C++ Programming. I need to develop my original code. Please help me write a C++ code following this instructions: (Please write your own code, not copy other contents please) The company needs a more detailed report to be generated at the end of each shift. The sample screen output with the report is listed below: Welcome to CTC Taxi. Do you need a taxi? Y Taxi CTC0001 will pick you up in a few minutes. (Taxi CTC0001 determined that there were 3 passengers) Do you need a taxi? Y Taxi CTC0004 will pick you up in a few minutes. (Taxi CTC0004 determined that there were 5 passengers) …… …… Do you need a taxi? N CTC Taxi served a total of 124 passengers today. CTC0001 CTC00022 CTC0003 CTC0004 CTC0005 CTC0006 19 Calls 25 Calls 10 Calls 12 Calls 22 Calls 13 Calls 25 Passengers 45 Passengers 34 Passengers 29 Passengers 24 Passengers 30 Passengers Today CTC0002 served most passengers. Today on average each taxi served 31.7 passengers. You must include the following parts in your program: Overload + to add total calls and total passengers of all taxis Overload < to determine which taxi served most passengers Store the report in a disk file --------------------------------------------------------------------- Original code is: #include #include #include using namespace std; #define SIZE 50 class Taxi { private: string taxi_id; string driver_name; string make; string model; string color; string license;
C++ Programming.
I need to develop my original code.
Please help me write a C++ code following this instructions:
(Please write your own code, not copy other contents please)
The company needs a more detailed report to be generated at the end of each shift. The sample screen output with the report is listed below:
Welcome to CTC Taxi.
Do you need a taxi?
Y
Taxi CTC0001 will pick you up in a few minutes. (Taxi CTC0001 determined that there were 3 passengers)
Do you need a taxi?
Y
Taxi CTC0004 will pick you up in a few minutes. (Taxi CTC0004 determined that there were 5 passengers)
……
……
Do you need a taxi?
N
CTC Taxi served a total of 124 passengers today.
CTC0001 CTC00022 CTC0003 CTC0004 CTC0005 CTC0006
19 Calls 25 Calls 10 Calls 12 Calls 22 Calls 13 Calls
25 Passengers 45 Passengers 34 Passengers 29 Passengers 24 Passengers 30 Passengers
Today CTC0002 served most passengers.
Today on average each taxi served 31.7 passengers.
You must include the following parts in your program:
- Overload + to add total calls and total passengers of all taxis
- Overload < to determine which taxi served most passengers
- Store the report in a disk file
---------------------------------------------------------------------
Original code is:
#include <iostream>
#include <fstream>
#include <time.h>
using namespace std;
#define SIZE 50
class Taxi
{
private:
string taxi_id;
string driver_name;
string make;
string model;
string color;
string license;
int num_passenger;
public:
Taxi():taxi_id(""), driver_name(""), make(""), model(""), color(""), license(""), num_passenger(0)
{}
void setTaxiID(string taxi_id)
{
this->taxi_id = taxi_id;
}
void setDriverName(string driver_name)
{
this->driver_name = driver_name;
}
void setMake(string make)
{
this->make = make;
}
void setModel(string model)
{
this->model = model;
}
void setColor(string color)
{
this->color = color;
}
void setLicenseNumber(string license)
{
this->license = license;
}
void setNumPassengers(int num_passenger)
{
this->num_passenger = num_passenger;
}
string getTaxiID() {return taxi_id;}
string getDriverName() {return driver_name;}
string getMake() {return make;}
string getModel() {return model;}
string getColor() {return color;}
string getLicense() {return license;}
int getPassengers() {return num_passenger;}
};
int readRecords(Taxi taxi[]);
int main()
{
Taxi taxi[50];
int numTaxi = readRecords(taxi);
ofstream out;
out.open("CTC.dat");
if(out.fail())
{
cout<<"Unable to open output file: CTC.dat... Terminating"<<endl;
}
else
{
for(int i=0;i<numTaxi;i++)
{
out<<taxi[i].getTaxiID()<<endl<<taxi[i].getDriverName()<<endl<<taxi[i].getMake()<<endl
<<taxi[i].getModel()<<endl<<taxi[i].getColor()<<endl<<taxi[i].getLicense()<<endl
<<taxi[i].getPassengers();
if(i < numTaxi-1)
out<<endl;
}
out.close();
}
cout << "\nWelcome to Citywide Taxi Company (CTC)." <<endl;
char c = 'Y';
srand(time(0));
int total = 0;
while(c == 'Y'){
cout<<"Do you need a taxi?"<<endl;
cin >> c;
if(c != 'Y')
break;
int i = rand()%numTaxi;
int passenger = 1 + rand()%4;
cout<<"Taxi "<<taxi[i].getTaxiID()<< " a " <<taxi[i].getColor()<<" "<<taxi[i].getMake()<<" "<<taxi[i].getModel() << " driven by "<<taxi[i].getDriverName()<< " will pick you up in a few minutes."<< endl;
taxi[i].setNumPassengers(passenger + taxi[i].getPassengers());
total += passenger;
}
cout<<endl;
cout<<"CTC served a total of " << total << " passengers today."<<endl;
for(int i=0; i<6; i++){
if(taxi[i].getPassengers() > 0)
cout<<taxi[i].getDriverName() << " served " << taxi[i].getPassengers() << " passengers."<<endl;
}
return 0;
}
int readRecords(Taxi taxi[])
{
cout<<"Enter records for upto 50 taxi(enter blank string for taxi id to exit):"<<endl;
bool done= false;
int count = 0;
string taxi_id;
string driver_name;
string make;
string model;
string color;
string license;
while(!done)
{
cout<<"Enter details for Taxi-"<<count+1<<":"<<endl;
cout<<"Taxi ID: ";
getline(cin, taxi_id);
if(taxi_id.length() == 0)
done = true;
else
{
cout<<"Driver name: ";
getline(cin, driver_name);
cout<<"Car maker: ";
getline(cin, make);
cout<<"Car model: ";
getline(cin, model);
cout<<"Car color: ";
getline(cin, color);
cout<<"License number: ";
getline(cin, license);
taxi[count].setTaxiID(taxi_id);
taxi[count].setColor(color);
taxi[count].setDriverName(driver_name);
taxi[count].setLicenseNumber(license);
taxi[count].setMake(make);
taxi[count].setModel(model);
count++;
if(count == SIZE)
done = true;
}
}
return count;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

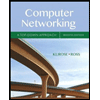
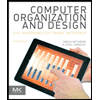
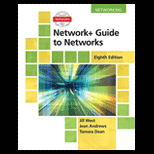
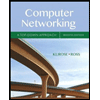
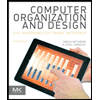
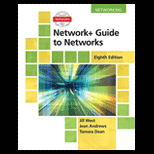
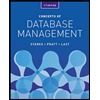
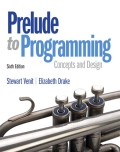
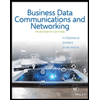