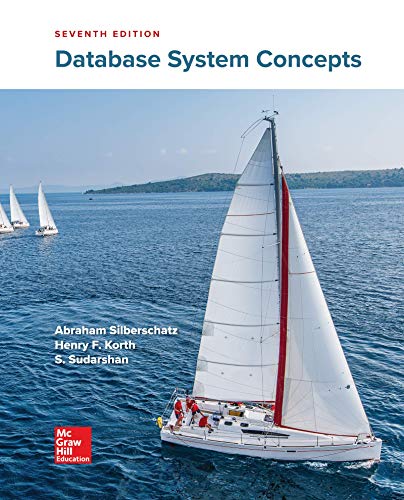
Any help with this first problem would be really helpful! Thank you so much!!
1. Write the following exercises using Java code. Add comments explaining what your code is doing:
Prompt the user to enter any full name (first middle surname). Do not bother with surnames like O'Reilly, Van Helsing, de Ville etc. Then output the following:
- the length of the full name.
- the length of the middle name.
- the three initials of the name.
- the name in all upper case.
Example Output
Enter a first name middle name and surname
Peggy Sue Palmer
Length of your name: 16 characters
Length of your middle name: 3 characters
Your initials are PSP
PEGGY SUE PALMER
-Write a program that generates two random integers, both in the range 50 to 100, inclusive. Use the Math class. Print both integers and then display the positive difference between the two integers, but use a selection. Do not use the absolute value method of the Math class.
-Duke Shirts sells Java t-shirts for $24.95 each, but discounts are possible for quantities as follows:
1 or 2 shirts, no discount and total shipping is $10.00
3-5 shirts, discount is 10% and total shipping is $8.00
6-10 shirts, discount is 20% and total shipping is $5.00
11 or more shirts, discount is 30% and shipping is free
Write a Java program that prompts the user for the number of shirts required. The program should then print the extended price of the shirts, the shipping charges, and the total cost of the order. Use currency format where appropriate.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Any help with this first problem would be really helpful! Thank you so much!! 1. Write the following exercises using Java code. Add comments in each program to explain what your code is doing. A. Write a program that generates two random integers, both in the range 50 to 100, inclusive. Use the Math class. Print both integers and then display the positive difference between the two integers, but use a selection. Do not use the absolute value method of the Math class. B. Duke Shirts sells Java t-shirts for $24.95 each, but discounts are possible for quantities as follows: 1 or 2 shirts, no discount and total shipping is $10.003-5 shirts, discount is 10% and total shipping is $8.006-10 shirts, discount is 20% and total shipping is $5.0011 or more shirts, discount is 30% and shipping is free Write a Java program that prompts the user for the number of shirts required. The program should then print the extended price of the shirts, the shipping charges, and the total cost of the…arrow_forwardin Java Tasks Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows. 1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don't display the computer's choice yet.) 2. The user enters his or her choice of "rock", "paper", or "scissors" at the keyboard. (You can use a menu if you prefer.) 3. The computer's choice is displayed. Tasks 4. A winner is selected according to the following rules: a. If one player chooses rock and the other player chooses scissors, then rock wins. (The rock smashes the scissors.) b. If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.) C. If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps…arrow_forwardPROBLEM STATEMENT: Return the String "odd" if the input is odd otherwisereturn "even". Can you help me with this Java Question.arrow_forward
- Instructor note: This lab is part of the assignment for this chapter. HINT: 1). The decision means how many times to flip the coin. 2). Use a random integer variable to indicate the head or tail in each toss. Java Define a method named coinFlip that takes a Random object and returns "Heads" or "Tails" according to a random value 1 or 0. Assume the value 1 represents "Heads" and 0 represents "Tails". Then, write a main program that reads the desired number of coin flips as an input, calls method coinFlip() repeatedly according to the number of coin flips, and outputs the results. Assume the input is a value greater than 0. Ex: If the random object is created with a seed value of 2 and the input is: 3 the output is: Heads Tails Heads Note: For testing purposes, a Random object is created in the main() method using a pseudo-random number generator with a fixed seed value. The program uses a seed value of 2 during development, but when submitted, a different seed value may be used…arrow_forward2. The initial value of the radius of a circle is equal to one unit and each succeeding radius is one unit greater than the value before it. Build a program to compute the area of the circle starting with R=1.0 up to R=5.0, then print out each radius and the corresponding area of the circle. USED JAVA PROGRAMMINGarrow_forwardDo not send AI generated response to me. I need correct answer without any plagiarism otherwise I'll reduce rating for sure.arrow_forward
- Help me find the problem with this java questionarrow_forward1. Write a program that draws various figures, as shown in Figure (a). The user selects a figure from a radio button and uses a check box to specify whether it is filled. Using Java programmingarrow_forwardPlease help me with this question below using javaarrow_forward
- Graphics / Fonts on digits, characters etc. 1. Generate graphics of digits 0, 1, 2, 3, .., until 8, and 9. Write Java program that generates the 10 digits 0, 1, 2, 3, .., until 9 (a) Display it like stick fonts as displayed in LED such as follows: 0123 Note your design does not have to be similar to mine like above, but just make it look like sticks that probably use drawLine method in class Graphics (b) Display it using pixels like dot matrix printer or bitmap image such as follows (you may use some fillRect method in class Graphics) 0123 (c) Display Graphic or image fonts for English letters (5 is enough). Make fonts (either stick fonts like Q(a) or image "thick” font like Q(b)) for 5 (five) English letters of your choice, it can be upper case or lower case, like say ABCDE or abcde. It does not have to be ABCDE, it can be AEIOU; or if you like you can make fonts of Foreign languages like those in Spanish, German etc.arrow_forwardInstructions from your teacher: Write a program that asks the user to enter a character. Then pass the character to the following methods. changeCase() – if the character is lower case then change it to upper case and if the character is in upper case then change it to lower case. Example output is given below: Enter a character a a is equivalent to A Note: Java represents character using Unicode encoding and ‘A’ to ‘Z’ is represented by numbers 65 to 90 and ‘a’ to ‘z’ is represented by numbers 97 to 122. So to convert ‘A’ to ‘a’ you need to add (97-65) to A and then cast it to Character type since the addition will change its type to integer. You can use the following code to read a character from console. When you call charAt(i) method for any String it returns the character at index i. The index of first character is 0, the index of second character is 1 and so on. Scanner input = new Scanner(System.in); char ch =input.next().charAt(0); If you…arrow_forwardCreate a java program that enters 5 adjectives. Count and print all the adjective that ends with the suffix 'ful'. (Use endsWith() method in the slides Lecture on Methods.pdf) Example run: Enter an adjective: happy Enter an adjective: joyful Enter an adjective: pretty Enter an adjective: peaceful Enter an adjective: prayerful Word Count: 3arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
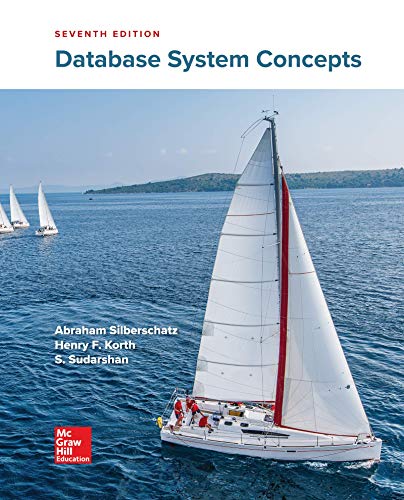
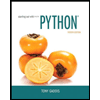
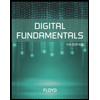
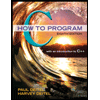
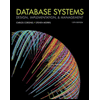
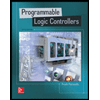