Code a Mortgage calculator using c++ and Microsoft visual Studio create a new project called exercise2 and use the code attached as exercise2.cpp. This mortgage calculation program obtains from the user a number of parameters including size of the loan, number of years, percent yearly interest rate, and the number of payments per year. It then calculates the size of the payments and the total paid over the life of the loan. (Note that the user enters percent annual interest rate while the formula uses decimal interest per year! Pay attention to variable names.) Remember to test your program by trying all different possible combinations of input. When testing your program, be sure to enter some incorrect data, such as text instead of numbers, decimal percentage rate instead of integer percentage rate, and unfilled fields to witness how the program behaves. Your program does not have to correct for bad input, but it should accept interest as percent per year. Note the use of really good variable names and the correct types. Don’t use int when you need a real number or double when you need an integer. The intermediate variable decIntRatePerPayment is a number between zero and one, not a percentage. In most cases the user knows the Annual Interest Rate rather than the decimal interest per payment. Loan duration is a positive integer while decimal interest per year is a real number. Use the formulas below to calculate the payment size. Note that the quantity within the parentheses is raised to the negative of the total number of payments. As a test case, a $100,000 loan at 8% for 15 years has a payment of $955.65 per month. Note that the factor formula uses the decimal interest per payment, not the percent interest per year obtained from the user. Note: if x and y are doubles, then pow(x, y) will raise x to the power of y. When you are finished, exercise2.cpp and submit it. sample code: /*********************************************** * mortgage Calculator. Given principle amount, percent interest * per year, loan duration in years, and number of payments per year, calculates * the payment size. Note intercap naming convention and CONSISTANT abbreviations(!) * code modified from code provided by David Beard. */ #include // includes system I/O software using namespace std; // eliminates the need for std:: int main() { double loanAmount; double percentInterestRatePerYr; // if abbreviate year w/ yr, they always use yr long loanDurationInYrs; long numPaymentsPerYr; double paymentSize = 42; double decIntRatePerPayment; long totalNumOfPayments = 42; double factor; double totalOfAllPayments; // obtain information from user cout << "Mortgage Calculator V1.0" << "\n"; cout << "Please enter the Principal: "; cin >> loanAmount; cout << "Please enter the interest rate: "; cin >> percentInterestRatePerYr; // add code to obtain loan duration and number of payments per year from user // calculate intermediate results for the following: decIntRatePerPayment = totalNumOfPayments = factor = paymentSize = totalOfAllPayments = // display results to user cout << "The payment size is: " << paymentSize << "\n"; // add a cout for the total of all payments return(0);
Code a Mortgage calculator
using c++ and Microsoft visual Studio create a new project called exercise2 and use the code attached as exercise2.cpp.
This mortgage calculation program obtains from the user a number of parameters including size of the loan, number of years, percent yearly interest rate, and the number of payments per year. It then calculates the size of the payments and the total paid over the life of the loan. (Note that the user enters percent annual interest rate while the formula uses decimal interest per year! Pay attention to variable names.)
Remember to test your program by trying all different possible combinations of input. When testing your program, be sure to enter some incorrect data, such as text instead of numbers, decimal percentage rate instead of integer percentage rate, and unfilled fields to witness how the program behaves. Your program does not have to correct for bad input, but it should accept interest as percent per year.
Note that the quantity within the parentheses is raised to the negative of the total number of payments. As a test case, a $100,000 loan at 8% for 15 years has a payment of $955.65 per month. Note that the factor formula uses the decimal interest per payment, not the percent interest per year obtained from the user.
Note: if x and y are doubles, then pow(x, y) will raise x to the power of y.
When you are finished, exercise2.cpp and submit it.
sample code:
/***********************************************
* mortgage Calculator. <your name> <date> Given principle amount, percent interest
* per year, loan duration in years, and number of payments per year, calculates
* the payment size. Note intercap naming convention and CONSISTANT abbreviations(!)
* code modified from code provided by David Beard.
*/
#include <iostream> // includes system I/O software
using namespace std; // eliminates the need for std::
int main()
{
double loanAmount;
double percentInterestRatePerYr; // if abbreviate year w/ yr, they always use yr
long loanDurationInYrs;
long numPaymentsPerYr;
double paymentSize = 42;
double decIntRatePerPayment;
long totalNumOfPayments = 42;
double factor;
double totalOfAllPayments;
// obtain information from user
cout << "Mortgage Calculator V1.0" << "\n";
cout << "Please enter the Principal: ";
cin >> loanAmount;
cout << "Please enter the interest rate: ";
cin >> percentInterestRatePerYr;
// add code to obtain loan duration and number of payments per year from user
// calculate intermediate results for the following:
decIntRatePerPayment =
totalNumOfPayments =
factor =
paymentSize =
totalOfAllPayments =
// display results to user
cout << "The payment size is: " << paymentSize << "\n";
// add a cout for the total of all payments
return(0);
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

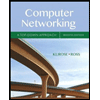
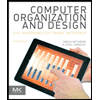
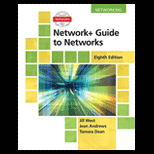
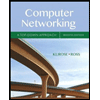
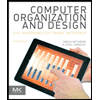
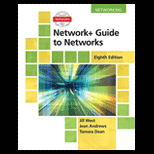
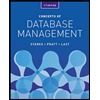
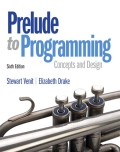
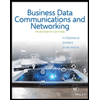