Background: This assignment will help you further learn to use multidimensional arrays. You will need a 10x2 matrix for this problem. Consider 10 points on a map, You need to identify the distance between each point (or vertex). A vertex requires two pieces of information. An x,y coordinate (think longitude and latitude.) This is why you have a matrix of 10x2, each entry is the two values you will need. You can think of these points a cities, or friend's houses. We're only looking for a direct route (as the crow flies) not taking roads to make it simpler. You can find the distance between any two points by use of: sqr_root((x1 - x2)^2 + (y1 - y2)^2) You will need to fill your matrix with some random values. Then you will need to calculate the distance between each possible point, eventually displaying it on a matrix in output. Think something like: 1 2 3 4 5 1 0 8 12 4 6 2 8 0 7 .... 3 12 7 .... 4 4 ... 5 6 ... Hint: You may want to display the point values somewhere as well. Hint: There should be a section where each value is 0, as the distance from a point to itself if zero (0)
Background: This assignment will help you further learn to use multidimensional arrays.
You will need a 10x2 matrix for this problem.
Consider 10 points on a map, You need to identify the distance between each point (or vertex). A vertex requires two pieces of information. An x,y coordinate (think longitude and latitude.) This is why you have a matrix of 10x2, each entry is the two values you will need.
You can think of these points a cities, or friend's houses. We're only looking for a direct route (as the crow flies) not taking roads to make it simpler.
You can find the distance between any two points by use of: sqr_root((x1 - x2)^2 + (y1 - y2)^2)
You will need to fill your matrix with some random values.
Then you will need to calculate the distance between each possible point, eventually displaying it on a matrix in output.
Think something like:
1 2 3 4 5
1 0 8 12 4 6
2 8 0 7 ....
3 12 7 ....
4 4 ...
5 6 ...
Hint: You may want to display the point values somewhere as well.
Hint: There should be a section where each value is 0, as the distance from a point to itself if zero (0)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

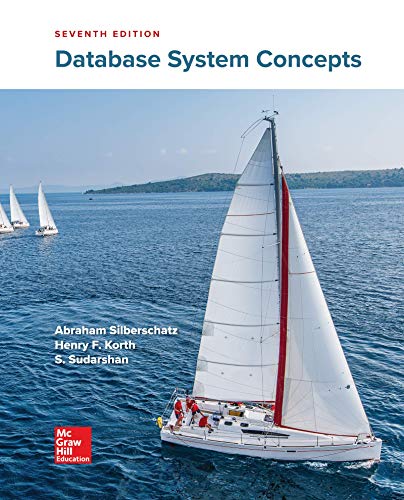
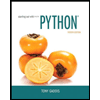
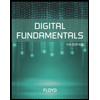
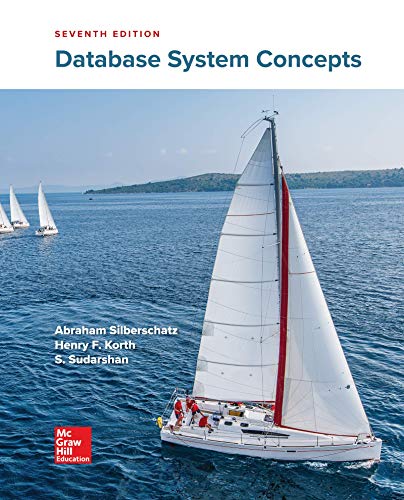
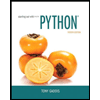
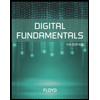
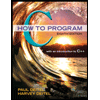
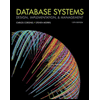
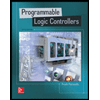