an you help me attain this output? class Voter { static int nVoters=0; String name; String voterId; // Creating the constructor Voter(String name) { this.name=name; nVoters=nVoters+1; voterId="Hi"+nVoters+""+name.length(); } public static int getNVoters()
Can you help me attain this output?
class Voter {
static int nVoters=0;
String name;
String voterId;
// Creating the constructor
Voter(String name)
{
this.name=name;
nVoters=nVoters+1;
voterId="Hi"+nVoters+""+name.length();
}
public static int getNVoters()
{
return nVoters;
}
@Override
public String toString() {
return voterId+" "+name;
}
public static void main(String args[]) {
Scanner input=new Scanner(System.in);
System.out.print("Voter name: " );
String name1=input.nextLine();
Voter voter1=new Voter(name1);
System.out.println("Voter "+Voter.getNVoters()+" created.");
System.out.print("Voter name: " );
String name2=input.nextLine();
Voter voter2=new Voter(name2);
System.out.println("Voter "+Voter.getNVoters()+" created.");
System.out.print("Voter name: " );
String name3=input.nextLine();
Voter voter3=new Voter(name3);
System.out.println("Voter "+Voter.getNVoters()+" created.");
System.out.println();
System.out.println(voter1);
System.out.println(voter2);
System.out.println(voter3);
}
}


Answer the above program are as follows
Step by step
Solved in 4 steps with 2 images

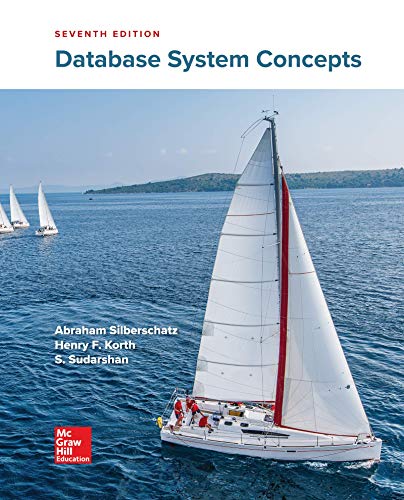
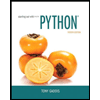
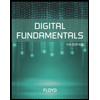
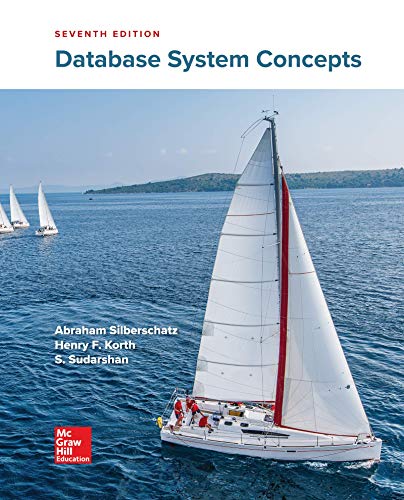
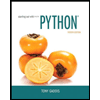
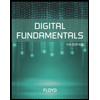
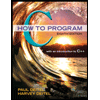
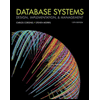
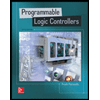