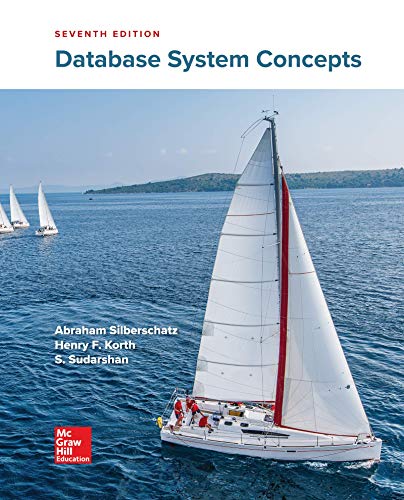
I need help with this question and I keep getting this error line -3: error: illegal character: '\'
X438: LinkedList - Create a LinkedList
Complete a custom singly-linked LinkedList to hold generic objects. The list will use nodes called LinearNodes, which have methods getNext, setNext, getElem, and setElem. It includes methods for adding an element to a specific index or simply to the end, removing or replacing elements at specific indexes, peeking at an element without removing it, and returning the size of the list. NEEDS TESTING
Code:
private class LinkedList<E> {
private int size;
private LinearNode<E> first;
private LinearNode<E> last;
public LinkedList() {
}
public void add(E elem) {
}
public void add(E elem, int index) {
}
public E remove(int index) {
}
public E peek(int index) {
}
public E replace(int index, E elem) {
}
public int size() {
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Based on the previous questions, create a Queue class that uses the LinkedList for its data storage. Create the __init__, isEmpty, insert, remove, and size methods. Assume that LinkedList class has the add, removeFront and size methods defined.arrow_forwardlinked_list_stack_shopping_list_manager.py This is a file that includes the class of linked list based shopping list manager. This class contains such methods as init, insert_item, is_list_empty, print_item_recursive_from_top, print_items_from_top, print_item_recursive_from_bottom, print_items_from_bottom, getLastItem, removeLastItem. In addition, this class requires the inner class to hold onto data as a linked list based on Stack. DO NOT use standard python library, such as deque from the collections module. Please keep in mind the following notes for each method during implementation: Init(): initializes linked list based on Stack object to be used throughout object life. insert_item(item): inserts item at the front of the linked list based on Stack. Parameters: item name. is_list_empty (): checks if the current Stack object is empty (ex. check if head is None). print_item_recursive_from_top(currentNode): a helper method to print linked list based on Stack item recursively. Note:…arrow_forwardQuestion Completion Status: QUESTION 1 Write a Java Program to do the following: Create an LinkedList object and store 5 different Float objects. Using a Listlterator do the following: Compute the average of all numbers. Attach File Browse My Computer lck Sape and Submit to save and submit Clhck Save Al Answers to save all answers.arrow_forward
- Start this lab with the code listed below. The LinkedList class defines the rudiments of the code needed to build a linked list of Node objects. You will first complete the code for its addFirst method. This method is passed an object that is to be added to the beginning of the list. Write code that links the passed object to the list by completing the following tasks in order:1. Create a new Node object.2. Make the data variable in the new Node object reference the object that was passed to addFirst.3. Make the next variable in the new Node object reference the object that is currently referenced in variable first.4. Make variable first reference the new Node.Test your code by running the main method in the LinkedListRunner class below. Explain, step by step, why each of the above operations is necessary. Why are the string objects in the reverse order from the way they were added? public class LinkedList{ private Node first; public LinkedList() { first = null; } public Object…arrow_forwardMake a data structure that enables the access and delete actions. If the item isn't already on the data structure, the access action adds it. The object with the least recent access is deleted and returned by the remove operation. Hint: Maintain references to the first and last nodes as well as the items' order of access in a doubly linked list. Use a symbol table where the keys represent the items and the values the links in the list. Remove the element from the linked list after accessing it, then reintroduce it at the start. When you eliminate an element, do both the symbol table and end deletions.arrow_forwardSolution plz..arrow_forward
- You are going to implement a program that creates an unsorted list by using a linked list implemented by yourself. NOT allowed to use LinkedList class or any other classes that offers list functions. It is REQUIRED to use an ItemType class and a NodeType struct to solve this homework. The “data.txt” file has three lines of data 100, 110, 120, 130, 140, 150, 160 100, 130, 160 1@0, 2@3, 3@END You need to 1. create an empty unsorted list 2. add the numbers from the first line to list using putItem() function. Then print all the current keys to command line in one line using printAll(). 3. delete the numbers given by the second line in the list by using deleteItem() function. Then print all the current keys to command line in one line using printAll().. 4. putItem () the numbers in the third line of the data file to the corresponding location in the list. For example, 1@0 means adding number 1 at position 0 of the list. Then print all the current keys to command line in one…arrow_forwardget_avg_ratings() takes a 2-D list similar to ratings_db as the parameter and returns a dictionary, where each {key: value} of this dictionary is {a valid movie id: the average rating this movie received by the reviewers}. I will refer to this dictionary as ratings.>>> ratings = get_avg_ratings(ratings_db)>>>> display_dict(ratings)1: 3.922: 3.433: 3.264: 2.365: 3.076: 3.957: 3.198: 2.889: 3.1210: 3.511: 3.6712: 2.4213: 3.1214: 3.83 15: 3.016: 3.9317: 3.7818: 3.719: 2.7320: 2.5 userId,movieId,rating,timestamp1,1,4,9649827035,1,4,8474349627,1,4.5,110663594615,1,2.5,151057797017,1,4.5,130569648318,1,3.5,145520981619,1,4,96570563721,1,3.5,140761887827,1,3,96268526231,1,5,85046661632,1,3,85673611933,1,3,93964744440,1,5,83205895943,1,5,84899398344,1,3,86925186045,1,4,95117018246,1,5,83478790650,1,3,151423811654,1,3,83024733057,1,5,965796031arrow_forwardLinked List, create your own code. (Do not use the build in function or classes of Java or from the textbook). Create a LinkedList class: Call the class MyLinkedList, (hint) Create a second class called Node.java and use it, remember in the class I put the Node class inside the LinkedList Class, but you should do it outside. This class should haveo Variables you may need for a Node,o (optional) Constructor Your linked list is of an int type. (you may do it as General type as <E>) For this Linked List you need to have the following methods: add, addAfter, remove, size, contain, toString, compare, addInOrder. This is just a suggestion, if you use Generic type, you must modify this Write a main function or Main class to test all the methods,o Create a 2 linked list and test all your methods. (Including the compare)arrow_forward
- The linked list is Select one: a. A link that points to another link b. a pointer that points to node structure c. Array of nodes structure d. array of pointers each one point to node structurearrow_forwardTask 13: The function below should insert a value as the head of a given UNORDERED linked list. Be careful to first search for the given value. The value is inserted only if it does not exist in the list. If the value exists, the function does nothing. Use Linked list implementation of Task 1 to make this function run. Write down the code of insert function run the code, take snap shot and paste it in your document. struct Node{ int data; Node* next; }; The function prototype is given. Implement the function. void insert(Node* &head, int value);arrow_forward###__write the proper code in C language.__##.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
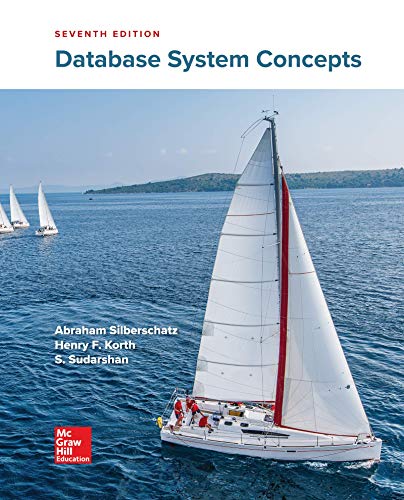
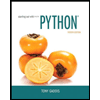
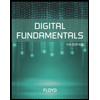
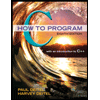
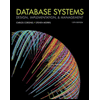
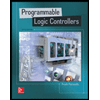