C++ Do not use file .txt and #include just use cin Write a program that declares a struct to store the data of a football player (player’s name, player’s position, number of touchdowns, num- ber of catches, number of passing yards, number of receiving yards, and the number of rushing yards). Declare an array of 10 components to store the data of 10 football players. Your program must contain a function to input data and a function to output data. Add functions to search the array to find the index of a specific player, and update the data of a player. (You may assume that the input data is stored in a file.) Before the program terminates, give the user the option to save data in a file. Your program should be menu driven, giving the user various choices.
C++
Do not use file .txt and #include <ifstream>
just use cin
Write a
player (player’s name, player’s position, number of touchdowns, num-
ber of catches, number of passing yards, number of receiving yards,
and the number of rushing yards). Declare an array of 10 components
to store the data of 10 football players. Your program must contain a
function to input data and a function to output data. Add functions to
search the array to find the index of a specific player, and update the
data of a player. (You may assume that the input data is stored in a file.)
Before the program terminates, give the user the option to save data
in a file. Your program should be menu driven, giving the user various
choices.

Step by step
Solved in 3 steps with 3 images

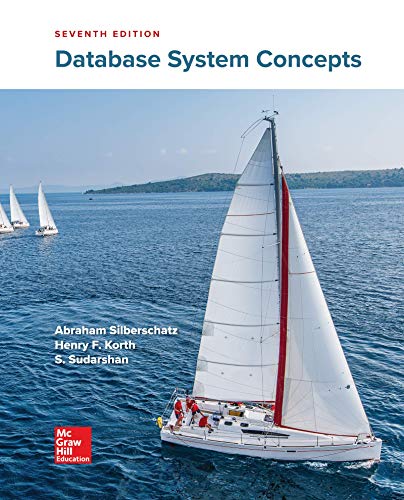
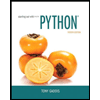
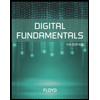
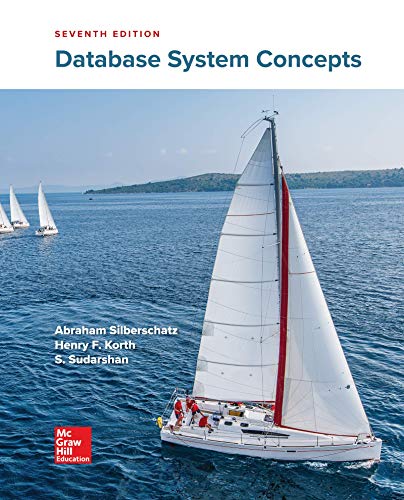
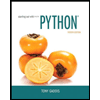
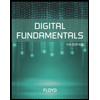
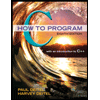
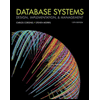
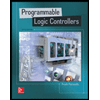