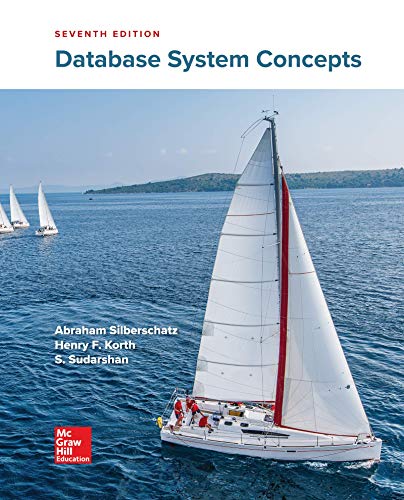
Can you convert my java code to python?
Code:
import java.util.*;
class Building
{
int x;
int y[]; // add u to y only if the path length between u and y is 2.
int count;
Building(int x,int[] y,int count)
{
this.x=x;
this.y = new int[y.length];
for(int i=0;i<y.length;i++)
{
this.y[i] = y[i];
}
this.count=count;
}
void display()
{
System.out.println("\nBuilding "+x+"->");
for(int j=0;j<y.length;j++)
{
System.out.print(y[j]+" ");
}
System.out.println("Count: "+count);
System.out.println();
}
}
public class BuildingDriver {
public static void main(String args[]) {
int n; // no. of buildings
int m; // no. of buses
Scanner in = new Scanner(System.in);
n = in.nextInt();
m = in.nextInt();
int[][] routes = new int[m][2];
Building[] build = new Building[n];
// read the m routes
for(int i=0;i<m;i++)
{
routes[i][0] = in.nextInt();
routes[i][1] = in.nextInt();
}
// determine the max no of buildings from which it is reachable
// and the buildings from which it is reachable
for(int i=0;i<n;i++)
{
int[] reachable = new int[n];
int nreach=0;
for(int j=0;j<n;j++)
{
if(i!=j)
{
// check whether there is direct route from j to i
for(int k=0;k<m;k++)
{
if(routes[k][0]==j && routes[k][1]==i)
{
reachable[nreach] = j;
nreach++;
break;
}
}
// check for route with path length 2
for(int k=0;k<m;k++)
{
if(routes[k][0]==j)
{
int adj = routes[k][1];
for(int l=0;l<m;l++)
{
if(routes[l][0]==adj && routes[l][1]==i)
{
boolean flag=false;
for(int p=0;p<nreach;p++)
{
if(reachable[p]==j)
{
flag=true;
break;
}
}
if(flag==false)
{
reachable[nreach] = j;
nreach++;
break;
}
}
}
}
}
}
}
reachable[nreach] = i;
Building b = new Building(i,reachable,nreach+1);
build[i] = b;
}
// display details
/*for(int i=0;i<n;i++)
build[i].display();*/
// find buildings that are max reachable and the count
int max=0;
for(int i=0;i<n;i++)
{
if(build[i].count > max)
{
max = build[i].count;
}
}
System.out.println(max);
// display count and buildings with max count
for(int i=0;i<n;i++)
{
if(build[i].count == max)
{
System.out.print(i+" ");
}
}
}
}

Step by stepSolved in 4 steps with 4 images

- Q2: Order of operations and math data types Use the Java IDE to create and run the following program: // Java program by [Student name] [Today's date] public class MathExample { public static void main(String[] args) { System.out.println(3/2+3); System.out.println(0.5*3+4/2); System.out.println(0.5*2/3+10/2); } } Please answer the following questions. What type of literal is 3: double or Integer? What type of literal is 0.5: double or integer? For the first expression, why is first value printed 4 instead of 4.5? For the second expression, which math operation is done last? Use parentheses to change the third expression so (3+10) is calculated first. Paste the revised expression here: Please give a full explanation of the program. Please keep in mind that I am a beginner and please keep it simple.arrow_forwarddef colours (guess tuple, hidden tuple) -> int: Preconditions: - guess is a sequence of four unique colours chosen from R, O, Y, G, B, V, - hidden is a sequence of four unique colours chosen from R, O, Y, G, B, V, Postconditions: output is number of correct colours in guess correct = 0 for colour in ('R','O','Y, 'G','B','V): if colour in guess and colour in hidden: correct = correct + 1 return correct colours (['R','B','O','G'],['R','B',V,'O']) Q1(a) What is the worst-case complexity of colours? What is the worst-case complexity of positions? Explain your answers.arrow_forwardJava programming: What's the output of the following program... a.) Compilation Error b.) 97~B~C~D~E~102~G~ c.) 65~b~C~d~E~70~G~ d.) a~66~C~68~E~f~G~arrow_forward
- Please written by computer sourcearrow_forwardFind error #include<bits/stdc++.h> using namespace std; class Solution{ public: bool isPossible(vector<int>ank,int n,int mid) { int count=0; for(int i=0;i<rank.size()) { int val= (-1 + sqrt(1+(8*mid)/rank[i]))/2; count+=val; } return count>=n; } int findMinTime(int N, vector<int>&A, int L){ int low=*min_element(A.end()),high=1000000; int ans=high; while(low<=high) { int mid=low+(high)/2; if(isPossible(A,mid)) { ans=mid; high=mid; } else low=mid; } return ans; } }; int main() { int t; cin>>t; while(t--) { int l; cin >> l; vector<int>arr(l); for(int i = 0; i < l; i++){ cin >> arr[i]; } Solution ob; int ans = ob.findMin(n, *arr, l); cout…arrow_forwardJAVA Language: Transpose Rotate. Question: Modify Transpose.encode() so that it uses a rotation instead of a reversal. That is, a word like “hello” should be encoded as “ohell” with a rotation of one character. (Hint: use a loop to append the letters into a new string) import java.util.*; public class TestTranspose { public static void main(String[] args) { String plain = "this is the secret message"; // Here's the message Transpose transpose = new Transpose(); String secret = transpose.encrypt(plain); System.out.println("\n ********* Transpose Cipher Encryption *********"); System.out.println("PlainText: " + plain); // Display the results System.out.println("Encrypted: " + secret); System.out.println("Decrypted: " + transpose.decrypt(secret));// Decrypt } } abstract class Cipher { public String encrypt(String s) { StringBuffer result = new…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
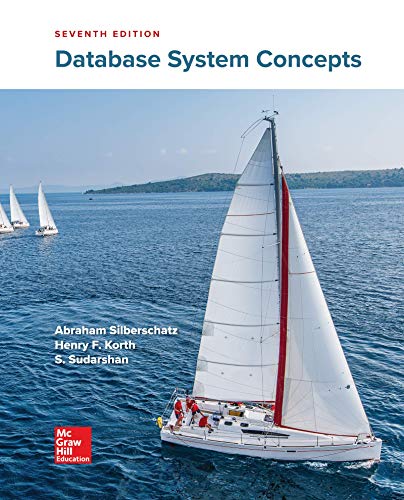
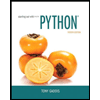
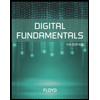
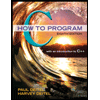
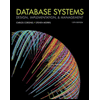
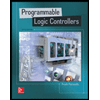