# cannot contain syntax error # stack class class Stack: def __init__(self, iL=[]): self.aList = iL[:] def push(self, newElem:int): """push a new element on top""" self.aList.append(newElem) def pop(self)->int: """return top item""" return self.aList.pop() def empty(self): """Is the stack empty?""" return self.aList == [] def __repr__(self): return repr(self.aList) # use only the above stack methods to define a function to # delete an item x from a stack, (only one x (the upper one) is to be deleted) # keep the order of the remaining items in the stack unchanged # can change orginal stack # return a new stack def delx(sk:Stack, x:'item')-> Stack: #Code goes here if __name__ == "__main__": #Testing code here
# cannot contain syntax error
# stack class
class Stack:
def __init__(self, iL=[]):
self.aList = iL[:]
def push(self, newElem:int):
"""push a new element on top"""
self.aList.append(newElem)
def pop(self)->int:
"""return top item"""
return self.aList.pop()
def empty(self):
"""Is the stack empty?"""
return self.aList == []
def __repr__(self):
return repr(self.aList)
# use only the above stack methods to define a function to
# delete an item x from a stack, (only one x (the upper one) is to be deleted)
# keep the order of the remaining items in the stack unchanged
# can change orginal stack
# return a new stack
def delx(sk:Stack, x:'item')-> Stack:
#Code goes here
if __name__ == "__main__":
#Testing code here

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

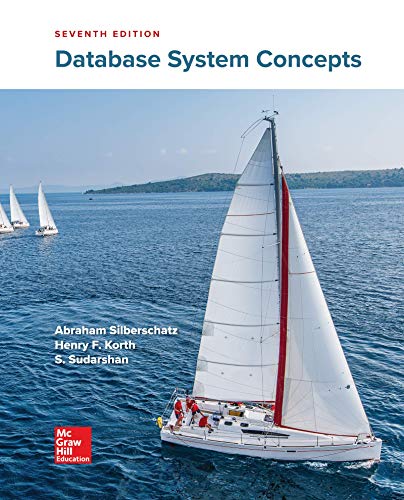
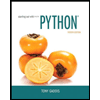
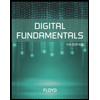
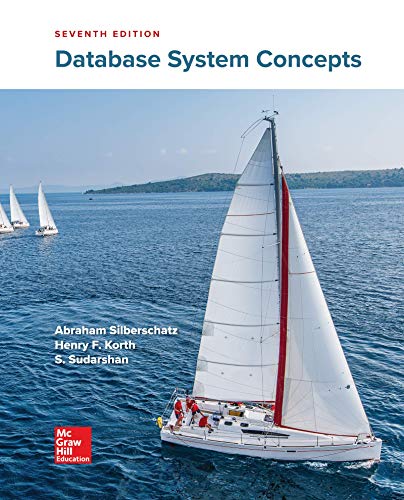
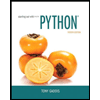
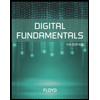
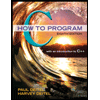
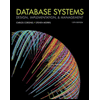
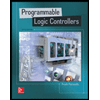