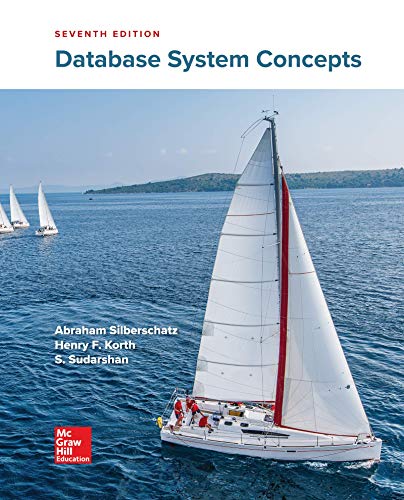
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
//Below is the starting code for this homework assignment//
#include <stdio.h>
struct employees
{
char name[20];
int ssn[9];
int yearBorn, salary;
};
struct employees e = {"kim deen", {1,2,3,4,5,6,7,8,9}, 1998, 35000};
void display(struct employees *e)
{
printf("%s", e->name);
printf(" %d%d%d-%d%d-%d%d%d%d", e->ssn[0],e->ssn[1],e->ssn[2],e->ssn[3],e->ssn[4],e->ssn[5],e->ssn[6],e->ssn[7],e->ssn[8]);
printf(" %d", e->yearBorn);
printf("\n$%d.", e->salary);
}
int main()
{
display(&e);
return 0;
}
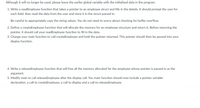
Transcribed Image Text:Although it will no longer be used, please leave the earlier global variable with the initialized data in the program.
1. Write a readEmployee function that takes a pointer to an employee struct and fills in the details. It should prompt the user for
each field, then read the data from the user and store it in the struct passed in.
Be careful to appropriately copy the string values. You do not need to worry about checking for buffer overflow.
2. Define a createEmployee function that will allocate the memory for an employee structure and return it. Before returning the
pointer, it should call your readEmployee function to fill in the data.
3. Change your main function to call createEmployee and hold the pointer returned. This pointer should then be passed into your
display function.
4. Write a releaseEmployee function that will free all the memory allocated for the employee whose pointer is passed in as the
argument.
5. Modify main to call releaseEmployee after the display call. You main function should now include a pointer variable
declaration, a call to createEmployee, a call to display and a call to releaseEmployee.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Consider the following structure and declarations: struct Player { string firstName; string lastName; int hits; int runs; }; int main() { Player p = { "Jackie", "Robinson", 1518, 947 }; Player team[24]; Player *tPtr = team; team[0] = p; // INSERT CODE HERE } Consider adding these 2 code statements at the location commented "//INSERT CODE HERE". What is printed out? cout << tPtr->hits << " "; cout << team[0].runs; a) it is compiler dependent -- some print out 1518 947, others print out 947 1518 b) 947 947 c) 0 0 d) 1518 1518 e) 1518 947arrow_forward#include <iostream> #include <iomanip> #include <string> using namespace std; struct Teletype { string name; string phonenum; Teletype *nextaddr; }; void populate(Teletype *); void displayrecord(Teletype *); //void insertrecord(Teletype *); // create //void removerecord(Teletype *); //create //void modifyrecord(Teletype *); // create //int find(TeleType *, string); // Extra Credit create bool check(); int main() { int location = 0; int count = 0; char answery_n; Teletype *list, *current; list = new Teletype; current = list; cout << "Please "; do { count++; populate(current); if (check() == false) { cout << " Not storage available" << endl; } else { current->nextaddr = new Teletype; current = current->nextaddr;…arrow_forwardstruct gameType { string title; int numPlayers; bool online; }; gameType Awesome[50]; Write the C++ statement that sets the first [0] title of Awesome to Best.arrow_forward
- //Below is the starting code for this homework assignment// #include <stdio.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees e = {"kim deen", {1,2,3,4,5,6,7,8,9}, 1998, 35000}; void display(struct employees *e) { printf("%s", e->name); printf(" %d%d%d-%d%d-%d%d%d%d", e->ssn[0],e->ssn[1],e->ssn[2],e->ssn[3],e->ssn[4],e->ssn[5],e->ssn[6],e->ssn[7],e->ssn[8]); printf(" %d", e->yearBorn); printf("\n$%d.", e->salary); } int main() { display(&e); return 0; }arrow_forwardConsider the following code: tyepdef struct { char grade; float score; } student; void f() { student a = { .grade = 'C', .score = 75.0 }; student b = { .grade = 'C', .score = 75.0 }; if (!memcmp(&a, &b, sizeof(student))) { printf("Same"); } else { printf("Different"); } } What could cause the program to print "Different"? A. memcmp examines the bytes in little endian B. memcmp returns true if contents of the memory are the same; remove the ! C. memcmp always returns a non-zero value D. The compiler may have included padding in the structs E. The compiler may have arranged the fields of the two structs differentlyarrow_forwardIn C++ struct myGrades { string class; char grade; }; Declare myGrades as an array that can hold 5 sets of data and then set a class string in each position as follows: “Math” “Computers” “Science” “English” “History” and give each class a letter gradearrow_forward
- C++ program #include<iostream> #include<fstream> #include<string> using namespace std; const int NAME_SIZE = 20; const int STREET_SIZE = 30; const int CITY_SIZE = 20; const int STATE_CODE_SIZE = 3; struct Customers { long customerNumber; char name[NAME_SIZE]; char streetAddress_1[STREET_SIZE]; char streetAddress_2[STREET_SIZE]; char city[CITY_SIZE]; char state[STATE_CODE_SIZE]; int zipCode; char isDeleted; char newLine; }; void add_data(int no_of_records, ofstream& fout) { struct Customers c; cout << "Enter the Customer data:" << endl; cout << "Name:"; cin >> c.name; cout << "Street Address 1:"; cin >> c.streetAddress_1; cout << "Street Address 2:"; cin >> c.streetAddress_2; cout << "City:"; cin >> c.city; cout << "State:"; cin >> c.state; cout << "Zip Code:"; cin >> c.zipCode; cout << endl; c.customerNumber = no_of_records; c.isDeleted = 'N'; c.newLine = '\n'; cout…arrow_forwardBelow is the starting code for this homework assignment #include <stdio.h> //1. struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; //2. struct employees e = {"kim deen", {1,2,3,4,5,6,7,8,9}, 1998, 35000}; //3. void display(struct employees *e) { printf("%s", e->name); printf(" %d%d%d-%d%d-%d%d%d%d", e->ssn[0],e->ssn[1],e->ssn[2],e->ssn[3],e->ssn[4],e->ssn[5],e->ssn[6],e->ssn[7],e->ssn[8]); printf(" %d", e->yearBorn); printf("\n$%d.", e->salary); } int main() { display(&e); return 0; }arrow_forward#include <iostream> #include <string> using namespace std; int main() { // Declare and initialize variables. string employeeFirstName; string employeeLastName; double employeeSalary; int employeeRating; double employeeBonus; const double BONUS_1 = .25; const double BONUS_2 = .15; const double BONUS_3 = .10; const double NO_BONUS = 0.00; const int RATING_1 = 1; const int RATING_2 = 2; const int RATING_3 = 3; // This is the work done in the housekeeping() function // Get user input cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter employee's yearly salary: "; cin >> employeeSalary; cout << "Enter employee's performance rating: "; cin >> employeeRating; // This is the work done in the detailLoop() function // Use switch statement to…arrow_forward
- #include <bits/stdc++.h> using namespace std; struct Employee { string firstName; string lastName; int numOfHours; float hourlyRate; char major[2]; float amount; Employee* next; }; void printRecord(Employee* e) { cout << left << setw(10) << e->lastName << setw(10) << e->firstName << setw(12) << e->numOfHours << setw(12) << e->hourlyRate << setw(10) << e->amount << setw(9) << e->major[0]<< setw(7) << e->major[1]<<endl; } void appendNode(Employee*& head, Employee* newNode) { if (head == nullptr) { head = newNode; } else { Employee* current = head; while (current->next != nullptr) { current = current->next; } current->next = newNode; } } void displayLinkedList(Employee* head) { Employee* current = head; if(current!=nullptr){ cout…arrow_forwardAn Inventory structure is declared as follows: struct Inventory{int itemCode; int qtyOnHand;}; Write a definition statement that creates an Inventory variable named trivet and initializes it with an initialization list so that its code is 555 and its quantity is110.arrow_forwardstruct gameType { string title; int numPlayers; bool online; }; gameType Awesome[50]; Write the C++ statements that print out all gameType fields from Awesome whose numPlayers is > 1.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
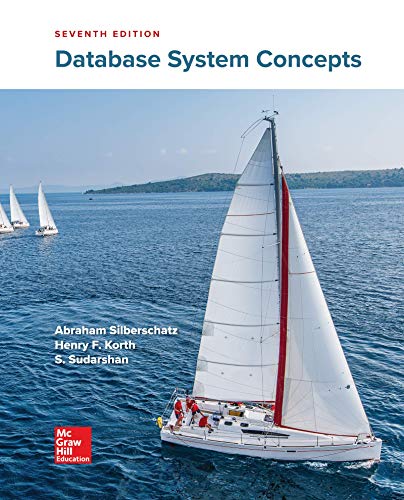
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
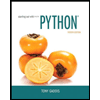
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
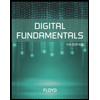
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
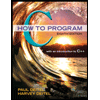
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
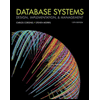
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
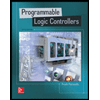
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education