C++ Dont include new files. READ ME Pets Breed Class Create a Breed class with the following: Member Variables Create the following private member variables, all of type std::string: species_ breed_name_ color_ Constructors Create a default constructor for Breed that sets its species_ to "Dog", breed_name_ to "Pug", and color_ to "Fawn". Create a non-default constructor that receives a std::string for species_, breed_name_, and color_; in that order. The values from the constructor should appropriately assign the member variables. Accessors and Mutators Create accessors and mutators for all member variables, following the naming conventions covered in class. e.g. for species_, name the accessor Species, and the mutator SetSpecies. Pet Class Create a Pet class with the following: Member Variables Create the following private member variables: std::string name_ Breed breed_ double weight_ Constructors Create a default constructor for Pet that sets its name to "Doug" and weight to 15.6. The Breed object will automatically be created using its default constructor. Create a non-default constructor that receives an std::string for name_, Breed for breed_, and a double for weight_ in that order. The values from the constructor should appropriately assign the member variables. Create another non-default constructor that receives an std::string for name_, std::string species_, name_, and color_ for the Breed constructor, and double for weight_. The values from the constructor should appropriately assign the data members. Accessors and Mutators Create accessors and mutators for name_, breed_, and weight_. Please name the accessor for breed_ as GetBreed, to avoid conflicting with the constructor for the Breed class. SetBreed overload Create a function overload for SetBreed that accepts a species_, name_, and color_, all of type std::string, that will internally create a Breed object using the values provided and then assign it to the breed_ member variable. Print Create a member function called Print that returns void and does not take in any parameters. Using the member variables, this function should print out the name and weight of the Pet. It should also utilize accessors of the Breed class to get the species, breed name, and color. Other instructions Complete the main function as described. Place the Pet class in pet.h, and the Breed class in breed.h. Member functions that take more than ten lines or use complex constructs should have their function prototype in the respective .h header file and implementation in the respective cc implementation file.
C++ Dont include new files.
READ ME
Pets
Breed Class
Create a Breed class with the following:
Member Variables
Create the following private member variables, all of type std::string:
species_
breed_name_
color_
Constructors
Create a default constructor for Breed that sets its species_ to "Dog", breed_name_ to "Pug", and color_ to "Fawn".
Create a non-default constructor that receives a std::string for species_, breed_name_, and color_; in that order. The values from the constructor should appropriately assign the member variables.
Accessors and Mutators
Create accessors and mutators for all member variables, following the naming conventions covered in class. e.g. for species_, name the accessor Species, and the mutator SetSpecies.
Pet Class
Create a Pet class with the following:
Member Variables
Create the following private member variables:
std::string name_
Breed breed_
double weight_
Constructors
Create a default constructor for Pet that sets its name to "Doug" and weight to 15.6. The Breed object will automatically be created using its default constructor.
Create a non-default constructor that receives an std::string for name_, Breed for breed_, and a double for weight_ in that order. The values from the constructor should appropriately assign the member variables.
Create another non-default constructor that receives an std::string for name_, std::string species_, name_, and color_ for the Breed constructor, and double for weight_. The values from the constructor should appropriately assign the data members.
Accessors and Mutators
Create accessors and mutators for name_, breed_, and weight_. Please name the accessor for breed_ as GetBreed, to avoid conflicting with the constructor for the Breed class.
SetBreed overload
Create a function overload for SetBreed that accepts a species_, name_, and color_, all of type std::string, that will internally create a Breed object using the values provided and then assign it to the breed_ member variable.
Print
Create a member function called Print that returns void and does not take in any parameters. Using the member variables, this function should print out the name and weight of the Pet. It should also utilize accessors of the Breed class to get the species, breed name, and color.
Other instructions
Complete the main function as described. Place the Pet class in pet.h, and the Breed class in breed.h. Member functions that take more than ten lines or use complex constructs should have their function prototype in the respective .h header file and implementation in the respective cc implementation file.
main.cc file
#include <iostream>
int main() {
// == YOUR CODE HERE ==
// 1. Create a
// Don't forget to #include <vector> and "pet.h"
// ====
std::string name;
std::string breed_name;
std::string species;
std::string color;
double weight = 0.0;
do {
std::cout << "Please enter the pet's name (q to quit): ";
std::getline(std::cin, name);
if (name != "q") {
std::cout << "Please enter the pet's species: ";
std::getline(std::cin, species);
std::cout << "Please enter the pet's breed: ";
std::getline(std::cin, breed_name);
std::cout << "Please enter the pet's color: ";
std::getline(std::cin, color);
std::cout << "Please enter the pet's weight (lbs): ";
std::cin >> weight;
std::cin.ignore();
// == YOUR CODE HERE ==
// 2. Create a Pet object using the input from the user
// Store the newly-created Pet object into the vector.
// ====
}
} while (name != "q");
std::cout << "Printing Pets:\n";
// ==== YOUR CODE HERE ====
// 3. Print information about each pet in the `pets`
// vector by writing a loop to access each Pet object.
// ====
return0;
}
pet.h file
#include <string>
#include "breed.h"
// == YOUR CODE HERE ==
// Write the Pet class here. Refer to the README for the member
// variables, constructors, and member functions needed.
//
// Note: mark functions that do not modify the member variables
// as const, by writing `const` after the parameter list.
// Pass objects by const reference when appropriate.
// Remember that std::string is an object!
// ===
breed.h file
#include <string>
// == YOUR CODE HERE ==
// Write the Breed class here. Refer to the README for the member
// variables, constructors, and member functions needed.
//
// Note: you may define all functions inline in this file.
// ====
pet.cc file
#include "pet.h"
#include <iomanip>
#include <iostream>
// == YOUR CODE HERE ==
// This implementation file (pet.cc) is where you should implement
// the member functions declared in the header (pet.h), only
// if you didn't implement them inline within pet.h.
//
// Remember to specify the name of the class with :: in this format:
// <return type> MyClassName::MyFunction() {
// ...
// }
// to tell the compiler that each function belongs to the Pet class.
// ===


Step by step
Solved in 8 steps

I still dont understand the addition of a new file breed.cc when there should only be 4 files. Main.cc, breed.h, pet.h, and pet.cc. How would I create a new file or even add it into an existing file of the 4 available.
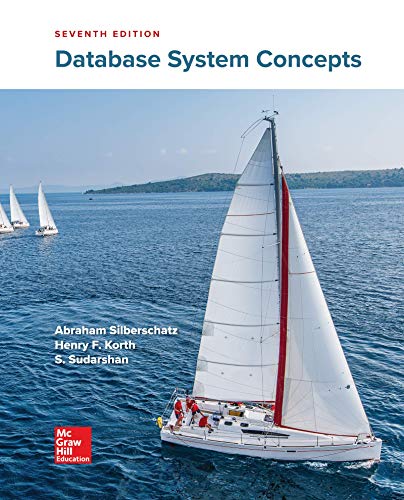
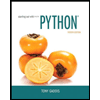
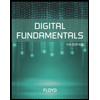
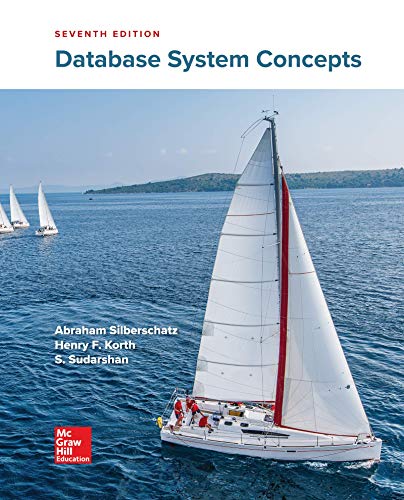
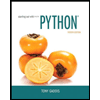
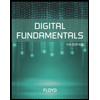
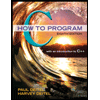
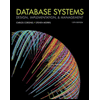
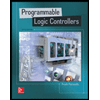