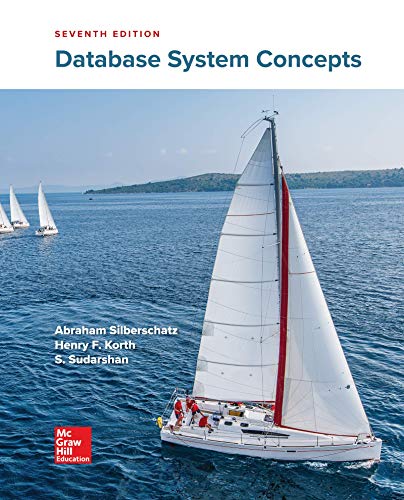
Concept explainers
C++
Shape is an abstract class with a pure virtual function: Area(). (2) Circle is inherited from Shape, with a data member Radius, and a member function Area() to get the area of a circle. (3) Triangle is inherited from Shape, with two data members Bottom-length and Height, and a member function Area() to get the area of a triangle.
Please complete the definition of Shape, Circle and Triangle, and define other necessary functions, to let a user can use Shape, Circle and Triangle as follows:
void main( )
{
Shape* p = new Circle(2);
cout << "The area of the circle is: " << PrintArea(*p) << endl;
Triangle triangle (3,4);
cout << "The area of the triangle is: " << PrintArea(triangle) << endl;
delete p;
}
The outputs:
The area of the circle is: 12.56
The area of the triangle is: 6
//Your codes with necessary explanations:
My code:
#include <iostream>
using namespace std;
class Shape
{
public:
double area;
virtual void Area() = 0;
void PrintArea();
};
class Triangle : public Shape
{
double Bottom_length, Height;
public:
Triangle(double l, double h)
{
Bottom_length=l;
Height = h;
}
void Area()
{
area= 0.5* Bottom_length*Height;
}
void PrintArea(){
cout<<area;
}
};
class Circle : public Shape
{
double Radius;
public:
Circle(double r)
{
Radius=r;
}
void Area(){
area=3.14*Radius*Radius;
}
void PrintArea(){
cout<<area;
}
};
Void main()
{
Shape* p = new Circle(2);
cout << "The area of the circle is: " << PrintArea(*p) << endl;
Triangle triangle (3,4);
cout << "The area of the triangle is: " << PrintArea(triangle) << endl;
delete p;
}
Please remove errors and run this code

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- 14. What is an object in C++? Group of answer choices An object is a class declared entirely within the body of another class or interface. An object is an extensible program-code-template for creating classes, providing initial values for state (member variables) and implementations of behavior (member functions, methods) Anything that may be apprehended intellectually. An object refers to a particular instance of a class where the object can be a combination of variables, functions, and data structures. Anything that is visible or tangible and is relatively stable in form.arrow_forwardQ 2. Implement in C++ the design of “Employee" as an abstract data type, declaring at least TWO main behaviors (ADT) Q 4. Answer the following: a) Write the necessary statements in C++ that create at least two objects of the above "Employee" ADT, b) Write the necessary statements in C++ that show the use of each of those behaviors on the two objects|arrow_forwardc++arrow_forward
- Submit the following Zipped: 1) A UML Class Diagram of the the class/abstract data type. 2) Implementation files of the class and its member functions. 3) A driver program exercising your class to test and demonstrate its functionality.arrow_forwardConsider the following definition of class Box and main function. Assuming constructor, copy constructor, assignment operator, and destructor of this class are properly without any error implemented, answer the following four questions: class Box { public: Box(); ~Box(); Box (const Box & source); fr Box& operator= (const Box & rhs); private: int* pointer; }; int main(void) { Box X; Box y(x); Box *z= new Box; x = y; return 0;arrow_forward1. Write a program in the C++ language to create a class Triangle with three member integer variables side1, side2, and side3. Add the parameterized constructor for the class and add a member function findArea that displays the area of the triangle in the output.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
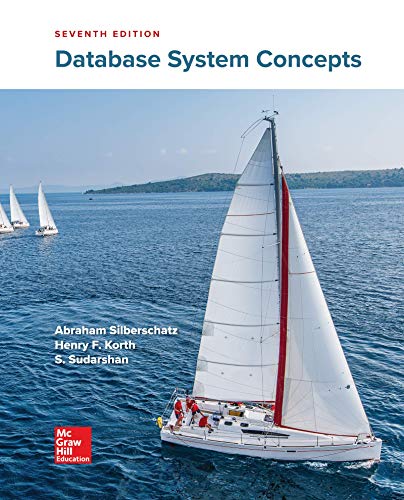
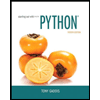
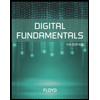
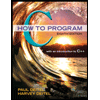
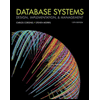
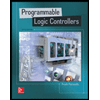