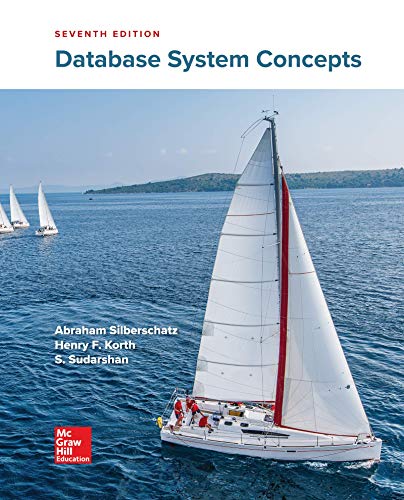
Assign courseStudent's name with Smith, age with 20, and ID with 9999. Use the printAll() member method and a separate println() statement to output courseStudents's data. Sample output from the given program:
Name: Smith, Age: 20, ID: 9999
// ===== Code from file PersonData.java =====
public class PersonData {
private int ageYears;
private String lastName;
public void setName(String userName) {
lastName = userName;
}
public void setAge(int numYears) {
ageYears = numYears;
}
// Other parts omitted
public void printAll() {
System.out.print("Name: " + lastName);
System.out.print(", Age: " + ageYears);
}
}
// ===== end =====
// ===== Code from file StudentData.java =====
public class StudentData extends PersonData {
private int idNum;
public void setID(int studentId) {
idNum = studentId;
}
public int getID() {
return idNum;
}
}
// ===== end =====
// ===== Code from file StudentDerivationFromPerson.java =====
public class StudentDerivationFromPerson {
public static void main(String[] args) {
StudentData courseStudent = new StudentData();
/* Your solution goes here */
}
}
// ===== end =====

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Write a function/method called “addStock” that takes: An array of Stocks The number of stocks in the array A string representing a tickerName A string representing the company the tickerName represents A double representing the price of the stock A double representing the quantity of stock owned. The function must create and add the stock to the array if there is space. The function must return “true” if the addition was successful and “false” otherwise.arrow_forward6. Design a Java class with a main method that does the following. You must include a meaningful comment for main and each method a. Main invokes a method readScores to read input from a file (name of your choice) that contains the name of the bowlers (no more than 25) and their bowling scores (two scores per bowler). The program stops reading values when it has reached the end of the file. For example, the file might contain: Bob 203 176 Jane 210 253 Jack 300 189 Eileen 220 298 • As the method reads the data, it stores the name into a String array and the average of the two bowling scores into an array of double. • The method returns the actual number of bowlers read in b. Main then invokes a method computeOverallAvg to: compute (a) the overall average score of all the bowlers combined, (b) the average score and name of the bowler with the highest average score and (c) the average score and name of the bowler with the lowest average scores. print to the console (a) the overall average…arrow_forwardprivate Vector location;private Vector velocity;private Vector acceleration;private int lifespan;private static final int MAX_LIFESPAN = 25;private static final int RADIUS = 7; public boolean isAlive() { } public void update() {//code here} Using java, if particle is alive then add velocity vector to location vector, add acceleration vector to velocity vector, and decrement lifespan by 1. Thank you.arrow_forward
- Design a Student class so that the following output is produced upon executing the following code: [Hint: Each course has 3.0 credit hours. You must take at least 9.0 and at most 12.0 credit hours] Driver Code Output # Write your code here Name: Alice # Do not change the following lines of code. ID: 20103012 s1 = Student("Alice","20103012","CSE") s2 = Student("Bob", “18301254","EEE") s3 = Student("Carol", “17101238","CSE") Department: CSE %3D #### Name: Bob ID: 18301254 print("##### ###") Department: EEE print(s1.details()) Alice, you have taken 9.0 credits. List of courses: CSE110, MAT110, PHY111 print("####### print(s2.details()) Status: Ok print("######### ##### Bob, you have taken 6.0 credits. s1.advise("CSE110", “MAT110", “PHY111") List of courses: BUS101, MAT120 print("############### ##") Status: You have to take at least 1 more course. #### ##### = Carol, you have taken 15.0 credits. s2.advise("BUS101", “MAT120") print("############ H## List of courses: MAT110, PHY111, ENG102,…arrow_forwardJAVA Program Homework #1. Chapter 7. PC# 2. Payroll Class (page 488-489) Write a Payroll class that uses the following arrays as fields: * employeeId. An array of seven integers to hold employee identification numbers. The array should be initialized with the following numbers: 5658845 4520125 7895122 8777541 8451277 1302850 7580489 * hours. An array of seven integers to hold the number of hours worked by each employee * payRate. An array of seven doubles to hold each employee’s hourly pay rate * wages. An array of seven doubles to hold each employee’s gross wages The class should relate the data in each array through the subscripts. For example, the number in element 0 of the hours array should be the number of hours worked by the employee whose identification number is stored in element 0 of the employeeId array. That same employee’s pay rate should be stored in element 0 of the payRate array. The class should have a method that accepts an employee’s identification…arrow_forwardIn python and include doctring: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie that takes a Movie…arrow_forward
- !! E! 4 2 You are in process of writing a class definition for the class Book. It has three data attributes: book title, book author, and book publisher. The data attributes should be private. In Python, write an initializer method that will be part of your class definition. The attributes will be initialized with parameters that are passed to the method from the main program. Note: You do not need to write the entire class definition, only the initializer method lili lilıarrow_forwardIn C# Use a one-dimensional array to solve the following problem. Write a class called ScoreFinder. This class receives a single dimensional integer array representing passer ratings for NFL players as an argument for its constructor. The test class will provides this argument. The test class then calls upon a method from the ScoreFinder class to start the process of finding a specific rating, which will be provided by the user. The method will iterate through the array and find any matching values. For all the matches that are found, the method will note the index of the cell along with its value. At completion, the method will print a list of all the indices and the associated scores along with a count of all the matching values.arrow_forwardJAVA PROGRAM Homework #1. Chapter 7. PC# 2. Payroll Class (page 488-489) Write a Payroll class that uses the following arrays as fields: * employeeId. An array of seven integers to hold employee identification numbers. The array should be initialized with the following numbers: 5658845 4520125 7895122 8777541 8451277 1302850 7580489 * hours. An array of seven integers to hold the number of hours worked by each employee * payRate. An array of seven doubles to hold each employee’s hourly pay rate * wages. An array of seven doubles to hold each employee’s gross wages The class should relate the data in each array through the subscripts. For example, the number in element 0 of the hours array should be the number of hours worked by the employee whose identification number is stored in element 0 of the employeeId array. That same employee’s pay rate should be stored in element 0 of the payRate array. The class should have a method that accepts an employee’s identification…arrow_forward
- Using python write a program that contains 1. a class named information that has the following hidden data attributes ( write appropriate accessor and mutator methods): name address age phone 2. using main() function create an instance of information that holds your information 3. following is the output after this program executes : my information: Name: Your name( first and last): address: 1111 my street. age: 24. phone Number: 555-555-1234 write code without commentsarrow_forward/*** This class describes a tweet. A tweet has a message in it, a unique ID,* a count of the number of likes, and a count of the number of times it* has been retweeted. In addition, the Tweet class will have a static * variable to count the total number of tweets to ever be created * (retweets don't count as a new tweet).* * You may NOT import any library class.* * **/public class Tweet { /*** This constructor creates a tweet with the given message.* Assume that the message is not null because only the tweet() method* from the TwitterUser class will call this constructor. There are* no length requirements on Tweets.* * The very first tweet to ever be created will have an ID of 0, the * next one will have 1, and so on and so forth. It may help you to use* the static count variable to set the ID. * * You will have to initialize other instance variables appropriately.* @param message the text of the tweet*/public Tweet(String message) {throw new UnsupportedOperationException("Implement…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
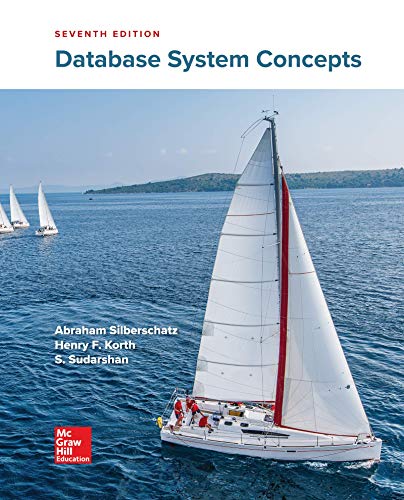
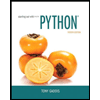
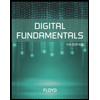
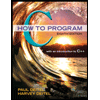
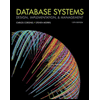
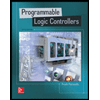