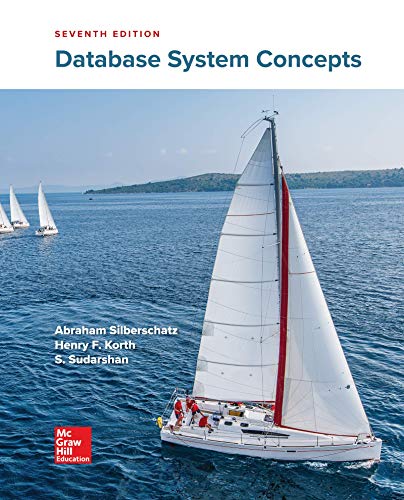
Write the report of this course that is the explanation of the
#include<iostream> // include necessary libraries
#include<stack> // include necessary libraries
#include<locale> // include necessary libraries
#include <climits> // include necessary libraries
using namespace std;
int preced(char ch) { // defining precedence function
if(ch == '+' || ch == '-') { // if condition
return 1;
}else if(ch == '*' || ch == '/') {
return 2;
}else {
return 0;
}
}
string inToPost(string infix ) { // defining push function
stack<char> stk;
stk.push('#');
string postfix = "";
string::iterator it;
for(it = infix.begin(); it!=infix.end(); it++) { // for loop
if(isalnum(char(*it))) // if condition
postfix += *it;
else if(*it == '(') // if condition
stk.push('(');
else if(*it == ')') { // if condition
while(stk.top() != '#' && stk.top() != '(') {
postfix += stk.top();
stk.pop(); // pop element
}
stk.pop();
}else {
if(preced(*it) > preced(stk.top())) // if condition
stk.push(*it);
else {
while(stk.top() != '#' && preced(*it) <= preced(stk.top())) { // while loop
postfix += stk.top();
stk.pop();
}
stk.push(*it);
}
}
}
while(stk.top() != '#') { // while loop
postfix += stk.top();
stk.pop();
}
return postfix;
}
float scanNum(char ch){
int value;
value = ch;
return float(value-'0');
}
int isOperator(char ch){ // defining function to check operator or not
if(ch == '+'|| ch == '-'|| ch == '*'|| ch == '/')
return 1;
return -1;
}
int isOperand(char ch){
if(ch >= '0' && ch <= '9')
return 1;
return -1;
}
float operation(int a, int b, char op){
if(op == '+')
return b+a;
else if(op == '-')
return b-a;
else if(op == '*')
return b*a;
else if(op == '/')
return b/a;
else
return INT_MIN;
}
float postfixEval(string postfix){
int a, b;
stack<float> stk;
string::iterator it;
for(it=postfix.begin(); it!=postfix.end(); it++){
if(isOperator(*it) != -1){
a = stk.top();
stk.pop();
b = stk.top();
stk.pop();
stk.push(operation(a, b, *it));
}else if(isOperand(*it) > 0){
stk.push(scanNum(*it));
}
}
return stk.top();
}
int main() { // main method
string infix;
Label:
cout<<"\n\nEnter the infix expression : "; // print message
getline(cin,infix);
int flag=0;
for(int i=0;i<infix.size();i++)
{
if(infix[i]>='a' && infix[i]<='z'|| infix[i]>='A'&& infix[i]<='Z')
{
printf("Invalid String!! Your string should have '*','/','+','-',','(',')' and integers.\nPlease re-enter the string..");
goto Label;
}
if(infix[i]>=32 && infix[i]<=39 || infix[i]==44 || infix[i]==46 || infix[i]>=58 && infix[i]<=64 || infix[i]>=91 && infix[i]<=96 )
{
printf("Invalid String!! Your string should have '*','/','+','-',','(',')' and integers.\nPlease re-enter the expression..");
goto Label;
}
if(infix[i]=='*' || infix[i]=='/' || infix[i]=='+' || infix[i]=='-' || infix[i]=='(' || infix[i]==')')
{
flag++;
}
}
if(flag!=0)
{
string postfix;
postfix=inToPost(infix);
cout << "Postfix Form Is : " << inToPost(infix) << endl;
cout << "Postfix evaluation : "<<postfixEval(postfix)<<endl;
}
else
{
printf("Invalid String!! Your string should only have '*','/','+','-',','(',')' and integers.\nPlease re-enter the string.");
goto Label;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- c++arrow_forwardThe destination of a function's return value may be represented as a sequence of instructions. When making modifications to the stack, keep in mind that they must not prevent the method from returning to its caller.arrow_forwardLanguage/Type: C++ binary trees pointers recursion Write a function named hasPath that interacts with a tree of BinaryTreeNode structures representing an unordered binary tree. The function accepts three parameters: a pointer to the root of the tree, and two integers start and end, and returns true if a path can be found in the tree from start down to end. In other words, both start and end must be element data values that are found in the tree, and end must be below start, in one of start's subtrees; otherwise the function returns false. If start and end are the same, you are simply checking whether a single node exists in the tree with that data value. If the tree is empty, your function should return false. For example, suppose a BinaryTreeNode pointer named tree points to the root of a tree storing the following elements. The table below shows the results of several various calls to your function: 67 88 52 1 21 16 99 45 Call Result Reason hasPath(tree, 67, 99) true path exists…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
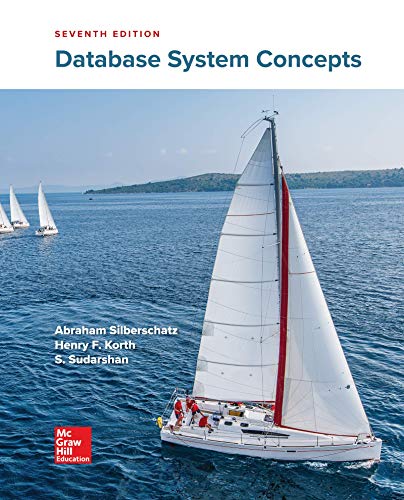
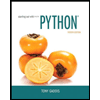
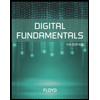
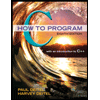
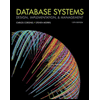
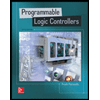