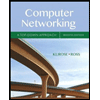
Code in C. Solve the code below.
write a function that receives a
int busca_bin (int vetor [ ], int n, int b)
{
#include <stdio.h>
int busca_bin(int vetor[] , int n , int a)
{
int s1 = 0;
int s2 = n-1;
int pass = 0;
int s3;
while(s1<s2)
{
s3 = (s1+s2)/2;
pass++;
if(vetor[s3]==a)
return pass;
else if(vetor[s3]>a)
s2 = s3 - 1;
else
s1 = s3 + 1;
}
return 0;
}
![Precheck only
Test
Input Expected Got
int vetor[]={1,2,3,4,5}, n=5, b, x; 3
1
1
scanf("%d", &b);
x=busca_bin(vetor,n,b);
printf("%d", x);
int vetor[]={1,2,3,4,5}, n=5, b, x; 5
scanf("%d",&b);
x=busca_bin(vetor,n,b);
printf("%d",x);
int vetor[]={1,2,3,4,5}, n=5, b, x; 6
scanf("%d", &b);
x=busca_bin(vetor,n,b);
printf("%d",x);
>
>
3.](https://content.bartleby.com/qna-images/question/705bcef8-c7ac-4483-b54f-9a358327c6e3/b3c310a6-2b40-406f-91d8-b31d40ddd72f/jp553y_thumbnail.png)
![For example:
Test
Input Result
int vetor[]={1,2,3,4,5}, n=5, b, x; 3
scanf("%d" , &b);
x=busca_bin(vetor,n,b);
printf("%d",x);
int vetor[]={1,2,3,4,5}, n=5, b, x; 5
3
scanf("%d", &b);
x=busca_bin(vetor,n,b);
printf("%d", x);
int vetor[]={1,2,3,4,5}, n=5, b, x; 6
scanf ("%d", &b);
x=busca_bin(vetor,n,b);
printf("%d", x);](https://content.bartleby.com/qna-images/question/705bcef8-c7ac-4483-b54f-9a358327c6e3/b3c310a6-2b40-406f-91d8-b31d40ddd72f/yjjyxtd_thumbnail.png)

Step by stepSolved in 2 steps

- Im having trouble with this Lab for my computer science class. Define a function named SortVector that takes a vector of integers as a parameter. Function SortVector() modifies the vector parameter by sorting the elements in descending order (highest to lowest). Then write a main program that reads a list of integers from input, stores the integers in a vector, calls SortVector(), and outputs the sorted vector. The first input integer indicates how many numbers are in the list. Ex: If the input is: 5 10 4 39 12 2 the output is: 39,12,10,4,2, For coding simplicity, follow every output value by a comma, including the last one. Your program must define and call the following function:void SortVector(vector<int>& myVec)arrow_forwardCreate a program which will ask the user to enter a list of names and provide a poker tournament order. It should do the following: Ask the user how many people are playing Ask the user to enter each name Print out a sorted list of names Print out a randomized list of names to determine who sits where To store the players, use a vector of strings, e.g. vector<string> players. Use the builtin sort and random shuffle algorithms. Use range-based for loops to print out your lists.arrow_forwardCreate a program which will ask the user to enter a list of names and provide a poker tournament order. It should do the following: Ask the user how many people are playing Ask the user to enter each name Print out a sorted list of names Print out a randomized list of names to determine who sits where To store the players, use a vector of strings, e.g. vector<string> players. Use the builtin sort and random shuffle algorithms. Use range-based for loops to print out your lists.arrow_forward
- Write a function that accepts a number, N, and a vector of numbers, V. The function will return two vectors which will make up any pairs of numbers in the vector that add together to be N. Do this with nested loops so the the inner loop will search the vector for the number N-V(n) == V(m). n and m are indices in the vector of numbers. Example A = [1,2,3,4,5,6, 7] Google(5, A) Return [1,2,3,4] and [4,3,2,1] being the pairs that sum to 5. Notice that each pair appears twice. Try to write code that does not do this.arrow_forwardWrite a function maxSpan(vector) measures the span between the smallest and largest integers in an array. For instance, if the smallest was 3 and the largest was 5, then the span would be 3 (that is the numbers 3,4,5). You cannot use a loop; you must use the algorithms from the STL. #include <vector>#include <algorithm>using namespace std; int maxSpan(const vector<int>& v){ .......... }arrow_forwardExercise Write a C++ program that reads a maximum of 100 integers from the keyboard, stores them in a long array, sorts the integers in ascending order, and displays sorted output. Input can be terminated by any invalid input, such as a letter. HINT: Use the bubble sort algorithm to sort the array. This algorithm repeatedly accesses the array, comparing neighboring array elements and swapping them if needed. The sorting algorithm terminates when there are no more elements that need to be swapped. You use a flag to indicate that no elements have been swapped. Example of bubble sort algorithm: Original array: 100 50 30 70 40 After the first loop: 50 30 70 40 || 100 largest element T After the second loop: || 30 50 40 70 100 second largest elementarrow_forward
- Define a function named GetWordFrequency that takes a vector of strings and a search word as parameters. Function GetWordFrequency() then returns the number of occurrences of the search word in the vector parameter (case insensitive). Then, write a main program that reads a list of words into a vector, calls function GetWordFrequency() repeatedly, and outputs the words in the vector with their frequencies. The input begins with an integer indicating the number of words that follow. Ex: If the input is: 5 hey Hi Mark hi mark the output is: hey 1 Hi 2 Mark 2 hi 2 mark 2 Hint: Use tolower() to set the first letter of each word to lowercase before comparing. The program must define and use the following function:int GetWordFrequency(vector<string> wordsList, string currWord)arrow_forwardUsing C++ Using only these simpler vector methods Create a vector of at least 10 integers. Cout the vector with spaces in between the integers. Cout the vector backward. Using loops that traverse the elements, create a new vector with the elements arranged from high to low. Cout the new vector. Do not use iterators for this exercise, or any other advanced methods--only the ones listed below. Hint: Use multiple vectors, including the copying of vectors. vector<int> grades1 = {45, 67, 88, 45, 23}; vector<int> grades2 = grades1; May Only Use These Vector Methods: size() // Returns the number of elements empty() // Returns a boolean True if the vector is empty push_back(element) // Adds an element to the back of the vector pop_back() // Removes the element at the back of the vector front() // Returns the element at the front of the vector back()…arrow_forwardPython codearrow_forward
- numStudents is read from input as the number of input values in the vector that follow. Use two for loops to output all numStudents elements of vector walkingLogs that are: even integers • odd integers ● For both loops, follow each element with a space, including the last element, and end with a newline. Ex: If the input is 8 11 30 70 39 69 29 138 162, then the output is: 30 70 138 162 11 39 69 29 3 using namespace std; 4 5 int main() { 6 7 8 9 10 11 12 13 14 15 16 17 18 19} int numStudents; int i; cin >> numStudents; vector walkingLogs (numStudents); for (i = 0; i > walkingLogs.at(i); } * Your code goes here */ return 0; ►arrow_forwardCode in C. Solve the code below. Write a function that takes a vector of integers, an integer n representing the number of elements in that vector, and an integer b. Implement the sequential search algorithm to look for the integer b in the vector. The sequential search works as follows: you must look at all the elements of the vector until you find the b, from the first to the last. The function must return an integer representing how many elements the function tested until it found b. If it does not find the function, it must return 0. The name of the function must be called "busca_seq". int busca_seq(int vetor[], int n, int b) { //your code } My Code #include<stdio.h> int busca_seq(int vetor[], int n, int b) { int i; for(i = 0; i < n; i++){ if(vetor[i] == b) return (i+1); } { int vet[5],n = 5,b,x; vet[0] = 1; vet[1] = 2; vet[2] = 3; vet[3] = 4; vet[4] = 5; scanf("%d",&b); x = busca_seq(vet,n,b); printf("%d",x); return 0; } }arrow_forwardFinding the Minimum of a Vector Write a function to return the index of the minimum value in a vector, or -1 if the vector doesn't have any elements. In this problem you will implement the IndexOfMinimumElement function in minimum.cc and then call that function from main.cc. You do not need to edit minimum.h. Index of minimum value with std::vector Complete IndexOfMinimumElement in minimum.cc. This function takes a std::vector containing doubles as input and should return the index of the smallest value in the vector, or -1 if there are no elements. Complete main.cc Your main function asks the user how many elements they want, construct a vector, then prompts the user for each element and fills in the vector with values. Your only task in main is to call the IndexOfMinimumElement function declared in minimum.h, and print out the minimum element's index. Here's an example of how this might look: How many elements? 3 Element 0: 7 Element 1: -4 Element 2: 2 The minimum value in your…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
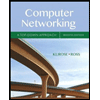
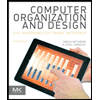
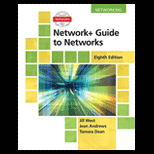
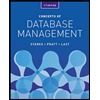
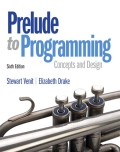
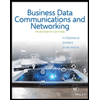