def count_crossings_and_nestings(arcs): Count the number of crossings and nestings between a set of arcs. Parameters: arcs (list): A list of tuples representing arcs. Each tuple contains two integers representing the start and end points of an arc. Returns: tuple: A tuple containing the number of crossings and nestings. || || || crossings = 0 nestings = 0 num_arcs = len(arcs) # Get the number of arcs using the len() function for i in range(num_arcs): for j in range(i+1, num_arcs): arci_start, arci_end = arcs [i] arcj_start, arcj_end: = arcs [j] # Check for crossings if arci_start < arcj_start < arci_end < arcj_end: crossings += 1 elif arcj_start < arci_start < arcj_end < arci_end: crossings += 1 # Check for nestings elif arci_start < arcj_start < arcj_end < arci_end: nestings += 1 elif arcj_start < arci_start < arci_end < arcj_end: nestings += 1 return crossings, nestings # Test the function arcs = [(1, 4), (0, 6), (3, 5), (2, 9), (7, 8)] crossings, nestings = count_crossings_and_nestings (arcs) print (f"Number of crossings: {crossings}") # Expected: 1 print (f"Number of nestings: {nestings}") Number of crossings: 3 Number of nestings: 4 # Expected: 3
def count_crossings_and_nestings(arcs): Count the number of crossings and nestings between a set of arcs. Parameters: arcs (list): A list of tuples representing arcs. Each tuple contains two integers representing the start and end points of an arc. Returns: tuple: A tuple containing the number of crossings and nestings. || || || crossings = 0 nestings = 0 num_arcs = len(arcs) # Get the number of arcs using the len() function for i in range(num_arcs): for j in range(i+1, num_arcs): arci_start, arci_end = arcs [i] arcj_start, arcj_end: = arcs [j] # Check for crossings if arci_start < arcj_start < arci_end < arcj_end: crossings += 1 elif arcj_start < arci_start < arcj_end < arci_end: crossings += 1 # Check for nestings elif arci_start < arcj_start < arcj_end < arci_end: nestings += 1 elif arcj_start < arci_start < arci_end < arcj_end: nestings += 1 return crossings, nestings # Test the function arcs = [(1, 4), (0, 6), (3, 5), (2, 9), (7, 8)] crossings, nestings = count_crossings_and_nestings (arcs) print (f"Number of crossings: {crossings}") # Expected: 1 print (f"Number of nestings: {nestings}") Number of crossings: 3 Number of nestings: 4 # Expected: 3
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 2PE
Related questions
Question
100%
Look at my code, What can you say about the computational complexity of count_crossings_and_nestings? Discuss how run time and memory requirement grow as the number n of arcs increases. Give reasons.
![def count_crossings_and_nestings(arcs):
Count the number of crossings and nestings between a set of arcs.
Parameters:
arcs (list): A list of tuples representing arcs. Each tuple contains two integers representing the start and end points of an arc.
Returns:
tuple: A tuple containing the number of crossings and nestings.
|| || ||
crossings = 0
nestings
= 0
num_arcs = len(arcs) # Get the number of arcs using the len() function
for i in range(num_arcs):
for j in range(i+1, num_arcs):
arci_start, arci_end = arcs [i]
arcj_start, arcj_end: = arcs [j]
# Check for crossings
if arci_start < arcj_start < arci_end < arcj_end:
crossings += 1
elif arcj_start < arci_start < arcj_end < arci_end:
crossings += 1
# Check for nestings
elif arci_start < arcj_start < arcj_end < arci_end:
nestings += 1
elif arcj_start < arci_start < arci_end < arcj_end:
nestings += 1
return crossings, nestings
# Test the function
arcs = [(1, 4), (0, 6), (3, 5), (2, 9), (7, 8)]
crossings, nestings = count_crossings_and_nestings (arcs)
print (f"Number of crossings: {crossings}") # Expected: 1
print (f"Number of nestings: {nestings}")
Number of crossings: 3
Number of nestings: 4
# Expected: 3](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F033c0d82-90fb-4c58-9b03-1325bfffdb8d%2F612e385a-da50-4cbf-8d5f-6d4ea0df8207%2Fdrzicul_processed.png&w=3840&q=75)
Transcribed Image Text:def count_crossings_and_nestings(arcs):
Count the number of crossings and nestings between a set of arcs.
Parameters:
arcs (list): A list of tuples representing arcs. Each tuple contains two integers representing the start and end points of an arc.
Returns:
tuple: A tuple containing the number of crossings and nestings.
|| || ||
crossings = 0
nestings
= 0
num_arcs = len(arcs) # Get the number of arcs using the len() function
for i in range(num_arcs):
for j in range(i+1, num_arcs):
arci_start, arci_end = arcs [i]
arcj_start, arcj_end: = arcs [j]
# Check for crossings
if arci_start < arcj_start < arci_end < arcj_end:
crossings += 1
elif arcj_start < arci_start < arcj_end < arci_end:
crossings += 1
# Check for nestings
elif arci_start < arcj_start < arcj_end < arci_end:
nestings += 1
elif arcj_start < arci_start < arci_end < arcj_end:
nestings += 1
return crossings, nestings
# Test the function
arcs = [(1, 4), (0, 6), (3, 5), (2, 9), (7, 8)]
crossings, nestings = count_crossings_and_nestings (arcs)
print (f"Number of crossings: {crossings}") # Expected: 1
print (f"Number of nestings: {nestings}")
Number of crossings: 3
Number of nestings: 4
# Expected: 3
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
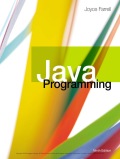
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
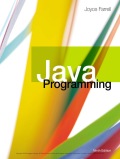
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT