Q: Step 1. You have been asked to add code in given template file “app.py” to enable login using employee table in test database. First Create Data Base: test Create table: employee Table fields: Id Int 10(primary key) auto increment username Varchar 25 Password Varchar 25 EmpMail Varchar 25 With 2 records 1 Manager Manager123 manager@gmail.com 2 Teller Teller123 teller@gmail.com Login should have username and password field. You are asked to add SQL code to get successful login. Step 2. you should also add error message if credentials are wrong-Validation step Step 3: Add code to login .html template file to create Login form using text and password fields. Step 4. Add button to get sign-in action Step 5: you should save the file in relevant locations to avoid path and output issues. Step 6. You should have proper database connectivity to succeed in this lab worksheet. Successful connection to database also will be counted for marking. Show your outputs through screenshots. (app.py) file: # Store this code in 'app.py' file from flask import Flask, render_template, request, redirect, url_for, session from flask_mysqldb import MySQL import MySQLdb.cursors import re app = Flask(__name__) if __name__ == "__main__": app.run(debug=True) app.secret_key = 'your secret key' app.config['MYSQL_HOST'] = 'localhost' app.config['MYSQL_USER'] = 'root' app.config['MYSQL_PASSWORD'] = '' app.config['MYSQL_DB'] = '' mysql = MySQL(app) @app.route('/') @app.route('/login', methods =['GET', 'POST']) def login(): msg = '' if request.method == 'POST' and 'username' in request.form and 'password' in request.form: username = request.form['username'] password = request.form['password'] cursor = mysql.connection.cursor(MySQLdb.cursors.DictCursor) cursor.execute('SELECT * FROM accounts WHERE username = % s AND password = % s', (username, password, )) account = cursor.fetchone() if account: msg = 'Logged in successfully !' return render_template('index.html', msg = msg) else: msg = 'Incorrect username / password !' return render_template('login.html', msg = msg) photo dowen if u need them see
Q:
Step 1. You have been asked to add code in given template file “app.py” to enable login using employee table in test
Create Data Base: test
Create table: employee
Table fields:
Id |
Int |
10(primary key) auto increment |
username |
Varchar |
25 |
Password |
Varchar |
25 |
EmpMail |
Varchar |
25 |
With 2 records
1 |
Manager |
Manager123 |
manager@gmail.com |
2 |
Teller |
Teller123 |
teller@gmail.com |
Login should have username and password field. You are asked to add SQL code to get successful login.
Step 2. you should also add error message if credentials are wrong-Validation step
Step 3: Add code to login .html template file to create Login form using text and password fields.
Step 4. Add button to get sign-in action
Step 5: you should save the file in relevant locations to avoid path and output issues.
Step 6. You should have proper database connectivity to succeed in this lab worksheet. Successful connection to database also will be counted for marking.
Show your outputs through screenshots.
(app.py) file:
# Store this code in 'app.py' file
from flask import Flask, render_template, request, redirect, url_for, session
from flask_mysqldb import MySQL
import MySQLdb.cursors
import re
app = Flask(__name__)
if __name__ == "__main__":
app.run(debug=True)
app.secret_key = 'your secret key'
app.config['MYSQL_HOST'] = 'localhost'
app.config['MYSQL_USER'] = 'root'
app.config['MYSQL_PASSWORD'] = ''
app.config['MYSQL_DB'] = ''
mysql = MySQL(app)
@app.route('/')
@app.route('/login', methods =['GET', 'POST'])
def login():
msg = ''
if request.method == 'POST' and 'username' in request.form and 'password' in request.form:
username = request.form['username']
password = request.form['password']
cursor = mysql.connection.cursor(MySQLdb.cursors.DictCursor)
cursor.execute('SELECT * FROM accounts WHERE username = % s AND password = % s', (username, password, ))
account = cursor.fetchone()
if account:
msg = 'Logged in successfully !'
return render_template('index.html', msg = msg)
else:
msg = 'Incorrect username / password !'
return render_template('login.html', msg = msg)
photo dowen if u need them see

![mo/AppData/Local/Temp/Rar$EXa12340.29280/login.html
Login
{{ msg }}
Enter Your Username
Enter Your Password
mo/AppData/Local/Temp/Rar$EXa12340.27329/index.html
Sign In
Don't have an account? Sign Up here
Index
Hi
Welcome to the index page...
Logout
* Below line is used for online Google font */
@import
h2{
background-color: #FEFFED;
padding: 30px 35px;
margin: -10px -50px;
text-align:center;
border-radius: 10px 10px 0 0;
}
hr{
url(http://fonts.googlepis.com/css?family=Raleway);
margin: 10px -50px;
border: 0;
border-top: 1px solid
margin-bottom: 40px;
}
div.container {
width: 900px;
height: 610px;
margin:35px auto;
font-family: Raleway', sans-serif;
}
div.main{
width: 300px;
padding: 10px 50px 25px;
border: 2px solid gray;
border-radius: 10px;
font-family: raleway;
float:left;
margin-top: 50px;
}
input[type=text],input[type=password] {
width: 100%;
height: 40px;
padding: 5px;
margin-bottom: 25px;
margin-top: 5px;
border: 2px solid #ccc;
color: #4f4f4f;
#ccc;
S
label{
color: #464646;
text-shadow: 0 1px #fff;
font-size: 14px;
font-weight: bold;
}
center{
font-size: 32px;
}
.note{
color: red;
}
.valid{
color: green;
}
.back{
text-decoration: none;
border: 1px solid rgb(0, 143, 255);
rgb(0, 214, 255);
background-color:
padding: 3px 20px;
border-radius: 2px;
color: black;
}
input[type=button]{
font-size: 16px;
background: linear-gradient(#ffbc00 5%,#ffdd7f 100 %);
border: 1px solid #e5a900;
color: #4E4D4B;
font-weight: bold;
cursor: pointer;
width: 100%;
border-radius: 5px;
padding: 10px 0;
outline: none;
}
input[type=button]:hover{
background: linear-gradient( #ffdd7f 5%, #ffbc00 100%);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4e5f358a-93dd-4ca8-9db7-e8099ebb1ddc%2Ff38a3e3a-e634-4ca1-9428-0aa8b10ddcd4%2F10w84bi_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

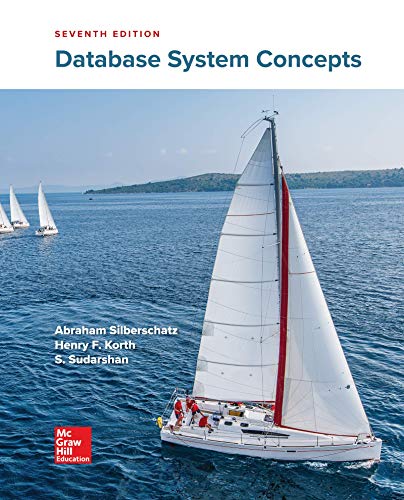
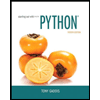
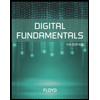
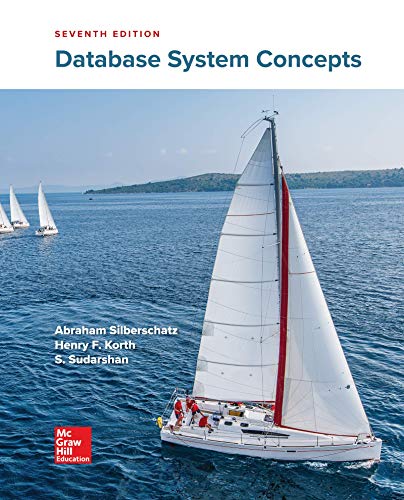
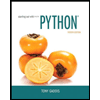
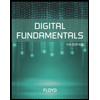
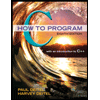
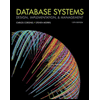
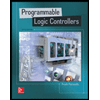