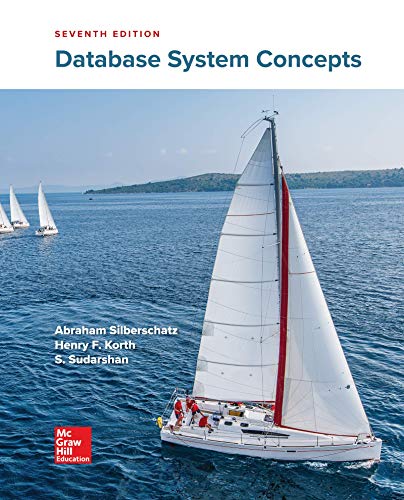
Concept explainers
Assign negativeCntr with the number of negative values in the linked list.
Thanks.
// ===== Code from file IntNode.java =====
public class IntNode {
private int dataVal;
private IntNode nextNodePtr;
public IntNode(int dataInit, IntNode nextLoc) {
this.dataVal = dataInit;
this.nextNodePtr = nextLoc;
}
public IntNode(int dataInit) {
this.dataVal = dataInit;
this.nextNodePtr = null;
}
/* Insert node after this node.
* Before: this -- next
* After: this -- node -- next
*/
public void insertAfter(IntNode nodePtr) {
IntNode tmpNext;
tmpNext = this.nextNodePtr; // Remember next
this.nextNodePtr = nodePtr; // this -- node -- ?
nodePtr.nextNodePtr = tmpNext; // this -- node -- next
}
// Grab location pointed by nextNodePtr
public IntNode getNext() {
return this.nextNodePtr;
}
public int getDataVal() {
return this.dataVal;
}
}
// ===== end =====
// ===== Code from file CustomLinkedList.java =====
import java.util.Random;
public class CustomLinkedList {
public static void main(String[] args) {
Random randGen = new Random();
IntNode headObj; // Create IntNode reference variables
IntNode currObj;
IntNode lastObj;
int i; // Loop index
int negativeCntr;
negativeCntr = 0;
headObj = new IntNode(-1); // Front of nodes list
lastObj = headObj;
for (i = 0; i < 10; ++i) { // Append 10 rand nums
int rand = randGen.nextInt(21) - 10;
currObj = new IntNode(rand);
lastObj.insertAfter(currObj); // Append curr
lastObj = currObj; // Curr is the new last item
}
currObj = headObj; // Print the list
while (currObj != null) {
System.out.print(currObj.getDataVal() + ", ");
currObj = currObj.getNext();
}
System.out.println("");
currObj = headObj; // Count number of negative numbers
while (currObj != null) {
/* Your solution goes here */
currObj = currObj.getNext();
}
System.out.println("Number of negatives: " + negativeCntr);
}
}
// ===== end =====

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- please fix code to match "enter patients name in lbs" thank you import java.util.LinkedList;import java.util.Queue;import java.util.Scanner; interface Patient { public String displayBMICategory(double bmi); public String displayInsuranceCategory(double bmi);} public class BMI implements Patient { public static void main(String[] args) { /* * local variable for saving the patient information */ double weight = 0; String birthDate = "", name = ""; int height = 0; Scanner scan = new Scanner(System.in); String cont = ""; Queue<String> patients = new LinkedList<>(); // do while loop for keep running program till user not entered q do { System.out.print("Press Y for continue (Press q for exit!) "); cont = scan.nextLine(); if (cont.equalsIgnoreCase("q")) { System.out.println("Thank you for using BMI calculator"); break;…arrow_forwardIn Java please help with the following: Sees whether this list is empty.@return True if the list is empty, or false if not. */ public boolean isEmpty(); } // end ListInterface Hint:Node class definition should look something like: public class Node<T> { T element; Node next; Node prev; public Node(T element, Node next, Node prev) { this.element = element;this.next = next;this.prev = prev; } }arrow_forwardimport java.util.Scanner; public class Playlist { // TODO: Write method to ouptut list of songs public static void main (String[] args) { Scanner scnr = new Scanner(System.in); SongNode headNode; SongNode currNode; SongNode lastNode; String songTitle; int songLength; String songArtist; // Front of nodes list headNode = new SongNode(); lastNode = headNode; // Read user input until -1 entered songTitle = scnr.nextLine(); while (!songTitle.equals("-1")) { songLength = scnr.nextInt(); scnr.nextLine(); songArtist = scnr.nextLine(); currNode = new SongNode(songTitle, songLength, songArtist); lastNode.insertAfter(currNode); lastNode = currNode; songTitle = scnr.nextLine(); } // Print linked list…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
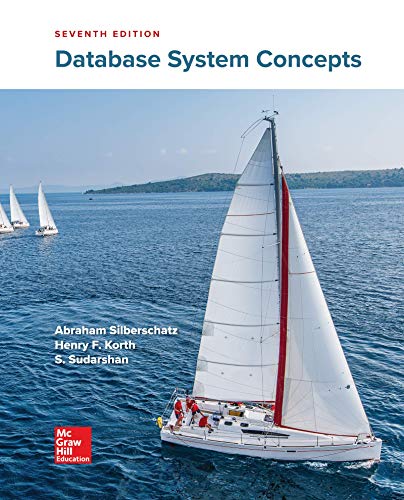
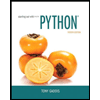
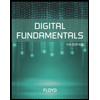
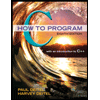
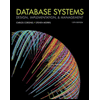
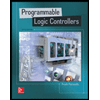