Complete/Modify the code satisfying the following: (put comments pls) in C++, pls put COMMENTS in the code 1. Create a 10x10 matrix using rand function to fill the said matrix with random numbers 2. Print all the numbers in matrix form 3. Ask the user the following options OPTIONS: A. Total sum, average, median per row B. Total sum, average, median per column C. Display the same matrix but all ODD numbers are replaced with "-" (minus sign) D. Display the same matrix but all EVEn numbers are replaced with "+" (plus sign E. Display the same matrix but all PRIME numbers are replaced with "*" (asterisksign) F. Display the whole matrix with every row in ascending order G. Display the whole matrix with every row in descending order H. Display the whole matrix with every column in ascending order I. Display the whole matrix with every cloumn in descending order J. Generate new set of random numbers Q. Close the program After asking what option to display, Display again the previous numbers entered and the same options. Here's the code: #include #include #include ascendrow(int a[10]) { int cntr1,cntr2,temp,b[10]; for(cntr1=0;cntr1<10;cntr1++) { b[cntr1] = a[cntr1]; } for(cntr1=0;cntr1<10;cntr1++) { for(cntr2=cntr1;cntr2<10;cntr2++) { if(b[cntr1]>b[cntr2]) { temp = b[cntr1]; b[cntr1] = b[cntr2]; b[cntr2] = temp; } } } for(cntr1=0;cntr1<10;cntr1++) { printf("\t%d",b[cntr1]); } } int matsum(int a[10]) { int totalsum = 0,cntr1; for(cntr1=0;cntr1<10;cntr1++) { totalsum = totalsum + a[cntr1]; } return totalsum; } main() { char rep; rep = 'y'; int cntr=0,temp; char option; int x[10],y[10]; for(cntr=0;cntr<10;cntr++) { x[cntr] = rand() % 100; } while(rep=='y'||rep =='Y') { printf("\n\nGenerated nos are: \n"); for(cntr=0;cntr<10;cntr++) { printf("\t%d",x[cntr]); } printf("\n\na: Display the total sum of the array.\n"); printf("b: Display ascending order.\n"); printf("q: Quit program.\n"); printf("--> "); scanf("%s",&option); switch(option) { case 'a': temp = matsum(x); printf("The total sum of the array is %d.\n",temp); break; case 'b': printf("Ascending order\n"); ascendrow(x); break; case 'q': rep = 'q'; printf("THANKS! BYE! :D"); break; default: printf("Please choose from options a-b.\n"); break; } } }
Complete/Modify the code satisfying the following: (put comments pls)
in C++, pls put COMMENTS in the code
1. Create a 10x10 matrix using rand function to fill the said matrix with random numbers
2. Print all the numbers in matrix form
3. Ask the user the following options
OPTIONS:
A. Total sum, average, median per row
B. Total sum, average, median per column
C. Display the same matrix but all ODD numbers are replaced with "-" (minus sign)
D. Display the same matrix but all EVEn numbers are replaced with "+" (plus sign
E. Display the same matrix but all PRIME numbers are replaced with "*" (asterisksign)
F. Display the whole matrix with every row in ascending order
G. Display the whole matrix with every row in descending order
H. Display the whole matrix with every column in ascending order
I. Display the whole matrix with every cloumn in descending order
J. Generate new set of random numbers
Q. Close the program
After asking what option to display, Display again the previous numbers entered and the same options.
Here's the code:
#include<stdio.h>
#include<math.h>
#include<stdlib.h>
ascendrow(int a[10])
{
int cntr1,cntr2,temp,b[10];
for(cntr1=0;cntr1<10;cntr1++)
{
b[cntr1] = a[cntr1];
}
for(cntr1=0;cntr1<10;cntr1++)
{
for(cntr2=cntr1;cntr2<10;cntr2++)
{
if(b[cntr1]>b[cntr2])
{
temp = b[cntr1];
b[cntr1] = b[cntr2];
b[cntr2] = temp;
}
}
}
for(cntr1=0;cntr1<10;cntr1++)
{
printf("\t%d",b[cntr1]);
}
}
int matsum(int a[10])
{
int totalsum = 0,cntr1;
for(cntr1=0;cntr1<10;cntr1++)
{
totalsum = totalsum + a[cntr1];
}
return totalsum;
}
main()
{
char rep;
rep = 'y';
int cntr=0,temp;
char option;
int x[10],y[10];
for(cntr=0;cntr<10;cntr++)
{
x[cntr] = rand() % 100;
}
while(rep=='y'||rep =='Y')
{
printf("\n\nGenerated nos are: \n");
for(cntr=0;cntr<10;cntr++)
{
printf("\t%d",x[cntr]);
}
printf("\n\na: Display the total sum of the array.\n");
printf("b: Display ascending order.\n");
printf("q: Quit program.\n");
printf("--> ");
scanf("%s",&option);
switch(option)
{
case 'a':
temp = matsum(x);
printf("The total sum of the array is %d.\n",temp);
break;
case 'b':
printf("Ascending order\n");
ascendrow(x);
break;
case 'q':
rep = 'q';
printf("THANKS! BYE! :D");
break;
default:
printf("Please choose from options a-b.\n");
break;
}
}
}

Step by step
Solved in 2 steps with 1 images

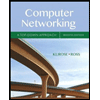
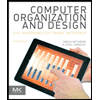
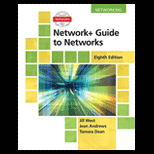
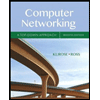
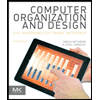
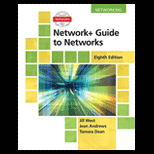
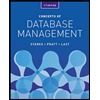
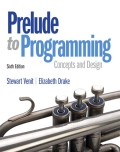
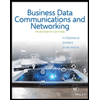