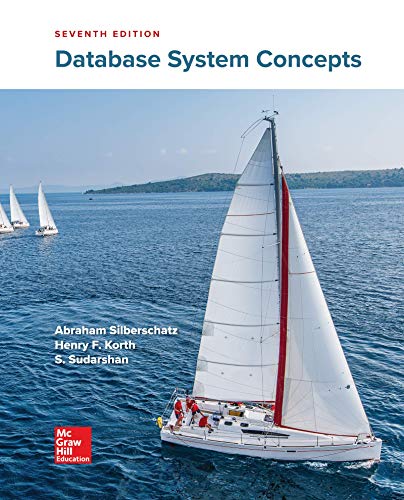
Concept explainers
Nearest smaller element
def nearest_smaller(items):
Given a list of integer items, create and return a new list of the same length but where each element has been replaced with the nearest element in the original list whose value is smaller. If no smaller elements exist because that element is the minimum of the original list, the element in the result list should remain as that same minimum element.
If there exist smaller elements equidistant in both directions, you must resolve this by using the smaller of these two elements. This again makes the expected results unique for every possible value of items, which is necessary for the automated testing framework to work at all. Being permissive in what you accept while being restrictive in what you emit is a pretty good principle to follow in all walks of life, not just in programming.
items | Expected result |
[42, 42, 42] | [42, 42, 42] |
[42, 1, 17] | [1, 1, 1] |
[42, 17, 1] | [17, 1, 1] |
[6, 9, 3, 2] | [3, 3, 2, 2] |
[5, 2, 10, 1, 13, 15, 14, 5, 11, 19, 22] | [2, 1, 1, 1, 1, 13, 5, 1, 5, 11, 19] |
[1, 3, 5, 7, 9, 11, 10, 8, 6, 4, 2] | [1, 1, 3, 5, 7, 9, 8, 6, 4, 2, 1] |

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- SingleLinkedList head- Node next - data- String value = "Tom" Node next- data- String value = "Dick" Node nodeRef = nodeRef.next; nodeRef.next = new Node("Peter"); next- data- String value="Harry" What change will be made to the list after the following codes are run? Node nodeRef = head; While (nodeRef.next!=null) Node next = null data- String value="Sam"arrow_forwardIn pyton: In this lab, you will be building a software application that removes duplicate values from a list.The purpose of the assignment isto gain experience with user-defined functions, forloop and listmanipulation. Start Start of remove duplicate function (numbers: list of numbers) Initialize an empty list as new_list Loop over each of the number of the numbers list If number not in new_list Append number to new_list Return new_list End of remove duplicate functionStart main function Prompt user for list of numbers Call remove duplicate function Print the new list returned by the remove function End of main functionEndarrow_forwardA list called parts has already been created and filled with data. It has a length of 5. Write a for loop that prints each value in this list.arrow_forward
- • find_last(my_list, x): Takes two inputs: the first being a list and the second being any type. Returns the index of the last element of the list which is equal to the second input; if it cannot be found, returns None instead. >> find_last(['a', 'b', 'b', 'a'], 'b') 2 >>> ind = find_last(['a', 'b', 'b', 'a'], 'c') >>> print(ind) Nonearrow_forwardSA1: What is the maximum number of values linear search must check to find a value in a list with 2 billion values? SA2: What is the maximum number of values binary search must check to find a value in a list with 2 billion values?arrow_forwardstruct nodeType { int infoData; nodeType * next; }; nodeType *first; … and containing the values(see image) Using a loop to reach the end of the list, write a code segment that deletes all the nodes in the list. Ensure the code performs all memory ‘cleanup’ functions.arrow_forward
- struct remove_from_front_of_dll { // Function takes no parameters, removes the book at the front of a doubly // linked list, and returns nothing. void operator()(const Book& unused) { //// TO-DO (13) |||| // Write the lines of code to remove the book at the front of "my_dll", // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. ///// END-TO-DO (13) //// } std::list& my_dll; };arrow_forwardO b. Remove the O. Remove the second element in the list O d. Remove the element before the last element in the list QUESTION 6 The following code inserts an element at the beginning of the list public void insertBegin(T e) { Node temp = new Node (e); head = temp; temp.Next = head; size++; } O True O False QUESTION 7 what does the following code do?arrow_forwardC Programming Language Task: Deviation Write a program that prompts the user to enter N numbers and calculates which of the numbers has the largest deviation from the average of all numbers. You program should first prompt the user to enter how many numbers that will specify. The program should then scan for each number, separated by a newline. You should calculate the average value and return the number from the list which is furthest away from this average (to 2dp). Try using dynamic memory functions to store the incoming array of numbers on the heap. Code to build from: + 1 #include 2 #include 3 4 int main(void) { 5 6} 7 Output Example: deviation.c How many numbers? 5 Enter them: 1.0 2.0 6.0 3.0 4.0 Average: 3.20 Largest deviation from average: 6.00arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
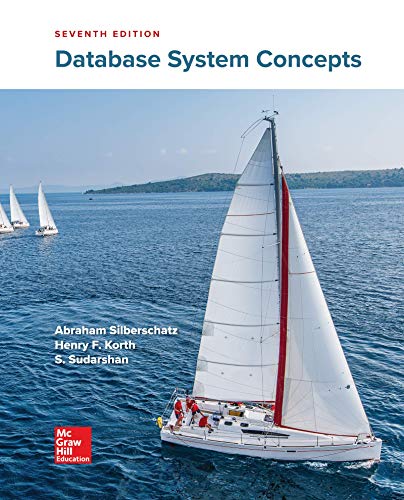
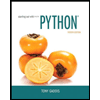
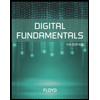
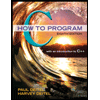
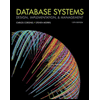
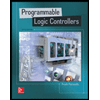