Consider the following class and member function implementation. Derive a class from GradedActivity named Presentation which has two member variable ints, content and delivery which determine a Presentation’s score. Implement the following member functions for Presentation: - a default constructor which sets both member variables and the score to zero - a void member function named set which takes two int parameters c and d, sets content to c and delivery to d, and sets the score to c+d. This function may assume that 0 <= c+d <=100, you don't have to check it. Additionally, provide a simple main() function which creates an object of class Presentation, uses set() to set the object’s member data with some example values, and calls getLetterGrade() to print out the letter grade.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Consider the following class and member function implementation. Derive a class from GradedActivity named Presentation which has two member variable ints, content and delivery which determine a Presentation’s score. Implement the following member functions for Presentation:
- a default constructor which sets both member variables and the score to zero
- a void member function named set which takes two int parameters c and d, sets content to c and delivery to d, and sets the score to c+d. This function may assume that 0 <= c+d <=100, you don't have to check it.
Additionally, provide a simple main() function which creates an object of class Presentation, uses set() to set the object’s member data with some example values, and calls getLetterGrade() to print out the letter grade.
class GradedActivity { private: int score; public: GradedActivity() { score = 0.0; } GradedActivity(int s) { score = s; } void setScore(int s) { score = s; } int getScore() { return score; } char getLetterGrade(); }; |
char GradedActivity::getLetterGrade() { char letterGrade; if (score > 89) letterGrade = 'A'; else if (score > 79) letterGrade = 'B'; else if (score > 69) letterGrade = 'C'; else if (score > 59) letterGrade = 'D'; else letterGrade = 'F'; return letterGrade; } |

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

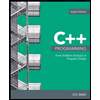
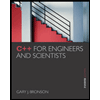
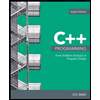
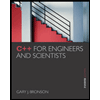