Annotate following code. def QualityPointCalculator(qualPnts, crdts): if crdts > 0: return qualPnts/crdts return 0 def convertGrade(grade): if grade == "A": return 4.00 elif grade == "A-": return 3.70 elif grade == "B+": return 3.30 elif grade == "B": return 3.00 elif grade == "B-": return 2.70 elif grade == "C+": return 2.30 elif grade == "C": return 2.00 elif grade == "C-": return 1.70 elif grade == "D+": return 1.30 elif grade == "D": return 1.00 else: return 0.00 def calcQualPnts(pnts, crdts): return pnts*crdts if __name__ == '__main__': quality_points = [] course_names = [] grades = [] credits = [] final_gpa = 0.0 semester_gpa = 0.0 final_total = 0.0 howMany = 0 print('GRADE & QUALITY NUMERICAL POINTS') choice = input('Would you like to calculate your GPA for a term? Enter Y/N: ') while choice.upper()[0] == 'Y': howMany = int(input('How many courses did you take in the term: ')) for i in range(howMany): course_name = input('Enter the name of course '+str(i+1)+': ') course_names.append(course_name) credit = float(input('Enter the number of credits associated with that course: ')) credits.append(credit) grade = input('Enter the grade for the course: ') grade = grade.upper() grades.append(grade) quality_point = calcQualPnts(convertGrade(grade),credit) quality_points.append(quality_point) semester_gpa += quality_point print('\nThis term you have earned:') print('%-15s%-10s%-10s%-15s' %('Course','Credits','Grade','Quality Points')) for i in range(howMany): print('%-15s%-10d%-10s%-15.1f' %(course_names[i],credits[i],grades[i],quality_points[i])) print('Total earned credits this term: %d' %(sum(credits))) gpa = QualityPointCalculator(sum(quality_points),sum(credits)) print('You earned %.1f quality points and a GPA of %.2f for the term.' %(sum(quality_points),gpa)) final_gpa += semester_gpa final_total += sum(credits) choice = input("\nWould you like to calculate another term's GPA? Enter Y/N: ") quality_points = [] course_names = [] grades = [] credits = [] semester_gpa = 0 print('\nYour overall earned credits: %d '%final_total) gpa = QualityPointCalculator(final_gpa,final_total) print('Your overall GPA: %.2f' %(gpa))
Annotate following code.
def QualityPointCalculator(qualPnts, crdts):
if crdts > 0:
return qualPnts/crdts
return 0
def convertGrade(grade):
if grade == "A":
return 4.00
elif grade == "A-":
return 3.70
elif grade == "B+":
return 3.30
elif grade == "B":
return 3.00
elif grade == "B-":
return 2.70
elif grade == "C+":
return 2.30
elif grade == "C":
return 2.00
elif grade == "C-":
return 1.70
elif grade == "D+":
return 1.30
elif grade == "D":
return 1.00
else:
return 0.00
def calcQualPnts(pnts, crdts):
return pnts*crdts
if __name__ == '__main__':
quality_points = []
course_names = []
grades = []
credits = []
final_gpa = 0.0
semester_gpa = 0.0
final_total = 0.0
howMany = 0
print('GRADE & QUALITY NUMERICAL POINTS')
choice = input('Would you like to calculate your GPA for a term? Enter Y/N: ')
while choice.upper()[0] == 'Y':
howMany = int(input('How many courses did you take in the term: '))
for i in range(howMany):
course_name = input('Enter the name of course '+str(i+1)+': ')
course_names.append(course_name)
credit = float(input('Enter the number of credits associated with that course: '))
credits.append(credit)
grade = input('Enter the grade for the course: ')
grade = grade.upper()
grades.append(grade)
quality_point = calcQualPnts(convertGrade(grade),credit)
quality_points.append(quality_point)
semester_gpa += quality_point
print('\nThis term you have earned:')
print('%-15s%-10s%-10s%-15s' %('Course','Credits','Grade','Quality Points'))
for i in range(howMany):
print('%-15s%-10d%-10s%-15.1f' %(course_names[i],credits[i],grades[i],quality_points[i]))
print('Total earned credits this term: %d' %(sum(credits)))
gpa = QualityPointCalculator(sum(quality_points),sum(credits))
print('You earned %.1f quality points and a GPA of %.2f for the term.' %(sum(quality_points),gpa))
final_gpa += semester_gpa
final_total += sum(credits)
choice = input("\nWould you like to calculate another term's GPA? Enter Y/N: ")
quality_points = []
course_names = []
grades = []
credits = []
semester_gpa = 0
print('\nYour overall earned credits: %d '%final_total)
gpa = QualityPointCalculator(final_gpa,final_total)
print('Your overall GPA: %.2f' %(gpa))

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

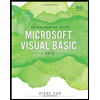
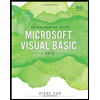