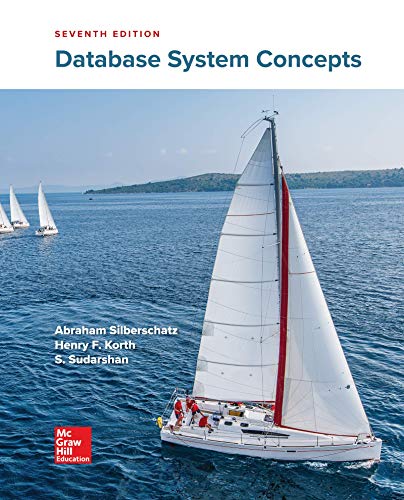
Concept explainers
Project 1b: Create a Flights Database using ArrayList of Objects
For this Lab assignment, you will create an ArrayList of Java Objects, populate them and print out the results.
Specifically, you will create an ArrayList of type Flight (ArrayList<Flight>). A Flight contains an attribute of type AircraftType, which is modeled above. An AircraftType contains an engineType attribute which is an enumeration type called EngineType.
Steps to create this project:
- You should study the modules relating to ArrayLists, ArrayList of Objects SimpleDateFormat, and Java Enums.
- Create a new project in IntelliJ called for example FlightArrayList and a class FlightArrayList. Follow the pattern in the ArrayLists of Objects module page.
- Create the classes Flight and AircraftType and the EngineType enumeration as modeled above. For simplicity, these can be inner classes (Links to an external site.) of your public main class FlightArrayList.
- Flight and AircraftType should have public constructors to allow you to easily populate objects of these types.
- Flight and AircraftType should have toString() (Links to an external site.) methods that display key Flight and AircraftType and EngineType attributes.
- Create a main method that declares a variable of type ArrayList<Flight>.
- To make your life easier, I suggest you create another ArrayList of type ArrrayList<AircraftType> and populate it with various aircraft types since a Flight must have an AircraftType. If you have an ArrayList of type ArrrayList<AircraftType> pre-populated, you can use the ArrayList get method to refer to an AircraftType, e.g. acType.get(3) where acType is an ArrayList, referring to the fourth element in the AircraftType array list. Set the engineType attributes using one of the EngineType enum values, e.g. EngineType.Jet or EngineType.TurboProp. Set up various aircraft types. Here a few real examples, you can use to populate your AircraftType ArrayList.
AircraftType Examples:
Manufacturer | Model | Type Designator | Engine Type | Number Engines |
Boeing | 737-600 | B736 | Jet | 2 |
Boeing | 737-800 | B738 | Jet | 2 |
Airbus | A-310 | A310 | Jet | 2 |
Airbus | A-300B2 | A30B | Jet | 2 |
Airbus | A-340-500 | A-340-500 | Jet | 2 |
Embraer | A-20 | E314 | TurboProp | 1 |
Embraer | 195 | E195 | Jet | 2 |
8. You will also need to set the scheduledArrival and scheduledDeparture times of flights. These schedules are of type Java Date. An easy way to do this to declare a SimpleDateFormat named for example, sdf, then use the sdf to parse a date string into a Java Date.
SimpleDateFormat sdf = new SimpleDateFormat("MM-dd-yyyy HH:mm");// Now you can convert a dateString to a Java date like this:Date date = sdf.parse("12-10-2020 09:35")
9. With aircraftTypes and dates, you can now create Flights using the Flight constructor. Create five or more flights, varying data, times, aircraft types, destinations, origins, gates, and so on. Gates should start with a letter, followed by a number. Origin and destination should be three-letter airport codes, e.g. PHL, JFK, CDG, LAX, SFO, ORD, MIA, DFW, etc.
10. Finally print out your results: (requires you to define toString() methods in your Flight and AircraftType classes )
for (Flight flt: fltArrayList) {System.out.println(flt);
}
Sample Output: AA 101 PHL/ORD Departs: 12-10-2020 07:35 Arrives: 12-10-2020 09:35 Aircraft: Boeing-737-600 EngType/Num: Jet/2 Gate: A27
UA 101 PHL/SFO Departs: 12-10-2020 08:35 Arrives: 12-10-2020 11:50 Aircraft: Airbus-A-310 EngType/Num: Jet/2 Gate: G15
DL 233 ATL/CDG Departs: 12-10-2020 16:35 Arrives: 12-11-2020 07:50 Aircraft: Airbus-A-310 EngType/Num: Jet/2 Gate: B15
WN 101 PHL/LAX Departs: 12-10-2020 08:35 Arrives: 12-10-2020 11:50 Aircraft: Airbus-A-340-500 EngType/Num: Jet/2 Gate: G15
AA 221 MCO/FLL Departs: 12-10-2020 05:35 Arrives: 12-10-2020 07:33 Aircraft: Airbus-A-310 EngType/Num: Jet/2 Gate: C11
UA 199 PHL/JFK Departs: 12-10-2020 08:35 Arrives: 12-10-2020 11:50 Aircraft: Embraer-A-20 EngType/Num: TurboProp/1 Gate: D15

Required a code: To provide a java code that can produce the below output.
AA 101 PHL/ORD Departs: 12-10-2020 07:35 Arrives: 12-10-2020 09:35 Aircraft: Boeing-737-600 EngType/Num: Jet/2 Gate: A27
UA 101 PHL/SFO Departs: 12-10-2020 08:35 Arrives: 12-10-2020 11:50 Aircraft: Airbus-A-310 EngType/Num: Jet/2 Gate: G15
DL 233 ATL/CDG Departs: 12-10-2020 16:35 Arrives: 12-11-2020 07:50 Aircraft: Airbus-A-310 EngType/Num: Jet/2 Gate: B15
WN 101 PHL/LAX Departs: 12-10-2020 08:35 Arrives: 12-10-2020 11:50 Aircraft: Airbus-A-340-500 EngType/Num: Jet/2 Gate: G15
AA 221 MCO/FLL Departs: 12-10-2020 05:35 Arrives: 12-10-2020 07:33 Aircraft: Airbus-A-310 EngType/Num: Jet/2 Gate: C11
UA 199 PHL/JFK Departs: 12-10-2020 08:35 Arrives: 12-10-2020 11:50 Aircraft: Embraer-A-20 EngType/Num: TurboProp/1 Gate: D15
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Input values seat1, travel_time1 and plane_num1 are read from input, representing the seat, travel_time, and plane_num of a passenger. An element representing a new seat is also read from input as new_seat. Complete the following tasks: Assign passenger1 with an instance of Passenger with seat1, travel_time1 and plane_num1 as attributes in that order. Call passenger1's print_data(). Call passenger1's move_seat() with argument new_seat. Call passenger1's print_data() again.arrow_forwardSay we are about to build an ArrayList. Your ArrayList should guarantee that the array capacity is at most four times the number of elements. What would you like to do to maintain such a limit on the capacity? What is the benefit of using iterators? Can you describe your first experience of GUIs? And could you describe what is the advantage of using GUIs over Command-Line Interface (CLI) operations?arrow_forwardCreate an Empty Activity Project to implement the following Layouts. Layouts: one Text, one E-mail, one Image and one Button. Tips: - The project’s layout structure is ConstraintLayout. - The first element is Text. You must write your name in place of “XYZ” with a textSize = 20sp and textColor of Red. - The second element is E-mail. You must write your email address under your name, with the textColor = Blue. - The third element is an image. You can add any image after your name and email with any size you like. - The fourth element is a button. Place the button on the end of the page and call it ”OK”. Implement the previous requirements and include the XML file and screenshot of the layout’s output, after you run the application. Layout’s output:arrow_forward
- Create a TeeShirt class for Lebo’s Tee Shirt Company. Attributes include an order number, size, color, and price. Create set methods for the order number, size, and color and get methods for all four attributes. The price is determined by the size: R122.99 for XXL or XXXL, and R109.99 for all other sizes. Create a subclass named CustomTee that descends from TeeShirt and includes an attribute to hold the slogan requested for the shirt, and include get and set methods this field. The display() method should me an abstract method. Write a test class named DemoTees.java that creates two objects of each class, and demonstrate that all the methods work correctly by communicating to the user to input the tee shirt size, color, and slogan, then display the tee shirt order number, size, color, slogan and price. Format your output to 2 decimal places. The user should be asked to repeat the process or not, (y) indicates yes they want to repeat the process an the process repeats and (n)…arrow_forwardplease help me with this JAVA programming 1 assignment. You are creating a database of fey creatures found in Western Arkansas. Your data includes the creature's species, description, diet, and difficulty to catch. Include the following in your database: Species Description Diet Danger Level Times Caught Sprite Small winged humanoid berries medium 1 Goblin Common evil fey Omnivorous medium 5 Troll Large, dangerous, ugly humanoids high 1 Boggart Mischievous teleporting goblin-like creature stolen pies low 4 Gnome Small, shy, burrowing burrowing critters low 2 Dryad tree-maiden spirit tied to a forest unknown medium 0 Using one or more arrays, display this list. Modify the program so the user enters a number. Then, display all the creatures (and their info) who have been caught at least that many times. In addition to the above, allow the user to enter the name of a creature. If that creature is in the list, add one to the number of times that creature has been…arrow_forwardfast in pyth pleasearrow_forward
- As the first step in writing an application for the local book store, we will design and implement a Book class that will define the data structure of the application. Each book has a name, author, type (paperback, hardback, magazine), integer ID and pageCount. Provide some data validation in the appropriate method to ensure that the ID and pageCount are not negative. Design and implement a Book class with separation, i.e., separate all functions and methods into both the prototype and an implementation below the main. Provide constructor methods for Book(void), Book(name, author), and one for all components Book(name, author, type, ID, pageCount) Provide accessor (get and set) methods for each property as well as a display method. Provide data validation for the ID and pageCount in the appropriate methods. Provide a method to calculate the cost for buying books (with a 7.75% sales tax) according to the following chart: Hardcover: $29.95 per book Paperback:…arrow_forwardCreate a new project named lab7_1. You will be implementing a Tacos class. For the class attributes, let’s use to type of taco, number of tacos, and price. Note that the price per taco of any type is 99 cents. Therefore, allow the taco type and number of tacos to be set directly, but not the price! The price should be set automatically based on the number of tacos entered. So a good idea may be to call the setter for the price inside the function that sets the number of tacos. Some other requirements: A default constructor and a constructor with parameters for your taco type and number of tacos member variables. You should also include setters and getters for these private member variables. However, make sure that the setter for price is private! You don’t want anyone outside the class modifying price. This setter should be called anytime you change the number of tacos. Your constructor needs to call a set method that allows you to set the two member variables, thus takes two…arrow_forwardQUESTION 3 Which of the following is true about Introduce parameter object refactoring technique?1. Does not work with Java2. The technique is used to increase performance as it requires less memory3. You will need to create a new structure (class) for the grouped data if that structure does not already exist 4.Used to to shorten methodsarrow_forward
- Q2: Create a book modelDraw a class diagram to depict a book as specified by the following statement: "A book is made up of several parts, each of which is made up of several chapters."Sections are made up of chapters." To begin, concentrate just on classes and associations.Multiplicity should be added to the class diagram you created.Improve the class diagram by adding the following features:• The book has a publisher, a publishing date, and an ISBN number.• A title and a number are included in a part; a title, a number, and an abstract are included in a chapter; and a title, a number, and an abstract are included in a section.Take a look at the refined class diagram. A title and a number characteristic are included in the Part, Chapter, and Section classes. To factor out these two traits, use inheritance.arrow_forwardDesign a class named CustomerRecord that holds a customer number, name, and address. Include separate methods to 'set' the value for each data field using the parameter being passed. Include separate methods to 'get' the value for each data field, i.e. "return" the field's value. Pseudocode:arrow_forwardAdd 2 constructors to the Course class. One that takes no arguments and initializes the data to all 0’s and “” (empty strings). And one constructor that takes all 4 arguments, one argument for each property and then sets the properties to these arguments that are passed in. Lastly change the main to use these new Constructors. You will not need to call the set functions any more, but do not remove the set functions from your class. Main Code à Course c1; c1 = new Course(323, “Intro to Php”, “Intro to Php Programming”, 4); c1.display(); Problem #2: Do the same as above for the Account class. Problem #3: Do the same as above for the Person class. Below is my Course code public class Course { int Courzeld; String CourteName; String Description; int creditHours; void display() { System.out.println("Course ID: " + Courzeld); System.out.println("Course Name: " + CourteName); System.out.println("Description: " + Description);…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
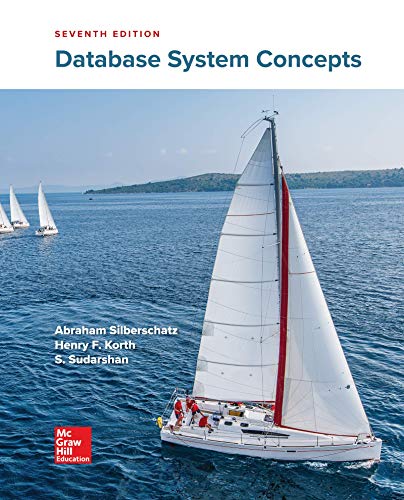
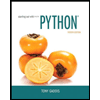
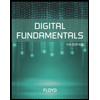
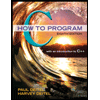
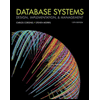
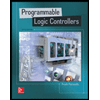