Consider the following version of insertion sort: Algorithm InsertSort2 (A[0...n − 1]) for i ← 1 to n − 1 do j ← i − 1 while j ≥ 0 and A[j] > A[j + 1] do swap(A[j], A[j + 1]) j ← j − 1 What is its time efficiency? How does it compare to the version given in the text? Apply insertion sort to sort the list E, X, A, M, P, L, E in alphabetical order. Consider the following algorithm to check connectivity of a graph defined by its adjacency matrix. Algorithm Connected(A[0...n - 1, 0...n - 1])// Input: Adjacency matrix A[0...n - 1, 0...n - 1] of an undirected graph G// Output: 1 (true) if G is connected and 0 (false) if it is notif n = 1 return 1 // one-vertex graph is connected by definitionelseif not Connected(A[0...n - 2, 0...n - 2]) return 0else for j ← 0 to n - 2 doif A[n - 1, j] return 1return 0 Does this algorithm work correctly for every undirected graph with n > 0 vertices? If you answer "yes," indicate the algorithm’s efficiency class in the worst case; if you answer "no," explain why. a. What sentinel should be put before the first element of an array beingsorted in order to avoid checking the in-bound condition j ≥ 0 on eachiteration of the inner loop of insertion sort?b. Will the version with the sentinel be in the same efficiency class asthe original version? a. Prove that the topological sorting problem has a solution for a digraphif and only if it is a dag.b. For a digraph with n vertices, what is the largest number of distinctsolutions the topological sorting problem can have? a. What is the time efficiency of the DFS-based algorithm for topologicalsorting?b. How can one modify the DFS-based algorithm to avoid reversing thevertex ordering generated by DFS?
Consider the following version of insertion sort:
for i ← 1 to n − 1 do
j ← i − 1
while j ≥ 0 and A[j] > A[j + 1] do
swap(A[j], A[j + 1])
j ← j − 1
What is its time efficiency? How does it compare to the version given in the text?
Apply insertion sort to sort the list E, X, A, M, P, L, E in alphabetical order.
Consider the following algorithm to check connectivity of a graph defined by its adjacency matrix.
Algorithm Connected(A[0...n - 1, 0...n - 1])
// Input: Adjacency matrix A[0...n - 1, 0...n - 1] of an undirected graph G
// Output: 1 (true) if G is connected and 0 (false) if it is not
if n = 1 return 1 // one-vertex graph is connected by definition
else
if not Connected(A[0...n - 2, 0...n - 2]) return 0
else for j ← 0 to n - 2 do
if A[n - 1, j] return 1
return 0
Does this algorithm work correctly for every undirected graph with n > 0 vertices? If you answer "yes," indicate the algorithm’s efficiency class in the worst case; if you answer "no," explain why.
a. What sentinel should be put before the first element of an array being
sorted in order to avoid checking the in-bound condition j ≥ 0 on each
iteration of the inner loop of insertion sort?
b. Will the version with the sentinel be in the same efficiency class as
the original version?
a. Prove that the topological sorting problem has a solution for a digraph
if and only if it is a dag.
b. For a digraph with n vertices, what is the largest number of distinct
solutions the topological sorting problem can have?
a. What is the time efficiency of the DFS-based algorithm for topological
sorting?
b. How can one modify the DFS-based algorithm to avoid reversing the
vertex ordering generated by DFS?
Unlock instant AI solutions
Tap the button
to generate a solution
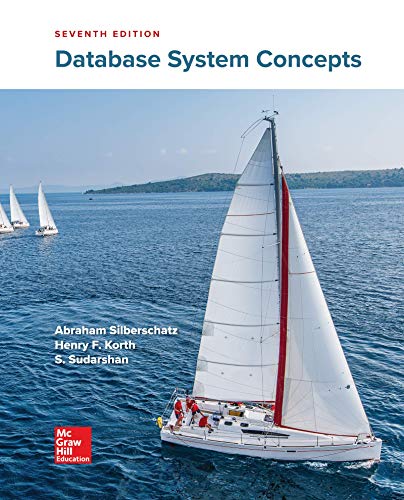
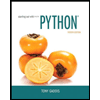
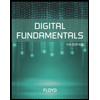
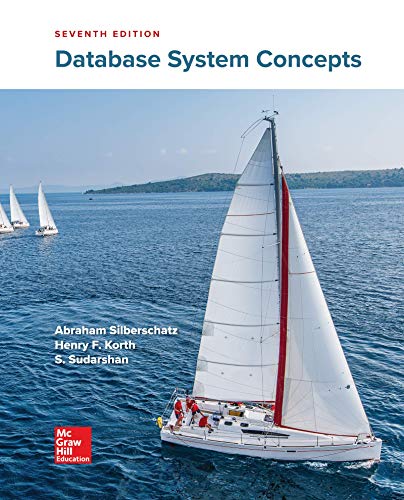
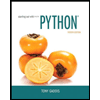
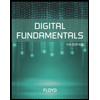
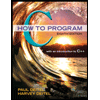
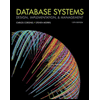
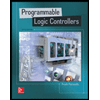