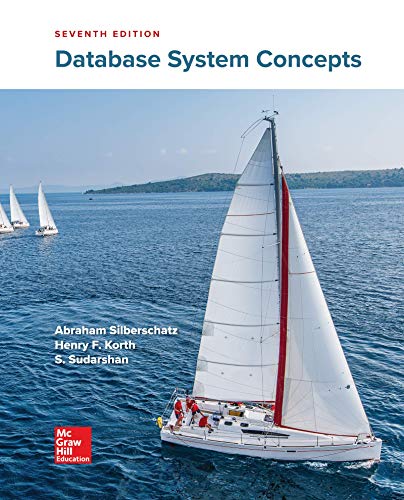
Concept explainers
Having trouble when I run this python
Write a class named Employee that has **private** data members for an employee's name, ID_number, salary, and email_address. It should have an init method that takes four values and uses them to initialize the data members. It should have get methods named get_name, get_ID_number, get_salary, and get_email_address.
Write a separate function (not part of the Employee class) named **make_employee_dict** that takes as parameters a list of names, a list of ID numbers, a list of salaries and a list of email addresses (which are all the same length). The function should take the first value of each list and use them to create an Employee object. It should do the same with the second value of each list, etc. to the end of the lists. As it creates these objects, it should add them to a dictionary, where the key is the ID number and the value for that key is the whole Employee object. The function should return the resulting dictionary.
For example, it could be used like this:
```
emp_names = ["Jean", "Kat", "Pomona"]
emp_ids = ["100", "101", "102"]
emp_sals = [30, 35, 28]
emp_emails = ["Jean@aol.com", "Kat@aol.com", "Pomona@aol.com"]
result = make_employee_dict(emp_names, emp_ids, emp_sals, emp_emails)
print(result["100"].get_name())
```
Remember in your testing that printing an object of a user-defined class will just print out the object's type and address in memory. If you want to print the values of its data members, you need to call its get functions, as shown in the print statement above.
CODE IS BELOW
class Employee:
def __init__(self, names, id_number, salary, email_address):
self.names = names
self.id_number = id_number
self.salary = salary
self.email_address = email_address
def get_names(self):
return self.names
def get_ID_number(self):
return self.id_number
def get_salary(self):
return self.salary
def get_email_address(self):
return self.email_address
def make_employee_dict(emp_names, emp_id, emp_salary, emp_email):
emp = {}
emp_len = len(emp_id)
for x in range(emp_len):
names = emp_names[z]
ID_number = emp_id[z]
salary = emp_salary[z]
email = emp_email[z]
emp[id_number] = emp(names, id_number, salary, email)
return emp

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

I am still confused about how to remedy this situation. Can you show me the section of the code that needs to be fixed? and further, explain?
I'm getting errors when I run this. Its saying "Traceback (most recent call last):
File "<string>", line 47, in <module>
File "<string>", line 30, in make_employee_dict
KeyError: ('Jean', '100', 30, 'Jean@aol.com')
> " How can this be remedied?
I am still confused about how to remedy this situation. Can you show me the section of the code that needs to be fixed? and further, explain?
I'm getting errors when I run this. Its saying "Traceback (most recent call last):
File "<string>", line 47, in <module>
File "<string>", line 30, in make_employee_dict
KeyError: ('Jean', '100', 30, 'Jean@aol.com')
> " How can this be remedied?
- Python Code: Write a class called Person with a constructor that takes two arguments, name and age and the saves these as attributes Write a method in this called print_person that prints out this information the console Confirm that you did it properly by instantiating an object called p1 and calling the method on the object. Lastly, extend the constructor by adding functionality that checks the input name only contains letters (no numbers allowed).arrow_forwardProgramming Demo 3: Dog Class Write the program below to familiarize with the conventional syntax and formatting of Object-Oriented Programming in Python. 1. Let us create a class Dog with the script below. Save this script as dogs.py. Take note of the indentions, colons, and number of parenthesis used to avoid errors on our program. 12345678 3 9 10 11 12 class Dog: definit_(self, name, breed, age): self.name = name self.breed breed = self.age = age def sit(self): print (self.name.title() + is now sitting.") 123456 def roll(self): " print (self.name.title() + rolled over!") def bark(self, times): print (self.name, 'says woof', times, times.') 2. Identify the user-defined attributes/parameters on our class Dog. " 3. Identify the user-defined methods or actions that can be acted out on our class Dog. 4. Create a new Python script and name it as sub_dogs.py. Make sure this script is saved on the same folder with dogs.py. Write the codes below then run it. from dogs import Dog dog1=…arrow_forwardI'm learning java in college and I missed one homework assignment. Since then it has been difficult to keep up with homework because they were based on the one I missed. Here's the prompt: Design a class Phone with one data member as long phoneNumber. Add constructors, get/set methods to class Phone. Then design a class InternationalPhone as subclass of class Phone, with one extra data member as int countryCode. Add constructors, get/set methods to class InternationalPhone. Write a main program to test these two classes. (We use netbeans btw) I can't get any credit for this so there's no rush but I would be very happy to recieve an answer before the end of the semester. Thank you in advance :)arrow_forward
- Written in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardIn the following code: def foo(value): value = value + 1 def main(): x = 10 foo(x) Ox is a formal parameter and value is an actual parameter. O Both value and x are actual parameters. value is a formal parameter and x is an actual parameter. O There are no parameters.arrow_forwardI've gotten stuck on this practice problem. I need help figuring this out. You need to make a Pet class that holds the following information as private data members: the name of the pet (e.g. "Spot", "Fluffy", or any word a user enters) the type of pet (e.g. dog, cat, snake, hamster, or any word a user enters) level of hungriness of the pet (2 means hungry, 1 means content, 0 means full) Your class also needs to have the following functions: a default constructor that sets the pet to be a dog named Buddy, with level of hungriness being “content” a parameterized constructor to allow having any type of pet the order of the parameters is: name of the pet, type of pet, hungriness level a PrintInfo function that prints the info for the pet according to the sample output below a TimePasses function that increases the hungry level of the pet by one (unless its hungry level is already 2, meaning hungry). a FeedPet function that changes the hungry level of the pet to 0 (Full) You must…arrow_forward
- Please include doctring, and write only in python: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie…arrow_forwardWritten in Python with docstring please if applicable Thank youarrow_forwardFirst question: Why am having an error pop up when running my program that says: "Editor does not contain main type" on my TestVehicle program? Second question: Does the program follow what my professor wants in his instructions? I have gone over them but would like another opinion. Instructions: Design and save your Motor class before you make Vehicle, so you can specify Motor as a Vehicle data attribute. Class Motor (make this class first because Motor is an attribute of class Vehicle)Attributes (all private) int cylinders int hp String type (possible values being gas, deisel, electric etc) Methods (all public) a constructor that can assign values to all attributes getters and for each attribute (setters not needed) a toString method that returns the status of a Motor instance, all attributes. Class VehicleAttributes (all private) String make String model int year double price Motor motor (see below) Methods (public) a constructor that can assign values to all attributes a…arrow_forward
- Help, I making a elevator simulator, I need help in writing code in the Elevator class and it's polymorphism class. I am not sure what to do next. Any help is appreciated. There are 4 types of passengers in the system:Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements.VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators.Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators.Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. There are 4 types of elevators in the system:StandardElevator: This is the most common type of elevator and has a request…arrow_forwardProgram - Python Make a class.The most important thing is to write a simple class, create TWO different instances, and then be able to do something. For example, create a pizza instance for Suzie, ask her for the toppings from the choices, and store her pizza order. Then create a second pizza instance for Larry, ask for his toppings and store his pizza order. Then print both of their orders back out. You do not need to make your class very complicated. (Shouldn't be the pizza example but something similar)arrow_forwardIn Python For problems A, B, and C you will be writing two different classes to simulate a Boat race. Problem A is to write the first class Boat. A Boat object must hold the following information boat_name: string top_speed: int current_progress: int Write a constructor that allows the programmer to create an object of type Boat with the arguments boat_name and top_speed. The boat_name should be set to the value of the corresponding argument - this argument is required. The top_speed should default to the value 3 if no value is passed in for the argument. The value for current_progress should always be set to 0. Implement Boat class with setter and getter methods: Provide setters for the following instance variables: set_top_speed takes in an int and updates the top_speed set_boat_name takes in a string and updates the boat_name set_current_progress takes in a int and updates the current_progress Provide getters for the following instance variables with no input parameters…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
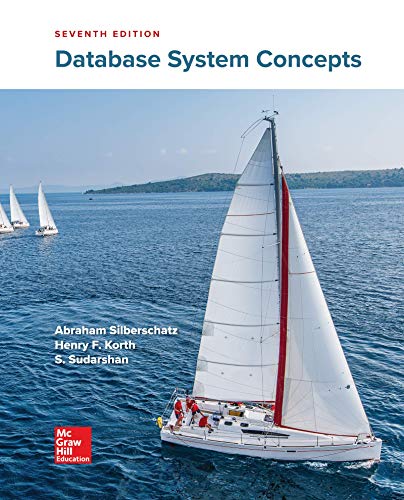
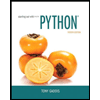
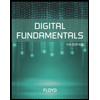
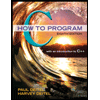
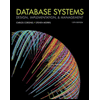
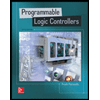