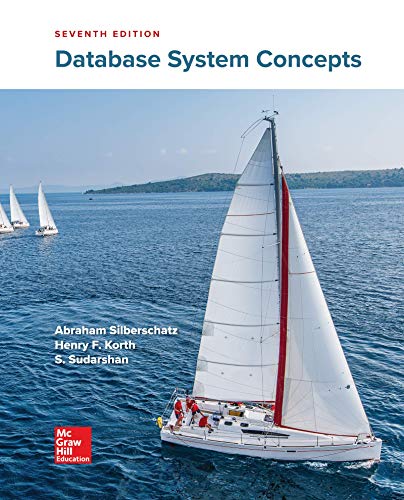
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
thumb_up100%
dict_graph = {}
# Read the data.txt file
with open('data.txt', 'r') as f:
for l in f:
city_a, city_b, p_cost = l.split()
if city_a not in dict_graph:
dict_graph[city_a] = {}
dict_graph[city_a][city_b] = int(p_cost)
if city_b not in dict_graph:
dict_graph[city_b] = {}
dict_graph[city_b][city_a] = int(p_cost)
# Breadth First Search Method
def BreadthFirstSearch(graph, src, dst):
q = [(src, [src], 0)]
visited = {src}
while q:
(node, path, cost) = q.pop(0)
for temp in graph[node].keys():
if temp == dst:
return path + [temp], cost + graph[node][temp]
else:
if temp not in visited:
visited.add(temp)
q.append((temp, path + [temp], cost + graph[node][temp]))
# Depth First Search Method
def DepthFirstSearch(graph, src, dst):
stack = [(src, [src], 0)]
visited = {src}
while stack:
(node, path, cost) = stack.pop()
for temp in graph[node].keys():
if temp == dst:
return path + [temp], cost + graph[node][temp]
else:
if temp not in visited:
visited.add(temp)
stack.append((temp, path + [temp], cost + graph[node][temp]))
# Iterative Deepening Search Method
def IterativeDeepening(graph, src, dst):
level = 0
count = 0
stack = [(src, [src], 0)]
visited = {src}
while True:
level += 1
while stack:
if count <= level:
count = 0
(node, path, cost) = stack.pop()
for temp in graph[node].keys():
if temp == dst:
return path + [temp], cost + graph[node][temp]
else:
if temp not in visited:
visited.add(temp)
count += 1
stack.append((temp, path + [temp], cost + graph[node][temp]))
else:
q = stack
visited_bfs = {src}
while q:
(node, path, cost) = q.pop(0)
for temp in graph[node].keys():
if temp == dst:
return path + [temp], cost + graph[node][temp]
else:
if temp not in visited_bfs:
visited_bfs.add(temp)
q.append((temp, path + [temp], cost + graph[node][temp]))
break
n = 1
print (dict_graph)
print ("------------------------------------------------")
while n == 1:
x = input("enter the type of search you want to do \n 1.BFS 2.DFS 3.ID \n ")
if x == 1:
src = raw_input("Enter the source: ")
dst = raw_input("Enter the Destination: ")
while src not in dict_graph or dst not in dict_graph:
print ("No such city name")
src = raw_input("Enter the correct source (case_sensitive):\n")
dst = raw_input("Enter the correct destination(case_sensitive):\n ")
print ("for BFS")
print (BreadthFirstSearch(dict_graph, src, dst))
elif x == 2:
src = raw_input("Enter the source: ")
dst = raw_input("Enter the Destination: ")
while src not in dict_graph or dst not in dict_graph:
print ("No such city name")
src = raw_input("Enter the correct source (case_sensitive):\n")
dst = raw_input("Enter the correct destination(case_sensitive):\n ")
print ("for DFS")
print (DepthFirstSearch(dict_graph, src, dst))
elif x == 3:
src = raw_input("Enter the source:")
dst = raw_input("Enter the Destination: ")
while src not in dict_graph or dst not in dict_graph:
print ("No such city name")
src = raw_input("Enter the correct source (case_sensitive):\n")
dst = raw_input("Enter the correct destination(case_sensitive):\n")
print ("for ID")
print (IterativeDeepening(dict_graph, src, dst))
#n = input("enter 1 if you wish to continue:\n")
Check these codes and fix the errors
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Sum of two squares def sum_of_two_squares(n): Some positive integers can be expressed as a sum of two squares of some positive integers greater than zero. For example, 74 = 49 + 25 = 7^2 + 5^2. This function should find and return a tuple of two positive integers whose squares together add up to n, or return None if the parameter n cannot be broken into a sum of two squares.To facilitate the automated testing, the returned tuple must present the larger of its two numbers first. Furthermore, if some integer can be broken down to a sum of squares in several ways, return the breakdown that maximizes the larger number. For example, the number 85 allows two such representations 7^2 + 6^2 and 9^2 + 2^2 , of which this function must return (9, 2).The technique of two approaching indices that start from the beginning and end of a sequence, respectively, and approach each other until they meet somewhere is directly applicable to this problem. In this problem, these indices crawl towards each…arrow_forwardPython I have a class QueryBuilder: def QueryBuilder(Data_Base, Query_Type, Query_Tuple):''' Build Query_String '''if Query_Type == "version":query_string = "SELECT sqlite_version()"elif Query_Type == "delete":query_string = "DELETE FROM {0} WHERE id = ?".format(Data_Base)elif Query_Type == "select":query_string = "SELECT * FROM {0} WHERE name = ?".format(Data_Base)elif Query_Type == "insert":query_string = "INSERT INTO {0} (id, name, photo, html) VALUES (?, ?, ?, ?)".format(Data_Base)elif Query_Type == "table":query_string = '''CREATE TABLE Database (id INTEGER PRIMARY KEY,name TEXT NOT NULL,photo text NOT NULL UNIQUE,html text NOT NULL UNIQUE)'''.format(Data_Base)else:raise ValueError("Invalid query type")return query_string Please use it to write more code below: Purpose: Create and use a Database Data Files: Use BS4, Regular Expressions or Pandas to read in the two data files for this assignment: Co2.html: <TABLE summary="csv2html program…arrow_forwardmain.cc file #include <iostream>#include <memory> #include "customer.h" int main() { // Creates a line of customers with Adele at the front. // LinkedList diagram: // Adele -> Kehlani -> Giveon -> Drake -> Ruel std::shared_ptr<Customer> ruel = std::make_shared<Customer>("Ruel", 5, nullptr); std::shared_ptr<Customer> drake = std::make_shared<Customer>("Drake", 8, ruel); std::shared_ptr<Customer> giveon = std::make_shared<Customer>("Giveon", 2, drake); std::shared_ptr<Customer> kehlani = std::make_shared<Customer>("Kehlani", 15, giveon); std::shared_ptr<Customer> adele = std::make_shared<Customer>("Adele", 4, kehlani); std::cout << "Total customers waiting: "; // =================== YOUR CODE HERE =================== // 1. Print out the total number of customers waiting // in line by invoking TotalCustomersInLine. //…arrow_forward
- c++ programming language. Part c I got a respond from you guys and you said it's a writing question but it's not, it a c++ programming question that i need help with please. I need help with part c please.arrow_forward#ifndef LLCP_INT_H#define LLCP_INT_H #include <iostream> struct Node{ int data; Node *link;};void DelOddCopEven(Node*& headPtr);int FindListLength(Node* headPtr);bool IsSortedUp(Node* headPtr);void InsertAsHead(Node*& headPtr, int value);void InsertAsTail(Node*& headPtr, int value);void InsertSortedUp(Node*& headPtr, int value);bool DelFirstTargetNode(Node*& headPtr, int target);bool DelNodeBefore1stMatch(Node*& headPtr, int target);void ShowAll(std::ostream& outs, Node* headPtr);void FindMinMax(Node* headPtr, int& minValue, int& maxValue);double FindAverage(Node* headPtr);void ListClear(Node*& headPtr, int noMsg = 0); // prototype of DelOddCopEven of Assignment 5 Part 1 #endifarrow_forwardmain.cc file #include <iostream>#include <memory> #include "train.h" int main() { // Creates a train, with the locomotive at the front of the train. // LinkedList diagram: // Locomotive -> First Class -> Business Class -> Cafe Car -> Carriage 1 -> // Carriage 2 std::shared_ptr<Train> carriage2 = std::make_shared<Train>(100, 100, nullptr); std::shared_ptr<Train> carriage1 = std::make_shared<Train>(220, 220, carriage2); std::shared_ptr<Train> cafe_car = std::make_shared<Train>(250, 250, carriage1); std::shared_ptr<Train> business_class = std::make_shared<Train>(50, 50, cafe_car); std::shared_ptr<Train> first_class = std::make_shared<Train>(20, 20, business_class); std::shared_ptr<Train> locomotive = std::make_shared<Train>(1, 1, first_class); std::cout << "Total passengers in the train: "; // =================== YOUR CODE HERE…arrow_forward
- What are the values of the variable number and the vector review after the following code segment is executed? vector<int> review(10,-1); int number; number = review[0]; review[1] = 150; review[2] = 350; review[3] = 450; review.push_back(550); review.push_back(650); number += review[review.size()-1]; review.pop_back();arrow_forwardBar Graph, v 1.0 Purpose. The purpose of this lab is to produce a bar graph showing the population growth of a city called Prairieville, California. The bar graph should show the population growth of the city in 20 year increments for the last 100 years. The bar graph should be printed using a loop. The loop can be either a while loop or a for loop. Write a program called cityPopulation Graph.cpp to produce the bar graph. Requirements. 1. Use these population values with their corresponding years: 1922, 2000 people 1942, 5000 people 1962, 6000 people 1982, 9000 people 2002, 14,000 people 2012, 15,000 people 2022, 17,000 people 2. For each year, the program should display the year with a bar consisting of one asterisk for each 1000 people. 3. Each time the loop is performed (that is, each time the loop iterates) one of the year values is displayed with asterisks next to it. That is: 1st time loop is performed/iterates the year 1922 and the correct number of asterisks should be…arrow_forwardGiven the following C code: struct Node int data; struct Node struct Node next; prev; struct Node head; void funx ( ) if (head==NULL) return; struct Node current=head; while (current->next!=NULL) current=current-->next; while (current!=NULL) printf("&d ", current->data; current=current->prev; What is the functionality of this function? a) Print the contents of linked list b) Print the contents of linked list in reverse order c) Nonearrow_forward
- Write a loop that finds the smallest value. Print it and the index code format: #include <iostream>using namespace std; #include <cstdlib> // required for rand() int main(){// Put Part2 code here // Declare smallestFoundSoFar// Q: what should be its initial value?int indexOfSmallest = -1;// sign that it is not initialized Part 2 code #include <iostream> using namespace std; // part 1 code#include <cstdlib> int main() { srand(17); const int ARRAYSIZE = 20; // size for the array int RandArray[ARRAYSIZE]; // array declared int i; // to iterate the loop // this loop will store thei random number in the array for (i = 0; i < ARRAYSIZE; i++) RandArray[i] = rand() % 100; // this loop will print the array for (i = 0; i < ARRAYSIZE; i++) cout <<"randArray["<< i <<"]=" << RandArray[i] << endl; int largestFoundSoFar=-1; // anything is larger than this! int indexOfLargest = -1; // sign that it is not initialized for (i = 0; i…arrow_forward#include <iostream>#include <cstdlib>using namespace std; class IntNode {public: IntNode(int dataInit = 0, IntNode* nextLoc = nullptr); void InsertAfter(IntNode* nodeLoc); IntNode* GetNext(); void PrintNodeData(); int GetDataVal();private: int dataVal; IntNode* nextNodePtr;}; // ConstructorIntNode::IntNode(int dataInit, IntNode* nextLoc) { this->dataVal = dataInit; this->nextNodePtr = nextLoc;} /* Insert node after this node. * Before: this -- next * After: this -- node -- next */void IntNode::InsertAfter(IntNode* nodeLoc) { IntNode* tmpNext = nullptr; tmpNext = this->nextNodePtr; // Remember next this->nextNodePtr = nodeLoc; // this -- node -- ? nodeLoc->nextNodePtr = tmpNext; // this -- node -- next} // Print dataValvoid IntNode::PrintNodeData() { cout << this->dataVal << endl;} // Grab location pointed by nextNodePtrIntNode* IntNode::GetNext() { return this->nextNodePtr;} int IntNode::GetDataVal() {…arrow_forwardConcatenate Map This function will be given a single parameter known as the Map List. The Map List is a list of maps. Your job is to combine all the maps found in the map list into a single map and return it. There are two rules for addingvalues to the map. You must add key-value pairs to the map in the same order they are found in the Map List. If the key already exists, it cannot be overwritten. In other words, if two or more maps have the same key, the key to be added cannot be overwritten by the subsequent maps. Signature: public static HashMap<String, Integer> concatenateMap(ArrayList<HashMap<String, Integer>> mapList) Example: INPUT: [{b=55, t=20, f=26, n=87, o=93}, {s=95, f=9, n=11, o=71}, {f=89, n=82, o=29}]OUTPUT: {b=55, s=95, t=20, f=26, n=87, o=93} INPUT: [{v=2, f=80, z=43, k=90, n=43}, {d=41, f=98, y=39, n=83}, {d=12, v=61, y=44, n=30}]OUTPUT: {d=41, v=2, f=80, y=39, z=43, k=90, n=43} INPUT: [{p=79, b=10, g=28, h=21, z=62}, {p=5, g=87, h=38}, {p=29,…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
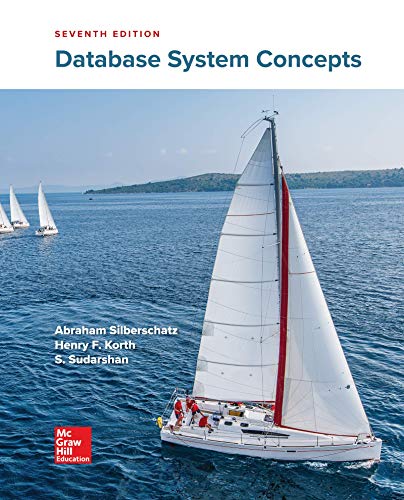
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
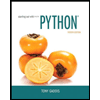
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
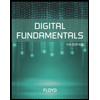
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
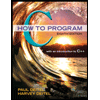
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
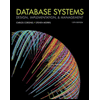
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
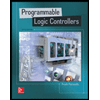
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education