) != -1) {a = stk.top();stk.pop();b = stk.top();stk.pop();stk.push(operation(a, b, *it));}else if(isOperand(*it) > 0) {stk.push(checkNumber(*it));}}return stk.top();} to evaluate postfix with more one digit in expression (multi digits) please just this function simple like the abovearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
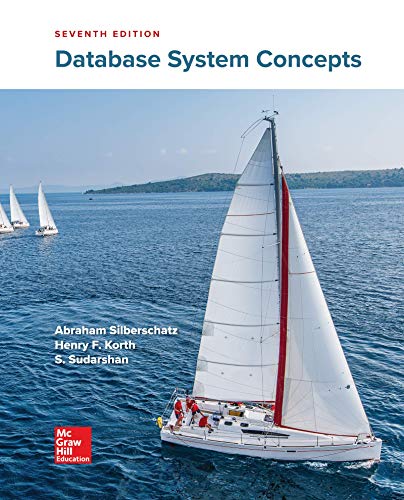
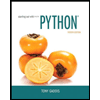
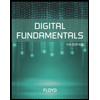
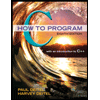
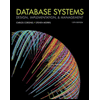
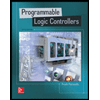