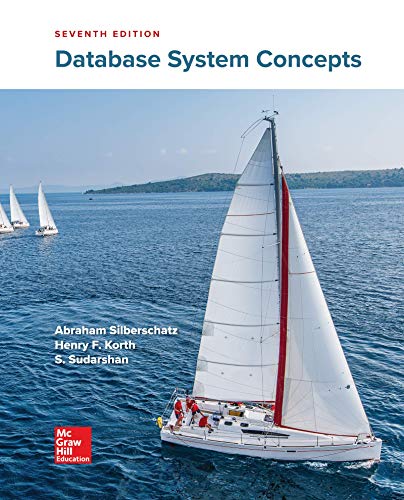
(c90)In my code below , can you help me achieve all the points in the image :
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <float.h>
#include "graph.h"
#include "dijkstra.h"
#define INFINITY DBL_MAX
/* find shortest paths between source node id and all other nodes in graph. */
/* upon success, returns an array containing a table of shortest paths. */
/* return NULL if *graph is uninitialised or an error occurs. */
/* each entry of the table array should be a Path */
/* structure containing the path information for the shortest path between */
/* the source node and every node in the graph. If no path exists to a */
/* particular desination node, then next should be set to -1 and weight */
/* to DBL_MAX in the Path structure for this node */
Path *dijkstra(Graph *graph, int id, int *pnEntries)
{
int n;
int i, j;
int* nv = get_vertices(graph, &n);
int *S = malloc(n * sizeof(int));
double *D = malloc(n * sizeof(double));
int *R = malloc(n * sizeof(int));
Path *table = malloc(n * sizeof(Path));
/* check if graph and starting node are valid */
if (graph == NULL || id < 0 || id >= n)
{
*pnEntries = 0;
return NULL;
}
/* initialize S to contain all the networks (vertices) except the source node */
for (i = 0, j = 0; i < n; i++)
{
if (i != id)
{
S[j++] = i;
}
}
/* initialize D with the weights of the edges from the source node, or infinity if no edge exists */
for (i = 0; i < n; i++)
{
Edge *edge = get_edge(graph, id, i);
if (edge != NULL)
{
D[i] = edge_weight(edge);
R[i] = id;
}
else
{
D[i] = INFINITY;
R[i] = 0;
}
}
/* repeatedly follow the remaining rules of Dijkstra's
while (n > 1)
{
/* find the vertex in S with the smallest value in D */
int u = S[0];
double min = D[u];
for (i = 1; i < n - 1; i++)
{
if (D[S[i]] < min)
{
u = S[i];
min = D[u];
}
}
/* remove vertex u from S */
for (i = 0; i < n - 1; i++)
{
if (S[i] == u)
{
S[i] = S[n - 2];
break;
}
}
n--;
/* update the values in D and R for the remaining vertices in S */
for (i = 0; i < n - 1; i++)
{
int v = S[i];
Edge *edge = get_edge(graph, u, v);
if (edge != NULL && D[v] > D[u] + edge_weight(edge))
{
D[v] = D[u] + edge_weight(edge);
R[v] = u;
}
}
}
/* create the routing table to be returned */
for (i = 0; i < n; i++)
{
table[i].next_hop = R[i];
table[i].weight = D[i];
}
/* free unused memory and set *pnEntries to the correct value */
free(S);
free(D);
free(R);
*pnEntries = n;
free(nv);
return table;
}
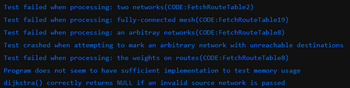

Step by stepSolved in 4 steps with 4 images

- Java Program: please choose an aswer:arrow_forwardSubject is C++ programmingarrow_forwardData Structures and algorithms: Topic: Doubly and circular Linked Lists in java: Please solve this on urgent basis: Attach output's picture and explain every statement in commments: Implemented Circular Linked List CODE: #include <bits/stdc++.h> // include the standard c++ header fileusing namespace std; class Node // definition of the class for the node of the linked list{public: int data; Node* next;}; void push(Node** head_ref, int data) // definition of the function to insert the element in the list{ Node* ptr1 = new Node(); ptr1->data = data; ptr1->next = *head_ref; if (*head_ref != NULL) // when the head of the list does not points to null { Node* temp = *head_ref; while (temp->next != *head_ref) temp = temp->next; temp->next = ptr1; } else…arrow_forward
- 7. a) Write down the 5 by 5 adjacency matrix for the undirected graph below (Figure 1). A B C D Figure 1: Undirected Graph for Question 7. (a) b) Write a program that asks user to enter number of vertices in an undirected graph and then the adjacency matrix representing the undirected graph. The program, then, must display whether the given graph is connected or not. You will find two sample runs of the program below. Sample 1 Sample 2 Enter number of vertices: 3 Enter number of vertices: 3 Enter adjacency matrix: 0 1 1 10 0 1 0 0 Enter adjacency matrix: 0 1 0 1 0 0 0 0 0 The graph is connected. The graph is not connected.arrow_forwardimport java.lang.System; 3. public class FibonacciComparison { 4. // Fibonacci Sequence: 0, 1, 1, 2, 3, 5, 8 .... /* 7 input cases 8. 1) 0 9 2) 3 10 3) -1 11 4) 9 12 output cases 13 1) 0 14 2) 2 15 3) 0 16 4) 34 17 */ // Note that you need to return 0 if the input is negative. // Please pay close attention to the fact that the first index in our fib sequence is 0. 18 19 20 // Recursive Fibonacci public static int fib(int n) { // Code this func. 21 22 23 24 return -1; 25 26 // Iterative Fibonacci 27 28 public static int fiblinear(int n) { // Code this func. 29 30 return -1; 31 32 33 public static void main(String[] args) { 34 // list of fibonacci sequence numbers int[] nlist w { 5,10, 15, 20, 25, 30, 35, 40, 45}; 35 36 37 // Two arrays (one for fibLinear, other for fibRecursive) to store time for each run. // There are a total of nlist.length inputs that we will test double[] timingsEF = new double[nlist.Length]; double[] timingsLF = new double[nlist.length]; 38 39 40 41 42 // Every…arrow_forward#include <stdio.h>#include <stdlib.h>#include <string.h> typedef struct LINKED_STACK_NODE_s *LINKED_STACK_NODE; typedef struct LINKED_STACK_NODE_s{LINKED_STACK_NODE next;void *data;} LINKED_STACK_NODE_t[1]; typedef struct LINKED_STACK_s{LINKED_STACK_NODE head;int count;} LINKED_STACK_t[1], *LINKED_STACK; typedef struct{int R;int C;} POS_t[1], *POS; LINKED_STACK stack_init();void stack_free(LINKED_STACK stack);void stack_push(LINKED_STACK stack, void *data);void *stack_pop(LINKED_STACK stack);void *stack_top(LINKED_STACK stack);int is_empty(LINKED_STACK stack); int is_empty(LINKED_STACK stack){return stack->head == NULL;} LINKED_STACK stack_init(){LINKED_STACK stack = (LINKED_STACK)malloc(sizeof(LINKED_STACK_t));if (stack == NULL){printf("\nproblem with initializing stack\n\n");return NULL;}stack->head = NULL;stack->count = 0;return stack;} void stack_free(LINKED_STACK stack){while (is_empty(stack) == 0){stack_pop(stack);}free(stack);}void…arrow_forward
- FASTarrow_forwardProblem overview As you may already know from earlier courses, integer values in C++ (as well as other programming languages) are limited by the number of bits used to represent these data. For instance, a 64-bit unsigned integer has the maximum value of 2^64 - 1 or 18446744073709551615. One method of representing integers of arbitrary length, which was covered last spring, is a a linked list data structure such that that a node in the linked list corresponds to a single digit in the integer. For instance, the number 123 can be stored as linked-list that could look like this: [ 3 ] -> [ 2 ] -> [ 1 ] -> NULLNote that the first (or head) node in this list contains the least significant digit (in this case 3), while the last node contains the most significant digit (1). For this problem, you are to read in pairs of arbitrary length integers and produce correct output as outlined below. InspirationNote, this problem is inspired by Problem 8.19 from Elements of Programming…arrow_forwardconvert C++ STL code into Python:int main(){ // empty map container map<int, int> gquiz1; // insert elements in random order gquiz1.insert(pair<int, int>(1, 40)); gquiz1.insert(pair<int, int>(2, 30)); gquiz1.insert(pair<int, int>(3, 60)); gquiz1.insert(pair<int, int>(4, 20)); gquiz1.insert(pair<int, int>(5, 50)); gquiz1.insert(pair<int, int>(6, 50)); gquiz1.insert(pair<int, int>(7, 10)); // printing map gquiz1 map<int, int>::iterator itr; cout << "\nThe map gquiz1 is : \n"; cout << "\tKEY\tELEMENT\n"; for (itr = gquiz1.begin(); itr != gquiz1.end(); ++itr) { cout << '\t' << itr->first << '\t' << itr->second << '\n'; } cout << endl; return 0;}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
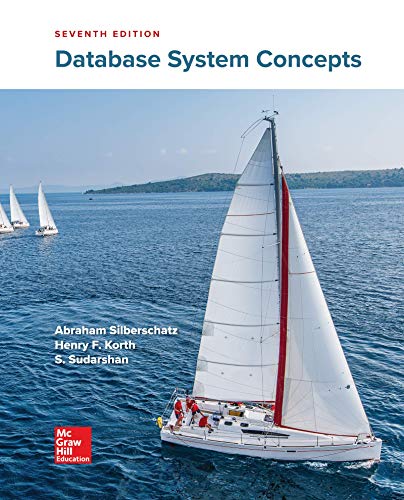
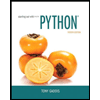
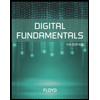
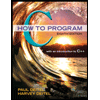
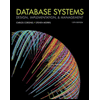
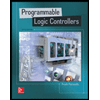