change this code to C++
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
change this code to C++
#include <stdio.h>
#include <stdlib.h>
/**
* structure to hold each point
*/
struct Point3D {
float x;
float y;
float z;
};
/**
* Parses all the vertices from the path.
* @param path The input file path.
* @param points The points storage.
* @param numPoints The number of points.
* @return points Dynamically allocated array of points.
* @return The center of mass.
*/
struct Point3D parseInput(const char *path,
struct Point3D **points,
int *numPoints) {
// to hold each record
char f_or_v;
float x;
float y;
float z;
// to store the file pointer
FILE *inFile;
// to iterate the points array
int it;
// structure to store the center of mass
struct Point3D centerOfMass;
// opening the file
inFile = fopen(path, "r");
// counting the number of points
*numPoints = 0;
while(!feof(inFile)) {
fscanf(inFile,
"%c %f %f %f\n",
&f_or_v,
&x,
&y,
&z);
*numPoints += f_or_v == 'v';
}
// dynamically allocating space
// for the points
*points =
(struct Point3D *)
malloc(sizeof(struct Point3D) *
(*numPoints));
// reading in the points
rewind(inFile);
it = 0;
while(!feof(inFile)) {
fscanf(inFile,
"%c %f %f %f\n",
&f_or_v,
&x,
&y,
&z);
if(f_or_v == 'v') {
(*points)[it] = (struct Point3D) {
.x = x,
.y = y,
.z = z,
};
++it;
}
}
// closing the file
fclose(inFile);
// calculating the center of mass
centerOfMass = (struct Point3D) {
.x = 0.0f,
.y = 0.0f,
.z = 0.0f,
};
for(it = 0; it < (*numPoints); ++it) {
centerOfMass.x += (*points)[it].x;
centerOfMass.y += (*points)[it].y;
centerOfMass.z += (*points)[it].z;
}
centerOfMass.x /= (*numPoints);
centerOfMass.y /= (*numPoints);
centerOfMass.z /= (*numPoints);
// returning the center of mass
return centerOfMass;
}
/**
* Translates points by the passed translation struct.
* @param points The points storage.
* @param numPoints The number of points.
* @param translateBy The struct to translate by.
* @return points The translated points.
*/
void translate(struct Point3D *points,
constint numPoints,
conststruct Point3D translateBy) {
// to iterate the points array
int it;
// translation
for(it = 0; it < numPoints; ++it) {
points[it].x += translateBy.x;
points[it].y += translateBy.y;
points[it].z += translateBy.z;
}
}
/**
* Stores the points into a file.
* @param path The output file path.
* @param points The points storage.
* @param numPoints The number of points.
*/
void store(const char *path,
conststruct Point3D *points,
constint numPoints) {
// to iterate the points array
int it;
// output file pointer
FILE *outFile;
// opens the file
outFile = fopen(path, "w");
// writing the points
for(it = 0; it < numPoints; ++it) {
fprintf(outFile,
"v %.2f %.2f %.2f\n",
points[it].x,
points[it].y,
points[it].z);
}
// closing the file
fclose(outFile);
}
int main(int argc, char *argv[]) {
// to store the points
struct Point3D *points;
int numPoints;
// to store the center of mass
struct Point3D centerOfMass;
// structure to translate by
struct Point3D translateBy;
// to iterate the points array
int it;
// calculating the center of mass
centerOfMass = parseInput(argv[1],
&points,
&numPoints);
// printing the center of mass
printf("Center of mass: %.2f %.2f %.2f\n",
centerOfMass.x,
centerOfMass.y,
centerOfMass.z);
// reading in the translation distances
fputs("Enter the translation vector: ", stdout);
scanf("%f %f %f",
&translateBy.x,
&translateBy.y,
&translateBy.z);
// calculating the translated points
translate(points, numPoints, translateBy);
// storing the points into a file
store(argv[2], points, numPoints);
// deallocating the array of points
free(points);
return0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
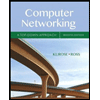
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
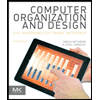
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
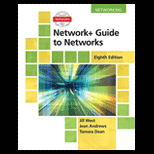
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
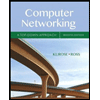
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
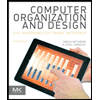
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
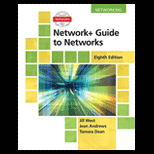
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
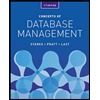
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
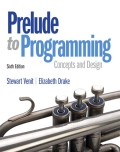
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
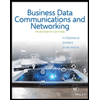
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY