(app.py) file: # Store this code in 'app.py' file from flask import Flask, render_template, request, redirect, url_for, session from flask_mysqldb import MySQL import MySQLdb.cursors import re app = Flask(__name__) if __name__ == "__main__": app.run(debug=True) app.secret_key = 'your secret key' app.config['MYSQL_HOST'] = 'localhost' app.config['MYSQL_USER'] = 'root' app.config['MYSQL_PASSWORD'] = '' app.config['MYSQL_DB'] = '' mysql = MySQL(app) @app.route('/') @app.route('/login', methods =['GET', 'POST']) def login(): msg = '' if request.method == 'POST' and 'username' in request.form and 'password' in request.form: username = request.form['username'] password = request.form['password'] cursor = mysql.connection.cursor(MySQLdb.cursors.DictCursor) cursor.execute('SELECT * FROM accounts WHERE username = % s AND password = % s', (username, password, )) account = cursor.fetchone() if account: msg = 'Logged in successfully !' return render_template('index.html', msg = msg) else: msg = 'Incorrect username / password !' return render_template('login.html', msg = msg)
Task question:
Step 1. You have been asked to add code in given template file “app.py” to enable login using employee table in test
Create Data Base: test
Create table: employee
Table fields:
Id |
Int |
10(primary key) auto increment |
username |
Varchar |
25 |
Password |
Varchar |
25 |
EmpMail |
Varchar |
25 |
With 2 records
1 |
Manager |
Manager123 |
manager@gmail.com |
2 |
Teller |
Teller123 |
teller@gmail.com |
Login should have username and password field. You are asked to add SQL code to get successful login.
Step 2. you should also add error message if credentials are wrong-Validation step
Step 3: Add code to login .html template file to create Login form using text and password fields.
Step 4. Add button to get sign-in action
Step 5: you should save the file in relevant locations to avoid path and output issues.
Step 6. You should have proper database connectivity to succeed in this lab worksheet. Successful connection to database also will be counted for marking.
Show your outputs through screenshots.
(app.py) file:
# Store this code in 'app.py' file
from flask import Flask, render_template, request, redirect, url_for, session
from flask_mysqldb import MySQL
import MySQLdb.cursors
import re
app = Flask(__name__)
if __name__ == "__main__":
app.run(debug=True)
app.secret_key = 'your secret key'
app.config['MYSQL_HOST'] = 'localhost'
app.config['MYSQL_USER'] = 'root'
app.config['MYSQL_PASSWORD'] = ''
app.config['MYSQL_DB'] = ''
mysql = MySQL(app)
@app.route('/')
@app.route('/login', methods =['GET', 'POST'])
def login():
msg = ''
if request.method == 'POST' and 'username' in request.form and 'password' in request.form:
username = request.form['username']
password = request.form['password']
cursor = mysql.connection.cursor(MySQLdb.cursors.DictCursor)
cursor.execute('SELECT * FROM accounts WHERE username = % s AND password = % s', (username, password, ))
account = cursor.fetchone()
if account:
msg = 'Logged in successfully !'
return render_template('index.html', msg = msg)
else:
msg = 'Incorrect username / password !'
return render_template('login.html', msg = msg)


Step by step
Solved in 3 steps with 3 images

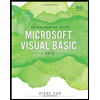
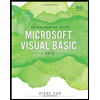