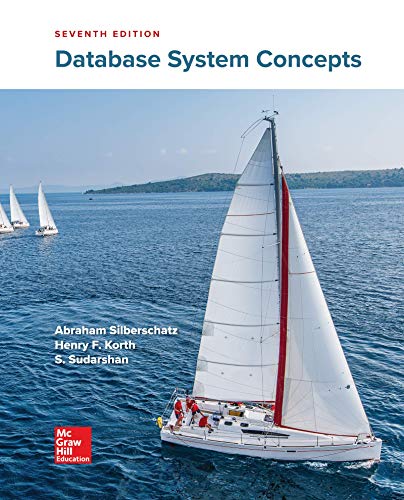
Concept explainers
C++
Assume that a class named Window has been defined, and it has two int member variables named width and height. Write a function that overloads the >> operator for the Window class. The function should accept a reference to an istream object and a reference to a Window object as arguments. The function should read two values from the istream object, into the width and height member variables respectively. Don't forget to have the function return the proper value as well. Assume that the function has already been declared as a friend in the Window class with the following statement: friend istream &operator >> (istream &, Window &);
No hand written and fast answer with explanation

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

Write this in C++ only.
Write this in C++ only.
- Task 2: use c++ to Create an employee class, basing it on Task 1. The member data should comprise an int for storing the employee number and a float for storing the employee’s compensation. Member functions should allow the user to enter this data and display it. Write a main() that allows the user to enter data for three employees and display it.arrow_forwardCreate a User class with the following features (C++): A Private class member fname that is a string A Private class member lname that is a string A setter function setFName(string s) that sets the fname member A setter function setLName(string s) that sets the lname member A getter function getName() that returns the full name with a space between the first and last names Use the following code and save the class in a header file User.hpparrow_forwardIn C++arrow_forward
- C++ Write a function named “createPurchaseOrder” that accepts the quantity(integer), the cost per item (double), and the description (string). It will return anewly created PurchaseOrder object holding that information if they are valid:quantity and cost per item cannot be negative and the description cannot be empty or blank(s). When it is invalid, it will return NULL to indicate that it cannot create such PurchaseOrder object. PurchaseOrder createPurchaseOrder(int quantity, double costPerItem, string description) { if(quantity < 0 || costPerItem < 0.0 || description == "") return NULL; else { PurchaseOrder p = new PurchaseOrder(quantity,costPerItem,description); return p; } } what's the issue with this function and how to rewrite itarrow_forwardC++ coding project just need some help getting the code thank you!arrow_forwardWrite a C++ program, you will create a class called Books to hold the information about the books in a library. The information required for each book is as follows Book Title Author name Publisher Publish year Subject Book ID (identical 5 digits’ number) Implement the following getBookInfo () member function that receives a pointer to a book and fills it up with user data. User input validation is necessary. The user should be properly prompted for each field. void getBookInfo (Books *b) Implement the following printBookInfo () member function. void printBookInfo (Books *b) Your function should print the book in the following format: Book Title : Object Oriented Programming Using C++ Author : Chris M. Szalwinski Publisher : Amazon Year : 2016 Subject : Computer Science Book Id : 00034 Note: You need to add required constructors/destructors, member functions or data members/variables in your program to complete its execution. //Missing lines of codes to be completed by the student }…arrow_forward
- Double Bubble For this exercise you need to create a Bubble class and construct two instances of the Bubble object. You will then take the two Bubble objects and combine them to create a new, larger combined Bubble object. This will be done using functions that take in these Bubble objects as parameters. The Bubble class contains one data member, radius_, and the corresponding accessor and mutator methods for radius_, GetRadius and SetRadius. Create a member function called CalculateVolume that computes for the volume of a bubble (sphere). Use the value 3.1415 for PI. Your main function has some skeleton code that asks the user for the radius of two bubbles. You will use this to create the two Bubble objects. You will create a CombineBubbles function that receives two references (two Bubble objects) and returns a Bubble object. Combining bubbles simply means creating a new Bubble object whose radius is the sum of the two Bubble objects' radii. Take note that the CombineBubbles function…arrow_forwardIn C++ Please: THE PROGRAM CANNOT CONTAIN -> OPERATORS! Create a class AccessPoint with the following: x - a double representing the x coordinate y - a double representing the y coordinate range - an integer representing the coverage radius status - On or Off Add constructors. The default constructor should create an access point object at position (0.0, 0.0), coverage radius 0, and Off. Add accessor and mutator functions: getX, getY, getRange, getStatus, setX, setY, setRange and setStatus. Add a set function that sets the location coordinates and the range. Add the following member functions: move and coverageArea. Add a function overLap that checks if two access points overlap their coverage and returns true if they do. Add a function signalStrength that returns the wireless signal strength as a percentage. The signal strength decreases as one moves away from the access point location. Represent this with bars like, IIIII. Each bar can represent 20% Test your class by writing a…arrow_forwardll data members must be declared as private. Global variables are not allowed. Constants are ok. There should be no usage of cin or cout in the method or in the class definition or required functions. They should be handled using parameters and return values. cin and cout can and should be used in the testing functions. There should be only one “return” statement in a function or method. Multiple return statements within the same function/method are not allowed. Please pay attention to the required data types in the description. tuple, list and vector classes are not allowed to be used in this exam. Please write C++ functions, class and methods to answer the following questions. 1. Define a new C++ class named “Exam” that manages simple exam information: student id (integer) and a score (integer) The class must provide at least the following two methods: • isPassing which will return a boolean: true if the score is >=60 and false otherwise • toString method that returns the string…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
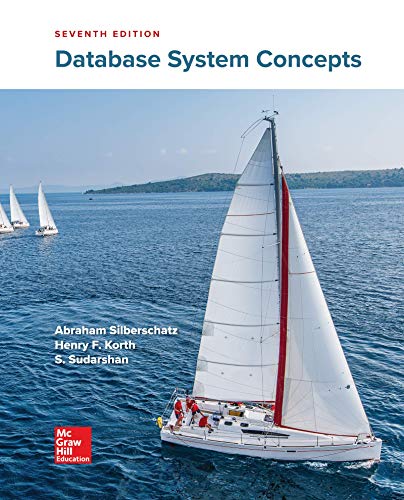
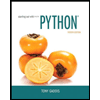
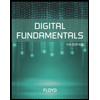
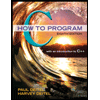
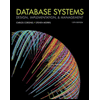
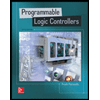